Efficiently handle Zip files in your dev workflow with unzip
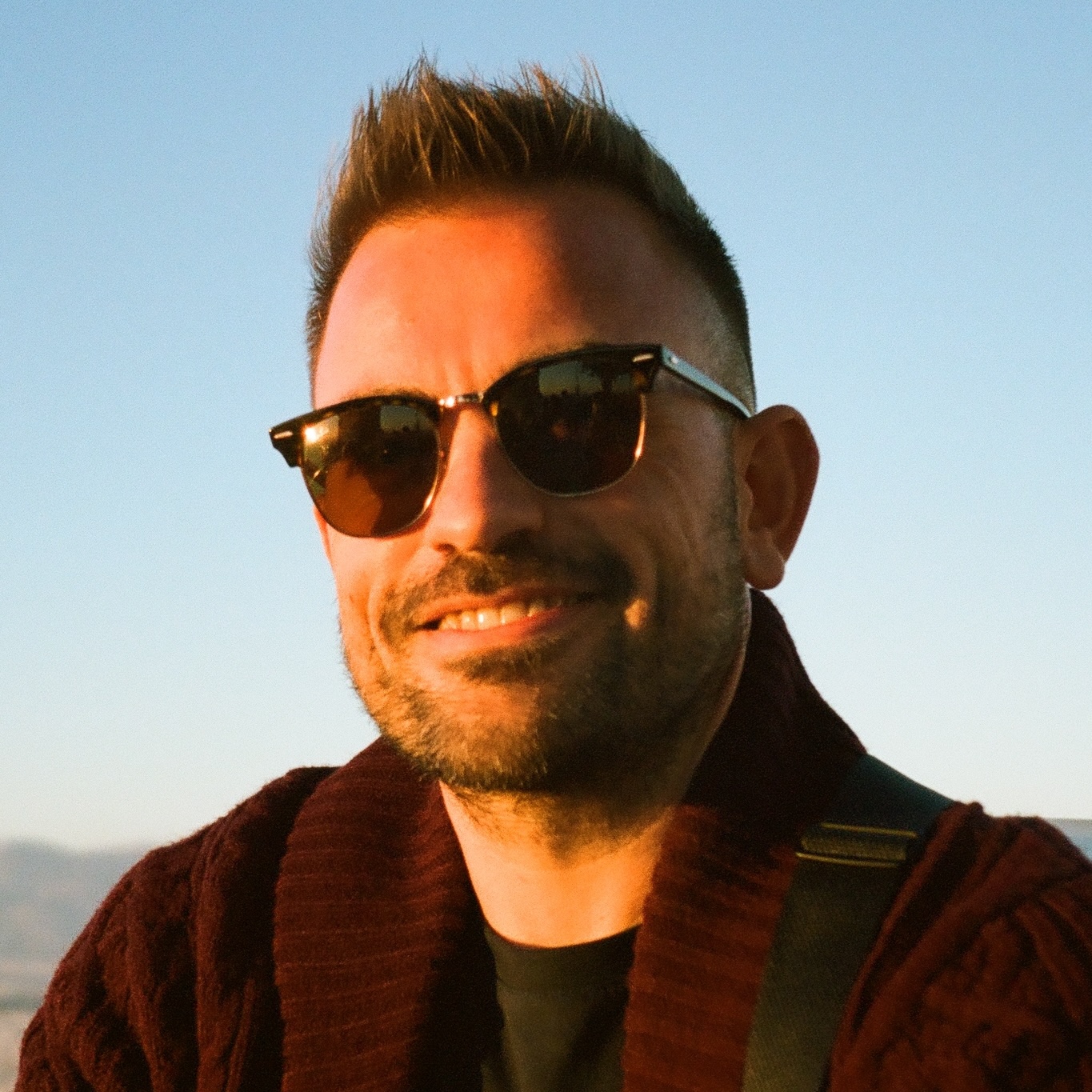
The unzip command-line tool is an essential utility for developers who regularly work with compressed files. Whether you're handling project dependencies, managing deployments, or processing user uploads, mastering unzip can significantly improve your development workflow.
Install unzip on your system
Unzip is available on most operating systems. Here's how to install it:
-
macOS:
brew install unzip
-
Linux:
For Debian/Ubuntu:
sudo apt-get update && sudo apt-get install unzip
For Fedora:
sudo dnf install unzip
-
Windows:
- Download and install 7-Zip for a GUI interface
- Use Windows Subsystem for Linux (WSL) to access the unzip command
Basic command usage
The unzip command offers straightforward syntax for common operations:
# Extract all files
unzip archive.zip
# List contents without extracting
unzip -l archive.zip
# Extract to a specific directory
unzip archive.zip -d /path/to/directory
# Extract a specific file
unzip archive.zip file.txt
Advanced techniques
Here are some powerful features for more complex scenarios:
# Extract while excluding specific files
unzip archive.zip -x "*.log" "*.tmp"
# Extract only files matching a pattern
unzip archive.zip "*.js" "*.ts"
# Overwrite files without prompting
unzip -o archive.zip
# Skip existing files
unzip -n archive.zip
# Print extracted files to stdout
unzip -p archive.zip config.json
Automation with unzip
Integrate unzip into your development scripts for automated workflows:
#!/bin/bash
# Function to handle Zip files in a directory
process_zips() {
local target_dir="$1"
local extract_dir="$2"
# Create extraction directory if it doesn't exist
mkdir -p "$extract_dir"
# Process each zip file
for zip_file in "$target_dir"/*.zip; do
if [ -f "$zip_file" ]; then
# Create a subdirectory based on the zip filename
filename=$(basename "$zip_file" .zip)
mkdir -p "$extract_dir/$filename"
# Extract and log
echo "Processing: $zip_file"
unzip -q "$zip_file" -d "$extract_dir/$filename"
# Optional: Remove the zip file after extraction
# rm "$zip_file"
fi
done
}
# Usage example
process_zips "./downloads" "./extracted"
Common use cases
Developers frequently use unzip for:
-
Extracting downloaded dependencies
curl -L https://example.com/package.zip -o package.zip && unzip package.zip
-
Processing multiple archives
find . -name "*.zip" -exec unzip {} \;
-
Inspecting package contents
unzip -l package.zip | grep -i "license"
-
Testing zip file integrity
unzip -t archive.zip
Error handling
Implement robust error handling in your scripts:
#!/bin/bash
handle_zip() {
local zip_file="$1"
local extract_dir="$2"
if ! command -v unzip >/dev/null 2>&1; then
echo "Error: unzip is not installed"
return 1
fi
if [ ! -f "$zip_file" ]; then
echo "Error: $zip_file not found"
return 1
fi
# Test zip file integrity
if ! unzip -t "$zip_file" >/dev/null 2>&1; then
echo "Error: $zip_file is corrupted"
return 1
fi
# Attempt extraction
if unzip -q "$zip_file" -d "$extract_dir"; then
echo "Successfully extracted $zip_file"
return 0
else
echo "Failed to extract $zip_file"
return 1
fi
}
Conclusion
The unzip command-line tool is a powerful utility for managing compressed files in your development workflow. By mastering its features and incorporating it into your automation scripts, you can handle file extraction tasks efficiently and reliably.
For automated file processing workflows, including zip file handling, check out Transloadit's file processing service with its robust file importing and filtering capabilities.