Creating a custom file upload UI with JavaScript
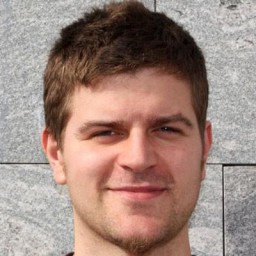
Default file inputs in HTML provide basic functionality but often lack the flexibility and user-friendliness required for modern web applications. In this DevTip, we'll show you how to create a custom file upload UI with JavaScript, enhancing both the appearance and functionality of the standard browser input. By building a custom file uploader, you can offer a more engaging experience to your users, including features like multiple file uploads, file previews, and validation.
Introduction to custom file upload UIs
Creating a custom file upload UI allows you to overcome the limitations of the default input and provide a seamless experience for users. You can integrate the file uploader into your application's design, handle multiple file uploads efficiently, and provide immediate feedback through file previews and custom validation messages.
Limitations of default file input elements
The standard <input type="file">
element presents several challenges:
- Inconsistent styling across browsers
- Limited control over appearance
- Lack of support for advanced features like multiple file selection previews or custom validation messages
Setting up the HTML structure
Let's start by creating a basic HTML structure for our custom file uploader:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Custom File Upload UI</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<div class="file-upload">
<input type="file" id="file-input" multiple />
<label for="file-input">Choose Files</label>
<div id="file-preview"></div>
</div>
<button id="upload-button">Upload Files</button>
<script src="script.js"></script>
</body>
</html>
This structure includes:
- An
<input>
element for file selection with themultiple
attribute to allow selecting multiple files - A
<label>
styled as a button to trigger the file selection dialog - A
<div>
for displaying file previews - An upload button to initiate the file upload process
Styling the file input with CSS
Next, let's style our custom file upload component:
.file-upload {
position: relative;
display: inline-block;
}
#file-input {
display: none;
}
.file-upload label {
display: inline-block;
padding: 10px 20px;
background-color: #007bff;
color: #fff;
cursor: pointer;
border-radius: 5px;
transition: background-color 0.3s;
}
.file-upload label:hover {
background-color: #0056b3;
}
#file-preview {
margin-top: 20px;
display: flex;
flex-wrap: wrap;
gap: 10px;
}
#file-preview img {
width: 100px;
height: 100px;
object-fit: cover;
border-radius: 4px;
}
#upload-button {
margin-top: 20px;
padding: 10px 20px;
background-color: #28a745;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s;
}
#upload-button:hover {
background-color: #218838;
}
This CSS hides the actual file input, styles the label as a button, and provides a layout for file previews. By hiding the default input, we can fully customize the appearance of our file uploader.
Implementing JavaScript for enhanced functionality
Now, let's add JavaScript to handle file selection, preview, validation, and upload.
Handling multiple file uploads
To handle multiple file uploads, we'll add an event listener to the file input:
const fileInput = document.getElementById('file-input')
const preview = document.getElementById('file-preview')
const uploadButton = document.getElementById('upload-button')
let selectedFiles = []
fileInput.addEventListener('change', handleFileSelect)
uploadButton.addEventListener('click', handleUpload)
function handleFileSelect(event) {
preview.innerHTML = ''
selectedFiles = Array.from(event.target.files)
selectedFiles.forEach((file) => {
if (validateFile(file)) {
const reader = new FileReader()
reader.onload = (e) => createPreview(e.target.result, file.name)
reader.readAsDataURL(file)
}
})
}
Displaying selected file previews
To enhance the user experience, we'll display previews of the selected files:
function createPreview(src, alt) {
const img = document.createElement('img')
img.src = src
img.alt = alt
preview.appendChild(img)
}
This function creates an <img>
element for each selected image file and adds it to the preview
container.
Validating file types and sizes
Before uploading, it's important to validate the files:
function validateFile(file) {
const validTypes = ['image/jpeg', 'image/png', 'image/gif']
const maxSize = 2 * 1024 * 1024 // 2 MB
if (!validTypes.includes(file.type)) {
alert(`${file.name} is not a supported file type.`)
return false
}
if (file.size > maxSize) {
alert(`${file.name} exceeds the 2 MB size limit.`)
return false
}
return true
}
This function checks if the file type is supported and if the file size is within the acceptable limit.
Integrating with back-end file upload APIs
Finally, we'll handle the file upload process:
function handleUpload() {
if (selectedFiles.length === 0) {
alert('Please select files to upload.')
return
}
const formData = new FormData()
selectedFiles.forEach((file) => {
formData.append('files[]', file)
})
fetch('/upload', {
method: 'POST',
body: formData,
})
.then((response) => response.json())
.then((data) => {
console.log('Upload successful:', data)
alert('Files uploaded successfully!')
})
.catch((error) => {
console.error('Error:', error)
alert('An error occurred during upload.')
})
}
This script sends the selected files to the server using the Fetch API and FormData. Replace
'/upload'
with your back-end API endpoint.
Conclusion
By creating a custom file upload UI with JavaScript, you can significantly enhance the user experience and provide additional functionalities that default file inputs lack. This approach allows you to implement multiple file uploads, display file previews, validate file inputs, and integrate seamlessly with your back-end.
For those seeking an even more robust and feature-rich solution, consider exploring Uppy, an open-source file uploader for web browsers. Uppy offers advanced features like progress bars, drag-and-drop support, and integrations with various file sources and storage providers.