Easily integrate subtitles into videos using Lua
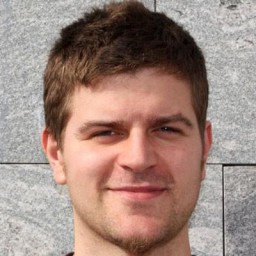
Adding subtitles to videos enhances accessibility and user engagement, making your content reach a broader audience. Lua, a lightweight scripting language, offers a flexible and efficient way to integrate subtitles into your video projects with ease and precision.
The significance of subtitles in video content
Subtitles play a crucial role in today's multimedia landscape. They not only improve accessibility for viewers with hearing impairments but also cater to a global audience by supporting multiple languages. Incorporating subtitles can significantly boost viewer engagement and comprehension, making your content more inclusive and effective.
Lua: a lightweight scripting language
Lua is a powerful yet lightweight scripting language, widely used for embedded applications and extending software functionalities. Known for its simplicity and speed, Lua is an excellent choice for tasks like video processing where performance and flexibility are essential.
Setting up the Lua environment for video processing
Before diving into subtitle integration, you'll need to set up your Lua development environment. Here's how you can get started:
-
Install Lua: Download and install Lua from the official website, or use package managers like
apt
for Ubuntu (sudo apt-get install lua5.3
) orbrew
for macOS (brew install lua
). -
Install FFmpeg: FFmpeg is a powerful multimedia framework used for processing video and audio files. Install it from the official website or via package managers (
sudo apt-get install ffmpeg
orbrew install ffmpeg
). -
Install LuaFFI: To interact with C libraries like FFmpeg, use LuaJIT's FFI library or the Alien library for Lua.
-
Set Up Your Project: Create a new directory for your Lua scripts and set up any necessary environment variables.
Choosing open-source tools for subtitle integration
Integrating subtitles into videos requires tools that can manipulate multimedia files effectively. The following open-source tools are highly recommended:
-
FFmpeg: An indispensable tool for video processing, FFmpeg allows you to manipulate video and audio streams with ease.
-
LuaFFmpeg Bindings: There are Lua bindings available for FFmpeg, such as lua-ffmpeg, which enable you to leverage FFmpeg's capabilities within Lua scripts.
-
Subtitle Libraries: Libraries like luasub can help you parse and work with subtitle files (e.g., SRT files).
Step-by-step guide on adding subtitles using Lua
Let's walk through the process of adding subtitles to a video using Lua and FFmpeg.
Prerequisites:
- Lua installed on your system
- FFmpeg installed
- A video file (
input.mp4
) - A subtitle file in SRT format (
subtitles.srt
)
Step 1: Prepare Your Lua Script
Create a new Lua script file add_subtitles.lua
:
-- add_subtitles.lua
local video_file = "input.mp4"
local subtitles_file = "subtitles.srt"
local output_file = "output.mp4"
-- Build the command to add subtitles using FFmpeg
local command = string.format(
'ffmpeg -i %s -vf subtitles=%s -c:a copy %s',
video_file,
subtitles_file,
output_file
)
-- Execute the command
local result = os.execute(command)
if result then
print("Subtitles added successfully to " .. output_file)
else
print("Failed to add subtitles.")
end
Step 2: Run Your Lua Script
Execute your script from the terminal:
lua add_subtitles.lua
This script uses Lua to construct and execute an FFmpeg command that embeds the subtitles into the video.
Step 3: Verify the Output
After the script finishes, check output.mp4
to ensure the subtitles have been successfully
integrated.
Leveraging Transloadit's video encoding Robot
While scripting can be an effective way to process videos, using a reliable service can simplify the workflow. Transloadit offers a Video Subtitle Robot that allows you to add subtitles to videos seamlessly through their API. Even though there isn't a Lua SDK, you can interact with Transloadit's REST API directly from Lua.
Challenges and solutions in subtitle integration
Integrating subtitles can present several challenges:
-
Subtitle Formatting: Ensuring your subtitle files are correctly formatted is essential. Improper formatting can lead to errors during processing.
-
Encoding Issues: Mismatched character encodings between the video and subtitle files can cause display problems. Make sure both files use the same encoding, preferably UTF-8.
-
Synchronization: Subtitles must be properly synchronized with the video. Tools like Subtitle Edit can help adjust timing.
Solutions:
-
Validate your subtitle files using subtitle editing tools.
-
Use Lua libraries to parse and verify subtitles before integration.
-
Handle exceptions in your script to catch and log errors.
Conclusion
Adding subtitles to videos using Lua is a straightforward process that enhances your content's accessibility and reach. By leveraging open-source tools like FFmpeg and integrating them with Lua scripts, you can automate and customize subtitle integration efficiently. Whether you're processing a single video or batch-processing multiple files, Lua provides the flexibility and performance to meet your needs.
If you're looking for a scalable solution to handle video encoding and subtitle integration, consider using Transloadit, which offers robust APIs to streamline your workflow.