Efficient image hosting: CDNs & processing
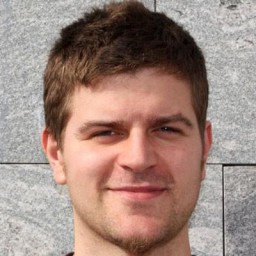
Static CDNs (Content Delivery Networks) are a popular choice for developers seeking efficient image hosting and delivery. Combining static CDNs with dynamic image processing enhances application performance and reduces bandwidth costs.
Introduction to image hosting with static CDNs
Static CDNs store and deliver static assets like images, CSS, and JavaScript files from geographically distributed servers. This ensures faster load times and an improved user experience by serving content from the server closest to the user.
Why use static CDNs for image hosting?
Static CDNs offer several advantages:
- Improved Performance: Reduced latency and faster load times.
- Scalability: Easily handle traffic spikes.
- Cost Efficiency: Lower bandwidth costs.
Key benefits of static CDNs for developers
- Simplified Infrastructure: No need for complex server setups.
- Global Reach: Serve content globally with minimal latency.
- Reliability: Built-in redundancy and failover.
Set up an image hosting CDN: step-by-step guide
Here's how to set up your CDN:
-
Choose a CDN Provider: Options include Cloudflare (Free tier: basic CDN and DDoS protection; Pro plan at $20/month: image optimization), AWS CloudFront, and Fastly.
-
Configure Origin Server: Set up storage (e.g., AWS S3, Google Cloud Storage).
# Example: Create an S3 bucket with public read access aws s3 mb s3://your-image-bucket --region us-east-1 aws s3api put-bucket-policy --bucket your-image-bucket --policy '{"Version":"2012-10-17","Statement":[{"Effect":"Allow","Principal":"*","Action":"s3:GetObject","Resource":"arn:aws:s3:::your-image-bucket/*"}]}'
-
Set Up CDN Distribution: Point your CDN to your storage.
# Example: Create a CloudFront distribution with AWS CLI aws cloudfront create-distribution \ --origin-domain-name your-image-bucket.s3.amazonaws.com \ --default-cache-behavior '{"TargetOriginId":"S3-your-image-bucket","ViewerProtocolPolicy":"redirect-to-https","AllowedMethods":{"Quantity":2,"Items":["GET","HEAD"]},"CachedMethods":{"Quantity":2,"Items":["GET","HEAD"]},"ForwardedValues":{"QueryString":true,"Cookies":{"Forward":"none"}}}'
-
Configure DNS: Update DNS records to route traffic through the CDN.
# Example: Add a CNAME record with AWS Route 53 aws route53 change-resource-record-sets \ --hosted-zone-id YOUR_HOSTED_ZONE_ID \ --change-batch '{"Changes":[{"Action":"CREATE","ResourceRecordSet":{"Name":"images.yourdomain.com","Type":"CNAME","TTL":300,"ResourceRecords":[{"Value":"d123456abcdef8.cloudfront.net"}]}}]}'
Integrate on-the-fly image processing: save time and bandwidth
Dynamic image processing allows real-time resizing, cropping, compression, and optimization. This reduces storage and bandwidth.
Example: real-time image resizing
Implement responsive images with modern formats:
<picture>
<source
srcset="
https://cdn.example.com/image.jpg?width=800&format=webp 800w,
https://cdn.example.com/image.jpg?width=400&format=webp 400w
"
sizes="(max-width: 800px) 100vw, 800px"
type="image/webp"
/>
<img
src="https://cdn.example.com/image.jpg?width=800"
srcset="
https://cdn.example.com/image.jpg?width=800 800w,
https://cdn.example.com/image.jpg?width=400 400w
"
sizes="(max-width: 800px) 100vw, 800px"
loading="lazy"
alt="Optimized responsive image"
/>
</picture>
Tools and libraries for image processing
Several tools can help with dynamic image processing:
Sharp (Node.js)
Sharp is a high-performance image processing library for Node.js (version 0.33.5 as of writing):
// Modern example with WebP support and error handling
const sharp = require('sharp')
async function processImage(input, output, options = {}) {
try {
const { width = 800, height = null, quality = 80, format = 'webp' } = options
await sharp(input)
.resize(width, height, {
fit: 'inside',
withoutEnlargement: true,
})
.webp({ quality })
.toFile(output)
console.log(`Image processed successfully: ${output}`)
return true
} catch (error) {
console.error('Image processing failed:', error)
return false
}
}
// Usage example
processImage('original.jpg', 'processed.webp', {
width: 1200,
quality: 85,
})
ImageMagick
A versatile command-line tool:
# Convert and optimize an image for web
magick convert original.jpg -resize "800x600>" -quality 85 -strip -format webp optimized.webp
Cloudinary
A cloud-based service with a URL-based API:
<!-- Cloudinary example with automatic format and quality -->
<img
src="https://res.cloudinary.com/demo/image/upload/f_auto,q_auto,w_800/sample.jpg"
alt="Cloudinary optimized image"
/>
Best practices for optimizing image delivery via CDN
-
Use Modern Formats: Serve WebP with JPEG fallback.
-
Implement Responsive Images: Use
srcset
andsizes
. -
Enable Lazy Loading: Use
loading="lazy"
. -
Set Cache Headers: Configure long cache times.
# Nginx configuration for image caching location ~* \.(jpg|jpeg|png|gif|webp)$ { expires 1y; add_header Cache-Control "public, no-transform"; }
-
Optimize Quality: Balance quality and file size (80-85% for JPEG/WebP).
-
Monitor Core Web Vitals: Track Largest Contentful Paint (LCP) and Cumulative Layout Shift (CLS).
Security considerations when using CDNs
-
Use HTTPS: Serve images securely.
-
Implement Signed URLs: Restrict access with time-limited URLs.
// AWS S3 signed URL example const AWS = require('aws-sdk') const s3 = new AWS.S3() function getSignedImageUrl(bucket, key, expirationSeconds = 3600) { const params = { Bucket: bucket, Key: key, Expires: expirationSeconds, } return s3.getSignedUrl('getObject', params) } // Usage const imageUrl = getSignedImageUrl('your-bucket', 'private/image.jpg', 1800) console.log(imageUrl)
-
Configure Security Headers: Implement
Content-Security-Policy
.# Nginx configuration for security headers add_header Content-Security-Policy "img-src 'self' https: data:; default-src 'none'"; add_header X-Content-Type-Options "nosniff"; add_header X-Frame-Options "DENY";
-
Audit Permissions: Ensure proper access controls.
-
Validate Image Content: Implement server-side validation.
Troubleshoot common CDN image hosting challenges
Cache invalidation
When updates aren't reflected:
# AWS cloudfront invalidation example
aws cloudfront create-invalidation \
--distribution-id DISTRIBUTION_ID \
--paths "/images/*"
Image load error handling
Implement client-side fallbacks:
// Image load error handling with format fallback
function handleImageError(img) {
// Try WebP fallback
if (img.src.endsWith('webp')) {
console.log(`WebP failed, trying JPEG: ${img.src}`)
img.src = img.src.replace('.webp', '.jpg')
return
}
// Log to monitoring
console.error(`Image load failed: ${img.src}`)
// Show fallback
img.src = '/assets/fallback-image.jpg'
img.setAttribute('data-load-failed', 'true')
}
// Add to images
document.addEventListener('DOMContentLoaded', () => {
const images = document.querySelectorAll('img')
images.forEach((img) => {
img.onerror = () => handleImageError(img)
})
})
Performance monitoring
Track image loading with the Performance API:
// Performance monitoring for image loading
if ('PerformanceObserver' in window) {
const observer = new PerformanceObserver((list) => {
for (const entry of list.getEntries()) {
if (entry.element?.tagName === 'IMG') {
console.log(`Image ${entry.element.src} LCP: ${entry.startTime}ms`)
// Send to analytics
if (typeof gtag === 'function') {
gtag('event', 'lcp_image', {
image_url: entry.element.src,
load_time: entry.startTime,
})
}
}
}
})
observer.observe({ entryTypes: ['largest-contentful-paint'] })
}
By combining static CDNs with dynamic image processing, you can enhance performance, scalability, and cost-efficiency. These techniques will help you deliver optimized images while maintaining quality. For more complex needs, consider Transloadit's content delivery service.