Encode audio with cURL and open-source tools
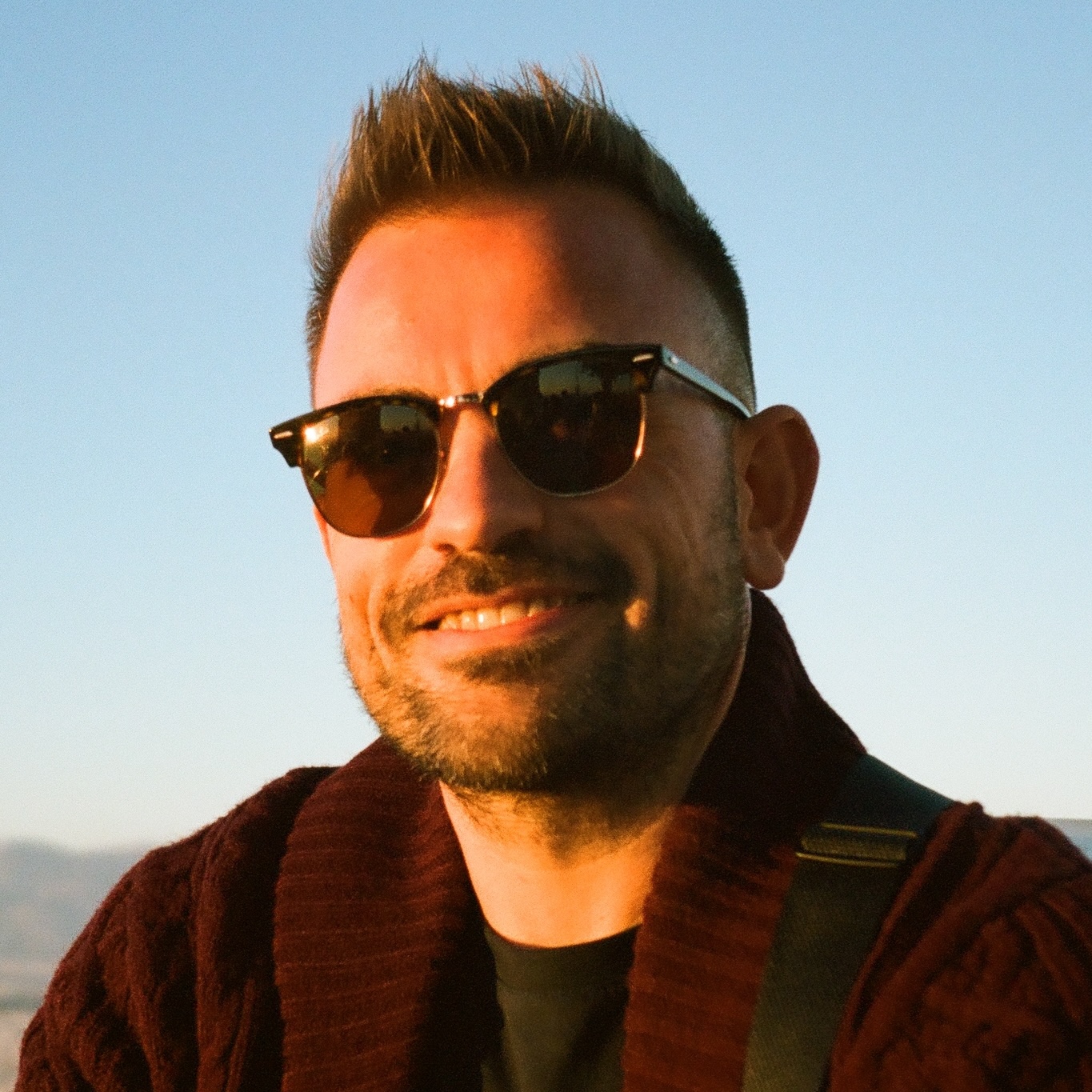
Audio encoding on the command line can be powerful and efficient when you combine cURL with open-source tools. This guide shows you how to create robust audio processing workflows using cURL and FFmpeg.
Set up your environment
First, ensure you have the necessary tools installed. You'll need cURL (typically pre-installed on most systems) and FFmpeg for audio processing.
Install FFmpeg:
# macOS
brew install ffmpeg
# Debian/Ubuntu
sudo apt-get update
sudo apt-get install ffmpeg
# Fedora
sudo dnf install ffmpeg
# Arch Linux
sudo pacman -S ffmpeg
# Windows
winget install Gyan.FFmpeg
Basic audio encoding with FFmpeg and cURL
Let's start with a simple example of downloading and encoding an audio file:
# Download an audio file
curl -o input.mp3 https://example.com/audio.mp3
# Convert to aac format
ffmpeg -i input.mp3 -c:a aac -b:a 192k output.m4a
# Upload the encoded file
curl -F "file=@output.m4a" https://example.com/upload
Create an audio encoding script
Here's a more advanced script that handles various audio formats and includes error checking:
#!/bin/bash
if [ "$#" -ne 3 ]; then
echo "Usage: $0 <input_url> <output_format> <output_bitrate>"
echo "Example: $0 https://example.com/audio.mp3 aac 192k"
exit 1
}
INPUT_URL=$1
OUTPUT_FORMAT=$2
BITRATE=$3
# Generate unique filenames
TIMESTAMP=$(date +%s)
INPUT_FILE="input_${TIMESTAMP}.audio"
OUTPUT_FILE="output_${TIMESTAMP}.${OUTPUT_FORMAT}"
# Download the input file
echo "Downloading input file..."
curl -s -f -o "${INPUT_FILE}" "${INPUT_URL}"
if [ $? -ne 0 ]; then
echo "Error: Failed to download input file"
exit 1
}
# Encode the audio
echo "Encoding to ${OUTPUT_FORMAT} format..."
ffmpeg -i "${INPUT_FILE}" -c:a "${OUTPUT_FORMAT}" -b:a "${BITRATE}" "${OUTPUT_FILE}" -y 2>/dev/null
if [ $? -ne 0 ]; then
echo "Error: Failed to encode audio"
rm "${INPUT_FILE}"
exit 1
}
# Clean up input file
rm "${INPUT_FILE}"
echo "Successfully encoded to: ${OUTPUT_FILE}"
Batch processing audio files
For processing multiple files, here's a script that handles batch encoding:
#!/bin/bash
if [ "$#" -ne 1 ]; then
echo "Usage: $0 <input_file_list.txt>"
echo "File list format: <input_url> <output_format> <bitrate>"
exit 1
}
INPUT_LIST=$1
while IFS=' ' read -r url format bitrate; do
echo "Processing: ${url}"
# Generate output filename from URL
filename=$(basename "${url}")
basename="${filename%.*}"
output="encoded_${basename}.${format}"
# Download and encode
curl -s -f "${url}" | \
ffmpeg -i pipe:0 -c:a "${format}" -b:a "${bitrate}" \
-f "${format}" "${output}" 2>/dev/null
if [ $? -eq 0 ]; then
echo "Success: ${output}"
else
echo "Failed: ${url}"
fi
done < "${INPUT_LIST}"
Security considerations
When working with remote files and APIs:
# Use environment variables for sensitive data
export API_TOKEN="your_secret_token"
curl -H "Authorization: Bearer ${API_TOKEN}" https://api.example.com/upload
# Verify SSL certificates
curl --cacert /path/to/certificate.pem https://api.example.com/audio
# Rate limiting for batch operations
sleep 1 # Add delay between requests
Error handling and validation
Implement proper error checking in your workflows:
# Check audio file validity
ffmpeg -v error -i input.mp3 -f null - 2>error.log
if [ -s error.log ]; then
echo "Invalid audio file"
exit 1
}
# Verify successful upload
HTTP_STATUS=$(curl -s -o /dev/null -w "%{http_code}" -F "file=@output.m4a" https://example.com/upload)
if [ "${HTTP_STATUS}" -ne 200 ]; then
echo "Upload failed with status: ${HTTP_STATUS}"
exit 1
}
Conclusion
Combining cURL with FFmpeg provides a powerful foundation for audio processing workflows. These tools enable you to create efficient, automated solutions for audio encoding tasks.
For more advanced audio processing capabilities, consider exploring Transloadit's /audio/encode Robot, which provides similar functionality with additional features and scalability.