Making real-time file previews with Transloadit's Smart CDN
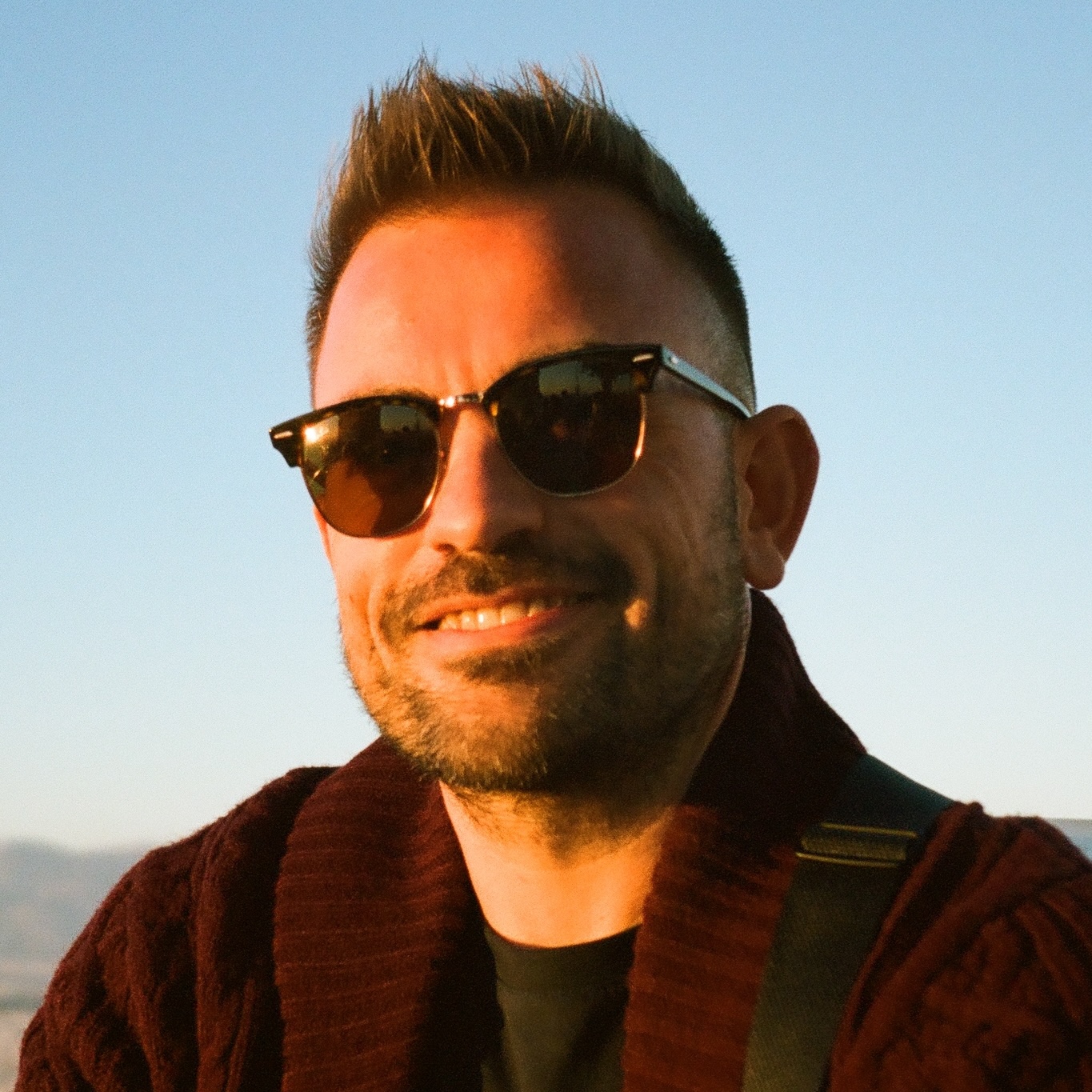
At Transloadit, we strive to make handling media files as efficient and seamless as possible. Today, we are excited to introduce a new feature that takes our offering to the next level: file previews. As the name suggests, this feature allows you to create presentable previews for all kinds of assets by leveraging our robust file processing capabilities.
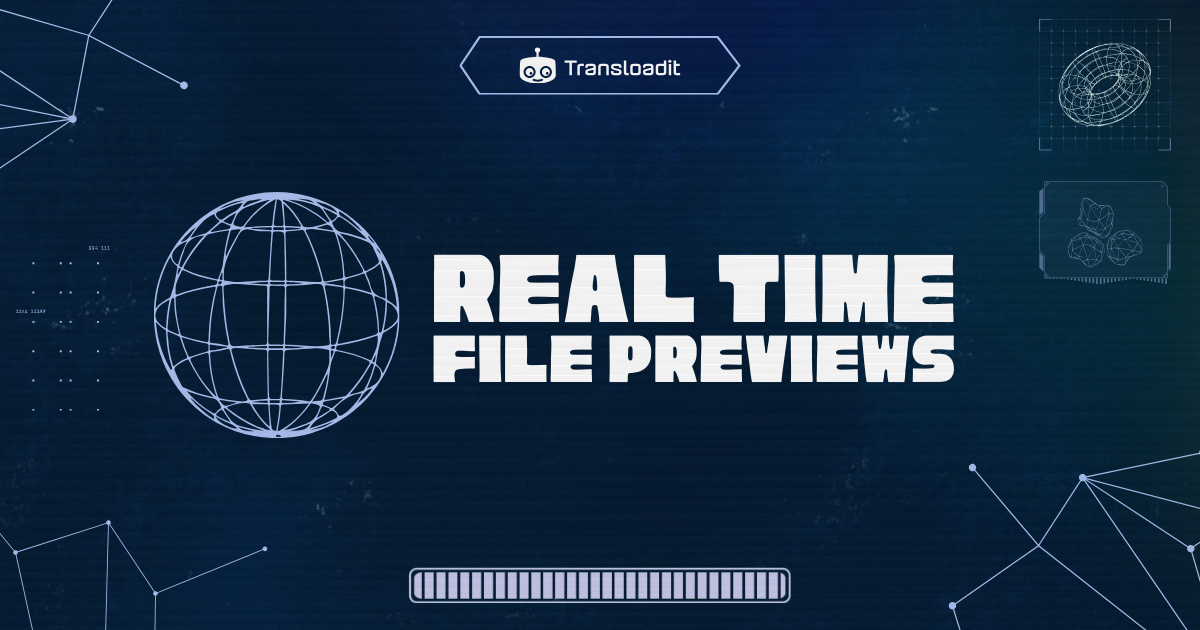
File previews are most effective when combined with our Smart CDN, the Transloadit CDN, ensuring previews are generated and served only upon user request. This approach minimizes preprocessing costs and simplifies integration into your application.
All you really need is:
- a Transloadit account (free or paid to remove watermarks)
- a Transloadit Template (see below for an example)
- the source files in a cloud bucket or on a server that you own
- a URL to our Smart CDN that references the three above
What is the Transloadit Smart CDN?
Content Delivery Networks (CDNs) are a crucial part of modern web applications. They cache your assets in multiple locations around the world, ensuring fast and reliable delivery to your users.
Our Smart CDN takes this concept a step further by integrating seamlessly with our already powerful Assembly engine. This means that we can modify files as end users request them. The first request will take a bit longer, as the file is processed, but any subsequent requests to the same URL will be served from a cache close to your end users, ensuring low latency and high performance, in addition to avoiding unnecessary processing costs.
What is the File Preview feature?
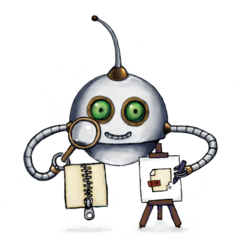
Transloadit offers 72 Robots, which correlate to the same amount of – if not more – features. These can be combined to create powerful media processing workflows.
Our new /file/preview Robot is designed to generate previews for a variety of media types. Whether it's a thumbnail for a video, artwork for an audio file, or a simple icon for a ZIP archive – this Robot can handle it all.
You can add this Robot to your existing preprocessing workflows, use it as a standalone for one-off jobs, or integrate it with our Smart CDN to provide on-the-fly, efficient, and affordable previews.
Key features
- Images: resize, reformat, and crop images on the fly.
- Videos: extract and resize a thumbnail.
- Audio: display artwork if available; otherwise, show a waveform.
- Documents: extract the first page and render it as an image.
- Web pages: take a screenshot of the page.
- Archives: show a compression icon.
To make this feature fast and cost-effective, we only download the first 20 MB and the last 1 MB of the original file. For more than 99.9% of files, this is enough to generate a meaningful preview. For the remaining files, we fall back to an icon for the file type. This approach allows us to quickly process large files without downloading them entirely, saving time and resources.
Benefits of file previews
Implementing file previews in your (web) application can significantly enhance the user experience. We've found that:
- Users can quickly identify the contents of files without opening them, leading to more time spent on your platform and fewer frustrating experiences.
- By generating previews on demand, you avoid the expense of pre-generating previews for all files, when some of those may never be viewed.
- Previews are smaller than original files and can be efficiently cached by our Smart CDN, close to the end user, resulting in faster page loads.
- Previews provide visual context, making it easier for users to navigate through collections of files.
Interactive demo
Naturally, with these kind of claims it's best that you see it to believe it. Use the interactive demo below to see how our /file/preview Robot works. Adjust the settings and watch as the previews and their load times update in real time.
How to use the demo
- Use the sliders to change the dimensions of the preview.
- Choose between JPG, PNG, or GIF formats.
- Select how you want the image to fit within the specified dimensions.
- Watch how your changes affect the preview image, file size, and load time.
How to get started
1. Sign up for Transloadit
Create an account at Transloadit.com.
2. Create a Workspace
Let's say we call it my-app
.
3. Create a Template
To start using the File Preview feature, you'll need to create a Transloadit Template
that includes the /file/preview
Robot. Here's an example Template that
generates the previews as shown in the demo above:
{
"steps": {
"imported": {
"robot": "/http/import",
"url": "https://my-website.com/${fields.input}"
},
"previewed": {
"use": "imported",
"background": "#00000000",
"format": "${fields.f || 'png'}",
"height": "${fields.h}",
"resize_strategy": "${fields.r || 'pad'}",
"robot": "/file/preview",
"width": "${fields.w}"
},
"served": {
"robot": "/file/serve",
"use": "previewed"
}
}
}
We'll call this Template preview
.
4. Use the Smart CDN
To serve the preview, you can use the URL provided by our own Smart CDN:
https://my-app.tlcdn.com/preview?input=audio.mp3&w=240&h=240&f=png&r=pad
As you can see, the URL includes:
- the Smart CDN domain (
tlcdn.com
), - the Workspace (
my-app
), - the Template (
preview
), and - the input file path (
audio.mp3
) (this becomes the value of the${fields.input}
variable in the Template, causing the audio file to be imported fromhttps://my-website.com/audio.mp3
),ß
In addition, it has query parameters that specify:
- the width (
240
) - height (
240
) - format (
png
), and - resize strategy (
pad
) for the preview.
The Workspace, Template, and input variable are a given. The rest of the fields can be
renamed and fully customized how you see fit. Would you rather have &resize_strategy=pad
instead
of &r=pad
? No problem! Simply adjust the Template and the URL accordingly.
It's worth noting that there are two reserved query parameters: sig
and exp
for use with
Signature Authentication. We'll cover this in the next section.
5. Implement security measures
To enhance security and stay in control of who can leverage our encoding platform, it is recommended to implement Signature Authentication. This ensures that only authorized users can generate new variations of your files.
It's important to note that existing files can still be served from the CDN's cache if their location is known.
Here's a simple function to generate signed URLs, along with an example of how to use these in an Express route with authentication:
const crypto = require('crypto')
const express = require('express')
function signUrl(url, secret) {
const expires = Date.now() + 3600000 // URL expires in 1 hour
const urlWithExpiry = `${url}${url.includes('?') ? '&' : '?'}exp=${expires}`
const signature = crypto
.createHmac('sha384', secret)
.update(Buffer.from(urlWithExpiry, 'utf-8'))
.digest('hex')
return `${urlWithExpiry}&sig=sha384:${signature}`
}
const app = express()
// Assume you have some authentication middleware
const authMiddleware = (req, res, next) => {
// Your actual authentication logic here:
req.isAuthenticated = req.headers['x-auth-token'] === 'valid-token'
next()
}
app.get('/get-signed-preview-url', authMiddleware, (req, res) => {
const { url } = req.query
if (!req.isAuthenticated) {
return res.status(401).json({ error: 'User not authenticated' })
}
try {
const signedUrl = signUrl(url, process.env.TRANSLOADIT_SECRET_KEY)
res.json({ signedUrl })
} catch (error) {
res.status(500).json({ error: 'Failed to generate signed URL' })
}
})
app.listen(3000, () => console.log('Server running on port 3000'))
This code:
- Defines a
signUrl
function that takes the original URL and your Secret Key. - Uses the SHA384 algorithm for signature generation.
- Adds an expiration time (1 hour in this example) to the URL. New variations of the file can only be generated within this time frame.
- Generates and appends the signature to the URL.
In the Express route, we use an authMiddleware
to check if the user is authenticated. If the user
is not authenticated, we return a 401 Unauthorized
response. If the user is authenticated, we
generate the signed URL and return it.
To use this in your application:
- Ensure
process.env.TRANSLOADIT_SECRET_KEY
holds your actual Transloadit Secret Key, or replace it with a mechanism for secret retrieval, such as Vault. - Implement your own authentication middleware to properly check user authentication status.
- Call the
/get-signed-preview-url
endpoint with the original URL as a query parameter.
Remember to implement proper authentication mechanisms in your application and keep your Secret Key secure. Never expose your Secret Key in client-side code. Always generate signed URLs on your server and provide them to your client application as needed. This approach allows you to control encoding access based on your own criteria, such as user authentication or time-based expiration.
6. Customize the behavior
You can customize the behavior of the /file/preview Robot by tweaking its parameters. For example, as we did above, you can adjust the width, height, format, and resize strategy for image previews. You can also specify different preview strategies. If you have a collection of documents, for instance, you may want to return icons instead of thumbnails. To learn more about these options, refer to our file preview documentation.
Bring your own storage
Transloadit will happily connect to your existing storage solutions, such as Amazon S3, Google Cloud Storage, or Azure Blob Storage. This way, you can keep your files where they are, and still benefit from our powerful file processing capabilities. You can use our Template Credentials to manage securely accessing and processing these files. To see if your platform is supported, please refer to our file importing documentation. Note that hosting on AWS could potentially reduce latency, and bandwidth costs. For large volumes, this could be a worthwhile consideration.
Bring your own CDN
If you already have a CDN in place, you can still benefit from our file processing capabilities by wrapping our platform with it. We will connect you directly on the origin level, to save one hop, and so that your CDN can cache & serve the processed files. This feature is available to customer who process over 1 TB a month. Please reach out and we'll be happy to help you set this up.
Preprocessing
If you have a large collection of files that you'd like to generate previews for, you can. While this post focusses on on-demand previews served directly to the browser, the power of Transloadit is its LEGO-like composability. You can use the /file/preview Robot in combination with other Robots to create a workflow that processes your files in bulk. This way, you can generate previews for all your files in one bucket, save them in another, use them where you see fit, with full ownership and control. Transloadit would only briefly touch the files.
Coming soon
We are only getting started. Here are a few of the things currently on our roadmap for the /file/preview Robot:
- More file types: RAW images, 3D models, and other file types will be supported soon. For RAW and PSD files, we'll extract embedded thumbnails.
- Improved document previews: Faster previews for documents. Flipping through pages will be
possible. We'll also add the option to show the first characters of
.txt
,.md
,.yaml
, and.json
files. - Video clips: Return clips (Animated GIF/WebP/etc) from videos, not just still thumbnails.
- Serve optimal formats: automatically serve WebP images to browsers that support it, if you set
the
format: 'auto'
parameter.
What other features would you like to see? Contact us and help us prioritize.
Pricing
The /file/preview Robot is available on all our plans, including the free Community Plan. Be aware, however, that this free plan adds a watermark to the previews. To remove the watermark, you can upgrade to a paid plan. For more information on our pricing, please refer to our pricing page and file preview documentation.
Demo Call
If you have big plans and you'd rather have an engineer walk you through this feature, we'd be happy to help.
Conclusion
The new File Preview feature, integrated with our Smart CDN offers a powerful, flexible, and cost-effective way to generate media previews. By providing instant visual context for your files, you can significantly enhance user experience and drive engagement on your platform.
We encourage you to try out the demo and see how file previews can improve your application. If you have any questions or need assistance in implementing this feature, our support team is here to help.
Ready to get started? Sign up for Transloadit and begin integrating file previews into your application today! Happy transcoding :)