Effortless audio encoding in the browser with WebAssembly
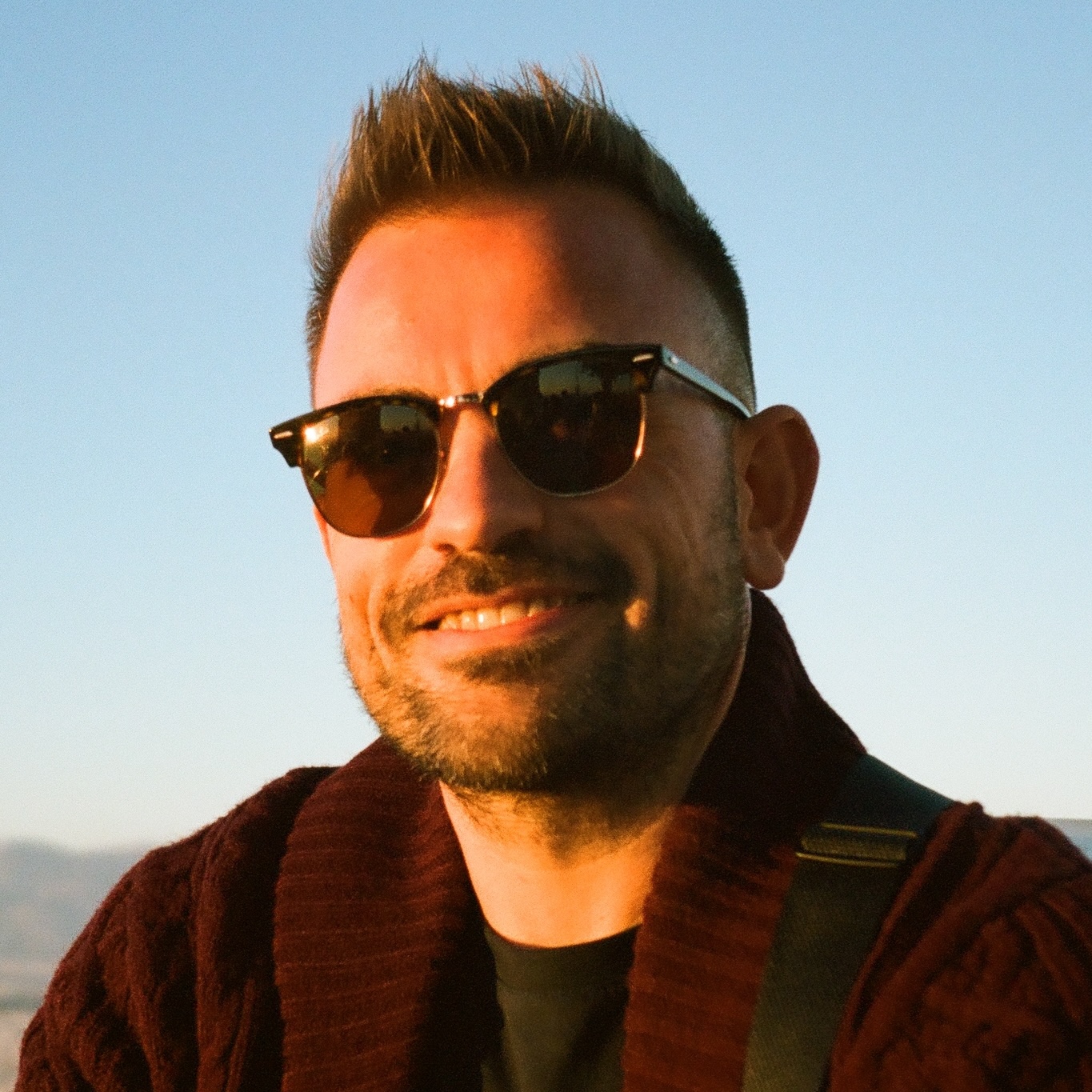
Encoding audio in browsers has evolved significantly over the years. With the introduction of WebAssembly, developers can now perform complex audio processing tasks directly in web applications. In this post, we'll explore how you can harness WebAssembly to encode audio efficiently in browsers.
Introduction to browser-based audio encoding
Traditionally, audio encoding was performed on the server side due to the limitations of JavaScript in handling intensive computational tasks. However, with the increasing need for responsive and interactive web applications, offloading some of these tasks to the client side has become advantageous.
The role of WebAssembly in audio processing
WebAssembly (Wasm) is a low-level bytecode format that runs in the browser at near-native speed. It allows developers to compile code written in languages like C, C++, or Rust into a format that can be executed efficiently in the browser. This is particularly useful for tasks like audio encoding, which require high performance.
Benefits of using WebAssembly for audio encoding
-
Performance: WebAssembly runs at speeds comparable to native applications, significantly outperforming traditional JavaScript for compute-intensive tasks.
-
Efficiency: By performing encoding on the client side, you can reduce server load and bandwidth usage, leading to cost savings.
-
Flexibility: WebAssembly allows you to use existing audio encoding libraries written in languages like C or Rust, expanding the tools at your disposal.
Setting up a simple web application for audio encoding
Let's start by setting up a basic web application that will handle audio encoding in the browser.
Prerequisites
-
Basic knowledge of JavaScript and HTML.
-
Node.js and npm installed on your machine.
Project setup
Create a new directory for your project and initialize it:
mkdir webassembly-audio-encoder
cd webassembly-audio-encoder
npm init -y
Install the necessary dependencies:
npm install @ffmpeg/ffmpeg @ffmpeg/core
Development server setup
Since we're using modules and WASM files, you'll need a proper development server. Create a simple server using Express:
npm install express
Create server.js
:
const express = require('express')
const app = express()
app.use(express.static('.'))
// Required CORS headers for WASM
app.use((req, res, next) => {
res.header('Cross-Origin-Opener-Policy', 'same-origin')
res.header('Cross-Origin-Embedder-Policy', 'require-corp')
next()
})
app.listen(3000, () => {
console.log('Server running at http://localhost:3000')
})
Add to your package.json
:
{
"scripts": {
"start": "node server.js"
}
}
Run with:
npm start
Ffmpeg.wasm setup and usage
After installing the dependencies, create an index.html
file:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>FFmpeg.wasm Audio Encoder</title>
</head>
<body>
<input type="file" id="uploader" accept="audio/*" />
<button id="encodeButton">Encode Audio</button>
<div id="progress"></div>
<script type="module" src="index.js"></script>
</body>
</html>
Create an index.js
file to handle the FFmpeg.wasm implementation:
// Import the required FFmpeg.wasm modules
import { FFmpeg } from '@ffmpeg/ffmpeg'
import { toBlobURL } from '@ffmpeg/util'
// Create FFmpeg instance
const ffmpeg = new FFmpeg()
// Initialize FFmpeg.wasm
async function init() {
const baseURL = 'https://unpkg.com/@ffmpeg/core@0.12.4/dist/umd'
await ffmpeg.load({
coreURL: await toBlobURL(`${baseURL}/ffmpeg-core.js`, 'text/javascript'),
wasmURL: await toBlobURL(`${baseURL}/ffmpeg-core.wasm`, 'application/wasm'),
})
console.log('FFmpeg is ready!')
}
// Handle file encoding
async function encodeFile() {
const uploader = document.getElementById('uploader')
const progressDiv = document.getElementById('progress')
if (!uploader.files.length) {
alert('Please select an audio file')
return
}
try {
// Initialize if not already done
if (!ffmpeg.loaded) {
await init()
}
const file = uploader.files[0]
const inputFileName = file.name
const outputFileName = 'output.mp3'
// Convert file to Uint8Array
const data = await file.arrayBuffer()
const inputData = new Uint8Array(data)
// Write input file to FFmpeg's virtual filesystem
await ffmpeg.writeFile(inputFileName, inputData)
// Set up progress handler
ffmpeg.on('progress', ({ progress, time }) => {
progressDiv.textContent = `Progress: ${(progress * 100).toFixed(2)}%`
})
// Run FFmpeg command
await ffmpeg.exec([
'-i',
inputFileName,
'-c:a',
'libmp3lame', // Use MP3 codec
'-b:a',
'192k', // Set bitrate
outputFileName,
])
// Read the output file
const outputData = await ffmpeg.readFile(outputFileName)
// Create download link
const blob = new Blob([outputData], { type: 'audio/mp3' })
const url = URL.createObjectURL(blob)
const a = document.createElement('a')
a.href = url
a.download = outputFileName
a.click()
// Cleanup
URL.revokeObjectURL(url)
} catch (error) {
if (!(error instanceof Error)) {
throw new Error(`Was thrown a non-error: ${error}`)
}
console.error('Error during encoding:', error)
alert('Error encoding file: ' + error.message)
}
}
// Add click handler
document.getElementById('encodeButton').addEventListener('click', encodeFile)
This implementation:
- Properly imports and initializes FFmpeg.wasm
- Handles file input and conversion
- Shows progress during encoding
- Creates a downloadable output file
- Includes proper error handling
- Cleans up resources after use
Direct WebAssembly implementation
Let's look at a simple audio processing example using WebAssembly directly. We'll create a module that applies gain to audio samples.
First, create a C file audio_processor.c
:
#include <emscripten.h>
EMSCRIPTEN_KEEPALIVE
void apply_gain(float* samples, int length, float gain) {
for (int i = 0; i < length; i++) {
samples[i] *= gain;
}
}
Compile it to WebAssembly using Emscripten:
emcc audio_processor.c -o audio_processor.wasm -O3 \
-s WASM=1 \
-s EXPORTED_FUNCTIONS='["_apply_gain"]' \
-s EXPORTED_RUNTIME_METHODS='["ccall", "cwrap"]'
Then use it in JavaScript:
let wasmInstance = null
async function initWasm() {
const response = await fetch('audio_processor.wasm')
const wasmBytes = await response.arrayBuffer()
const wasmModule = await WebAssembly.instantiate(wasmBytes, {
env: {
memory: new WebAssembly.Memory({ initial: 256 }),
},
})
wasmInstance = wasmModule.instance
}
async function processAudio(audioBuffer, gain) {
if (!wasmInstance) await initWasm()
const samples = audioBuffer.getChannelData(0)
const wasmMemory = new Float32Array(wasmInstance.exports.memory.buffer)
// Copy samples to WebAssembly memory
wasmMemory.set(samples)
// Process the audio
wasmInstance.exports.apply_gain(wasmMemory.byteOffset, samples.length, gain)
// Copy processed samples back
samples.set(wasmMemory.subarray(0, samples.length))
return audioBuffer
}
This example demonstrates:
- Direct WebAssembly compilation from C code
- Memory management between JavaScript and WebAssembly
- Real-time audio processing using WebAssembly
Integrating WebAssembly with JavaScript for enhanced audio features
By integrating WebAssembly modules with JavaScript and the Web Audio API, you can create rich audio processing applications entirely in the browser. The Web Audio API provides a powerful and versatile system for controlling audio, allowing developers to create complex audio synthesis and processing functions directly in their web applications.
Additional audio processing
You can extend the encoder to perform various operations, such as:
-
Changing Bitrate:
await ffmpeg.exec(['-i', file.name, '-b:a', '192k', 'output.mp3'])
-
Converting to Different Formats:
await ffmpeg.exec(['-i', file.name, 'output.ogg'])
-
Extracting Audio from Video:
await ffmpeg.exec(['-i', file.name, '-q:a', '0', '-map', 'a', 'output.mp3'])
-
Applying Audio Filters: Using FFmpeg audio filters to modify audio.
await ffmpeg.exec(['-i', file.name, '-af', 'volume=1.5', 'output.mp3'])
Testing and optimizing audio encoding performance
Testing is crucial to ensure that the encoding process works smoothly across different browsers and devices.
Performance considerations
-
Web Workers: Offload encoding to a Web Worker to prevent blocking the main UI thread.
const worker = new Worker('encoder-worker.js')
-
Progress Feedback: Use
ffmpeg.setProgress()
to provide progress updates to the user.ffmpeg.setProgress(({ ratio }) => { console.log(`Encoding progress: ${Math.round(ratio * 100)}%`) })
Challenges and solutions
Implementing audio encoding in browsers presents several challenges:
-
Browser Compatibility: Not all browsers fully support WebAssembly or the Web Audio API. Ensure your application checks for support and provides fallbacks or friendly messages.
if (typeof WebAssembly !== 'object' || !window.AudioContext) { alert('Your browser does not support the necessary features for this application.') }
-
Performance Constraints: Encoding large audio files can be resource-intensive. Optimize performance by:
- Using Web Workers to prevent UI blocking.
- Processing smaller files or chunks.
- Providing feedback to users during encoding.
-
Security Considerations: Handle user files securely.
- Only access files explicitly provided by the user.
- Avoid uploading sensitive data to remote servers without user consent.
- Respect user privacy and security best practices.
Deploying and future-proofing your web audio application
Handling browser compatibility
Ensure your application checks for the necessary features:
if (typeof WebAssembly === 'object' && window.AudioContext) {
// Proceed with application
} else {
alert('Your browser does not support WebAssembly or the Web Audio API.')
}
Security considerations
-
User Permissions: Access only files that users select.
-
CORS Policies: If fetching resources, ensure your server's CORS policies allow cross-origin requests.
-
HTTPS: Host your application over HTTPS to ensure secure communication.
Conclusion
Encoding audio in browsers using WebAssembly empowers developers to create powerful and efficient
web applications with advanced audio processing capabilities. By leveraging tools like ffmpeg.wasm
and integrating with the Web Audio API, you can perform tasks previously limited to server-side or
native applications.
If you're looking for a solution that handles audio encoding and processing efficiently, Transloadit offers robust audio encoding services that could complement your application's needs.