Efficient Flask file uploads: a step-by-step guide
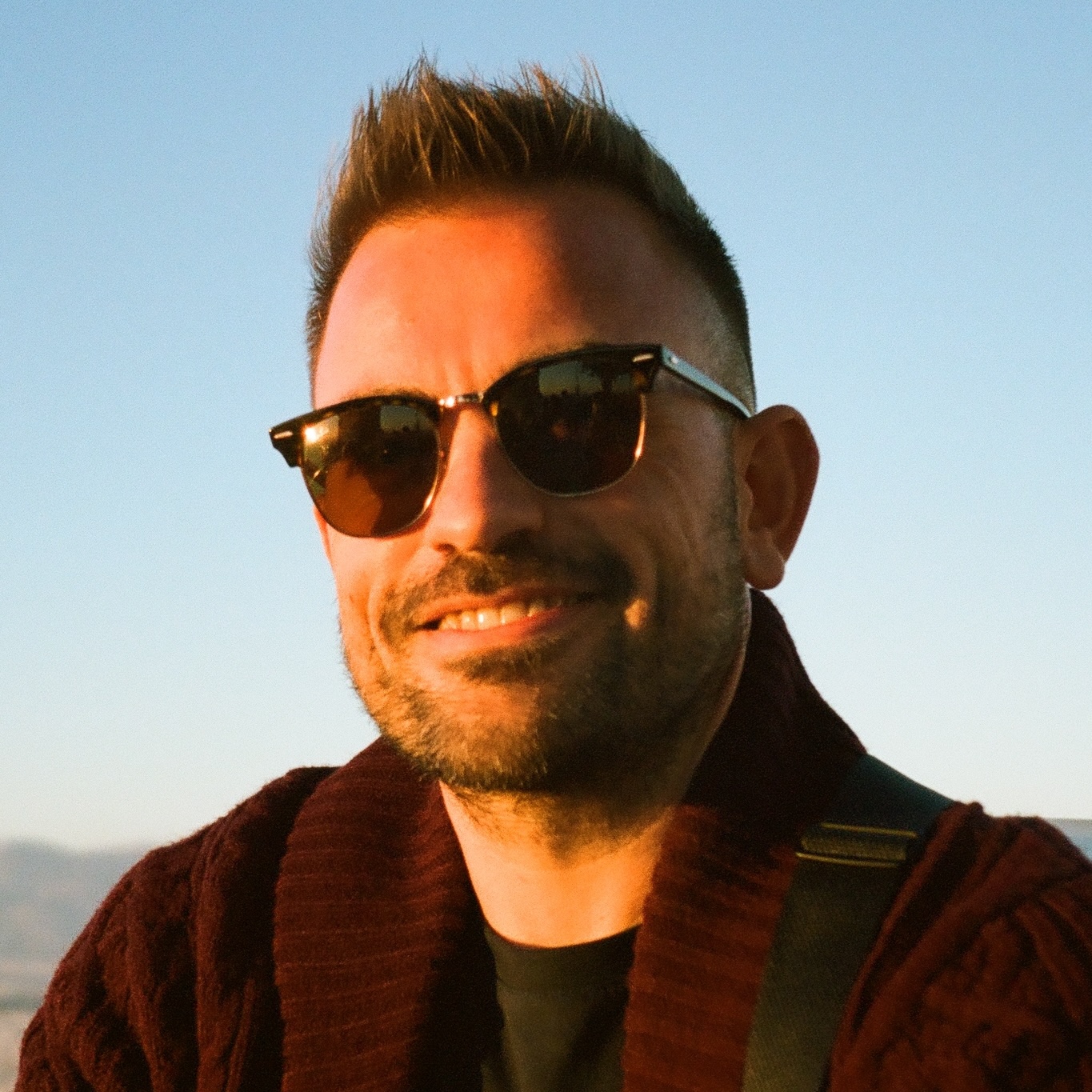
File uploads are a crucial feature in modern web applications, enabling users to share and store data efficiently. Flask, a lightweight yet powerful Python web framework, offers robust capabilities to handle file uploads securely and efficiently. In this step-by-step guide, we'll explore how to implement file uploads in Flask applications, covering best practices, security measures, and advanced techniques.
Setting up your Flask environment
To get started with file uploads in Flask, we'll set up a basic Flask application with the necessary dependencies. Install Flask and Flask-WTF using pip:
pip install flask flask-wtf
Now, let's create a basic Flask application structure in a file named app.py
:
from flask import Flask
import os
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your-secret-key'
app.config['UPLOAD_FOLDER'] = 'uploads'
app.config['MAX_CONTENT_LENGTH'] = 16 * 1024 * 1024 # 16MB max file size
# Ensure the upload folder exists
os.makedirs(app.config['UPLOAD_FOLDER'], exist_ok=True)
Configuration Explained:
-
SECRET_KEY
: A secret key used by Flask-WTF for securely signing the session cookie and can be used for any other security-related needs by extensions or your application. -
UPLOAD_FOLDER
: The directory where uploaded files will be stored. -
MAX_CONTENT_LENGTH
: The maximum size (in bytes) of the uploaded files. Here, it's set to 16 megabytes to prevent oversized uploads.
Building the file upload form
To facilitate file uploads, we'll create a Flask-WTF form that includes validation for the uploaded files.
First, create a form class in forms.py
(or include it in your app.py
):
from flask_wtf import FlaskForm
from flask_wtf.file import FileField, FileRequired, FileAllowed
class UploadForm(FlaskForm):
file = FileField('File', validators=[
FileRequired(),
FileAllowed(['jpg', 'png', 'pdf'], 'Allowed file types are jpg, png, pdf')
])
This form uses FileField
for the file input and includes validators to ensure that a file is
provided and that it has an allowed file extension.
Next, create a template templates/upload.html
for the upload form:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Upload File</title>
</head>
<body>
<h1>Upload File</h1>
<form method="POST" enctype="multipart/form-data">
{{ form.hidden_tag() }} {{ form.file.label }} {{ form.file }}
<input type="submit" value="Upload" />
</form>
{% with messages = get_flashed_messages(with_categories=true) %} {% if messages %}
<ul>
{% for category, message in messages %}
<li class="{{ category }}">{{ message }}</li>
{% endfor %}
</ul>
{% endif %} {% endwith %}
</body>
</html>
This template renders the form and includes a section to display flashed messages for user feedback.
Implementing file upload handling
Now, let's handle the file upload in our Flask application. Update your app.py
to include the
necessary imports and the route for file uploads:
from flask import Flask, render_template, redirect, url_for, flash
from werkzeug.utils import secure_filename
from forms import UploadForm
import os
# ... [previous configuration code]
@app.route('/', methods=['GET', 'POST'])
def upload():
form = UploadForm()
if form.validate_on_submit():
file = form.file.data
filename = secure_filename(file.filename)
file_path = os.path.join(app.config['UPLOAD_FOLDER'], filename)
try:
file.save(file_path)
flash('File uploaded successfully', 'success')
return redirect(url_for('upload'))
except Exception as e:
flash(f'An error occurred while uploading the file: {e}', 'danger')
return redirect(url_for('upload'))
return render_template('upload.html', form=form)
Explanation:
-
secure_filename()
: Sanitizes the filename to prevent directory traversal attacks. -
File Saving: The uploaded file is saved to the specified upload folder.
-
User Feedback: Uses
flash()
to provide success or error messages to the user.
Validating and securing file uploads
To ensure that uploaded files are safe and meet your application's requirements, it's important to implement proper validation and security measures.
File type validation
While the form validators check the file extension, it's more secure to validate the file's content
by checking its MIME type. Install python-magic
to detect the file's MIME type:
pip install python-magic
Update the file handling code in your upload()
route:
import magic
@app.route('/', methods=['GET', 'POST'])
def upload():
form = UploadForm()
if form.validate_on_submit():
file = form.file.data
# Read a small portion of the file for MIME type detection
file_content = file.read(1024)
file.seek(0) # Reset the file pointer after reading
mime = magic.Magic(mime=True)
file_type = mime.from_buffer(file_content)
allowed_types = ['image/jpeg', 'image/png', 'application/pdf']
if file_type not in allowed_types:
flash('Invalid file type', 'danger')
return redirect(url_for('upload'))
filename = secure_filename(file.filename)
file_path = os.path.join(app.config['UPLOAD_FOLDER'], filename)
try:
file.save(file_path)
flash('File uploaded successfully', 'success')
return redirect(url_for('upload'))
except Exception as e:
flash(f'An error occurred while uploading the file: {e}', 'danger')
return redirect(url_for('upload'))
return render_template('upload.html', form=form)
Explanation:
-
MIME Type Checking: Verifies the file's content type to prevent malicious files with disguised extensions.
-
Allowed Types: Only allows files with MIME types matching the specified list.
Limiting file size
The MAX_CONTENT_LENGTH
configuration setting limits the maximum size of uploaded files, helping to
prevent denial-of-service attacks by large uploads.
Handling malicious files
Consider scanning uploaded files with an antivirus tool if your application requires high-security measures.
Creating a REST API endpoint
In some applications, you might need to handle file uploads via a REST API. Let's implement an API endpoint for file uploads.
from flask import request, jsonify
@app.route('/api/upload', methods=['POST'])
def api_upload():
if 'file' not in request.files:
return jsonify({'error': 'No file part in the request'}), 400
file = request.files['file']
if file.filename == '':
return jsonify({'error': 'No file selected for uploading'}), 400
# Validate file type as before
file_content = file.read(1024)
file.seek(0)
mime = magic.Magic(mime=True)
file_type = mime.from_buffer(file_content)
allowed_types = ['image/jpeg', 'image/png', 'application/pdf']
if file_type not in allowed_types:
return jsonify({'error': 'Invalid file type'}), 400
filename = secure_filename(file.filename)
file_path = os.path.join(app.config['UPLOAD_FOLDER'], filename)
try:
file.save(file_path)
return jsonify({'message': 'File uploaded successfully', 'filename': filename}), 200
except Exception as e:
return jsonify({'error': str(e)}), 500
Testing the API Endpoint:
Use cURL or Postman to test the API endpoint.
Using cURL:
curl -F 'file=@/path/to/your/file.jpg' http://localhost:5000/api/upload
Handling large file uploads
Uploading large files can pose challenges due to server memory limitations and potential timeouts. Here are some strategies to handle large file uploads:
Increase file size limit
Adjust the MAX_CONTENT_LENGTH
setting if necessary, but be cautious about server capacity.
Implement chunked uploads
Implementing chunked uploads allows users to upload large files in smaller parts. While Flask doesn't provide this functionality out of the box, you can use Flask extensions or client-side JavaScript libraries to handle chunked uploads.
Use asynchronous tasks
Offload file processing to background tasks using a tool like Celery. This prevents the request from timing out and improves user experience.
Leverage cloud storage
Store uploaded files directly to cloud storage services like Amazon S3 or Google Cloud Storage using
extensions like Flask-S3
or Flask-GoogleCloud
. This approach offloads storage and bandwidth to
cloud services.
Error handling and validation
Implement robust error handling to improve the reliability and user experience of your application.
Handle file size errors
from werkzeug.exceptions import RequestEntityTooLarge
@app.errorhandler(RequestEntityTooLarge)
def handle_file_too_large(e):
return 'File Too Large', 413
General exception handling
@app.errorhandler(Exception)
def handle_unexpected_error(error):
return 'An unexpected error occurred: {}'.format(str(error)), 500
Conclusion
Implementing file uploads in Flask requires careful consideration of security, performance, and user experience. By following these best practices and implementing proper validation and error handling, you can create a robust file upload system for your Flask applications.
For more advanced file upload capabilities, consider using open-source tools like Tus or Uppy, which can be integrated with Flask to provide features like resumable uploads and progress tracking. Additionally, Transloadit offers robust file uploading and processing services that can further enhance your application's capabilities.