Boost Java performance with memory-mapped files
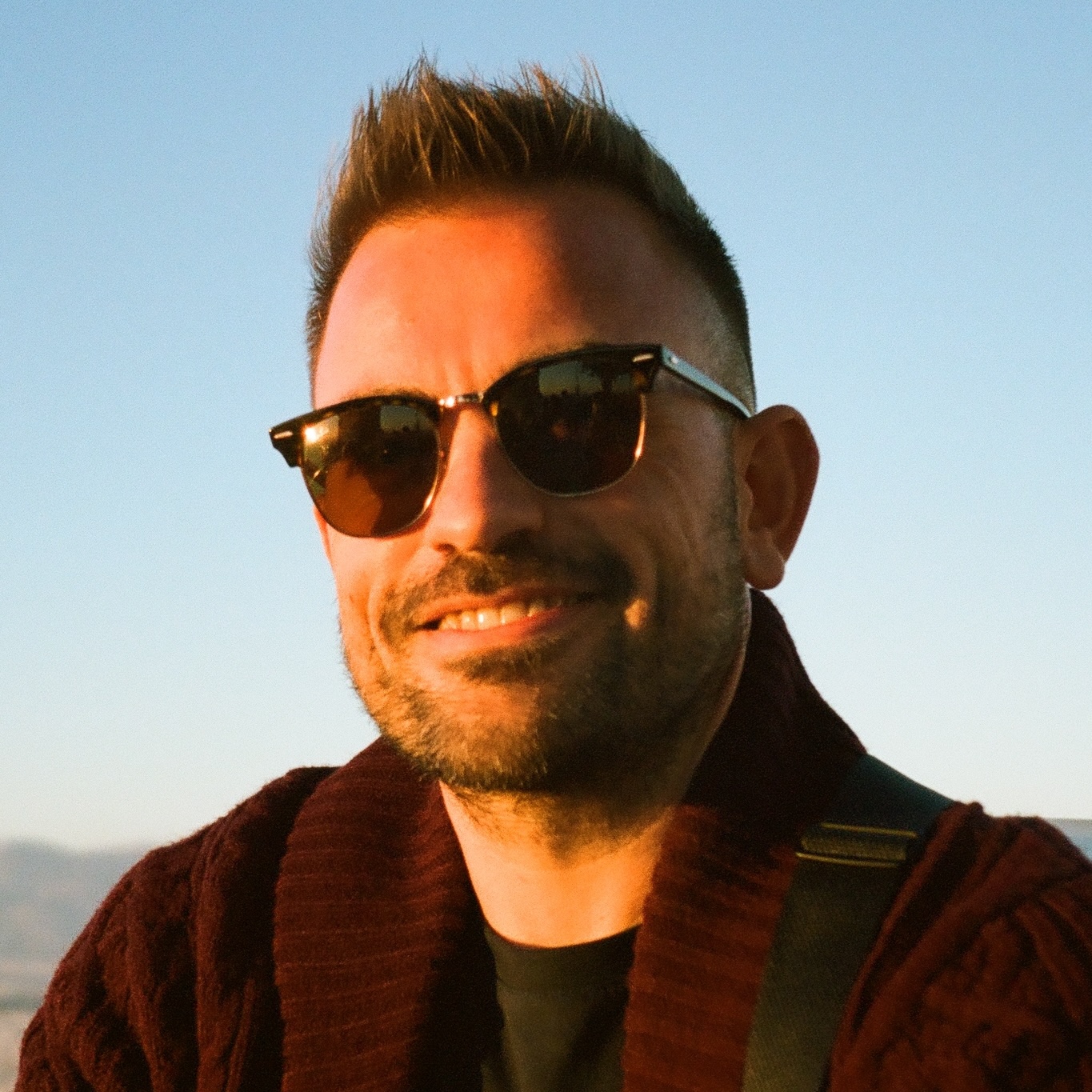
Memory-mapped files allow Java developers to treat file data as if it were in memory, enabling efficient, high-speed file access. This approach can significantly improve performance for large files and random access patterns. Let's explore how to implement memory-mapped file I/O using Java's NIO.2 API and understand when to use this technique.
Understanding memory-mapped files
Memory-mapped files leverage the operating system's virtual memory capabilities to map a file directly into memory. This approach minimizes overhead by reducing system calls and data copying between user space and kernel space. The operating system handles the actual reading and writing of data pages, providing efficient access to file contents.
Important limitations
Before implementing memory-mapped files, be aware of these critical limitations:
- 32-bit systems limit memory-mapped files to 2GB due to addressing constraints
- 64-bit systems are limited by available contiguous virtual memory
- Large files (>2GB) should be split into multiple mapped regions
- Memory-mapped files consume virtual memory address space
Performance considerations
Memory-mapped files excel in specific scenarios:
- Random access patterns benefit most from memory mapping
- Sequential reading of moderate-sized files may perform better with traditional buffered I/O
- Large files requiring frequent random access show significant performance gains
- Multiple processes accessing the same file can share memory pages
Using Java NIO for memory-mapped files
Here's a robust example of reading a file using memory mapping:
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.charset.StandardCharsets;
public class MemoryMappedFileReader {
public static String readFile(String filePath) throws IOException {
File file = new File(filePath);
try (FileInputStream fis = new FileInputStream(file);
FileChannel channel = fis.getChannel()) {
long fileSize = channel.size();
if (fileSize > Integer.MAX_VALUE) {
throw new IllegalArgumentException("File too large to map as a single region");
}
MappedByteBuffer buffer = channel.map(
FileChannel.MapMode.READ_ONLY, 0, fileSize);
byte[] data = new byte[buffer.remaining()];
buffer.get(data);
return new String(data, StandardCharsets.UTF_8);
}
}
public static void main(String[] args) {
try {
String content = readFile("example.txt");
System.out.println("File contents: " + content);
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
} catch (IllegalArgumentException e) {
System.err.println("File size error: " + e.getMessage());
}
}
}
Thread safety considerations
When using memory-mapped files in multi-threaded applications:
- FileChannel operations are thread-safe
- MappedByteBuffer operations are not thread-safe
- Coordinate access to mapped regions between threads explicitly
- Use java.util.concurrent utilities for thread coordination
Common use cases
Memory-mapped files are particularly effective for:
- Database implementations requiring random access
- Large file processing with frequent random access patterns
- Shared memory between processes (platform-dependent)
- Memory-efficient processing of large data files
- Real-time data analysis applications
Best practices
Follow these guidelines for optimal use of memory-mapped files:
- Use try-with-resources to ensure proper resource cleanup
- Consider file size and available memory when choosing mapping strategy
- Implement proper error handling for file size limitations
- Monitor system memory usage when mapping multiple files
- Use direct buffers for large files to avoid copying to JVM heap
Conclusion
Memory-mapped files offer significant performance benefits for specific use cases, particularly when dealing with large files or requiring random access patterns. By understanding the limitations and following best practices, you can effectively leverage this feature in your Java applications. For alternative approaches to file processing, consider exploring Transloadit's /file/read robot, which offers robust document processing capabilities.