Export files to Amazon S3 in Node.js: a complete guide
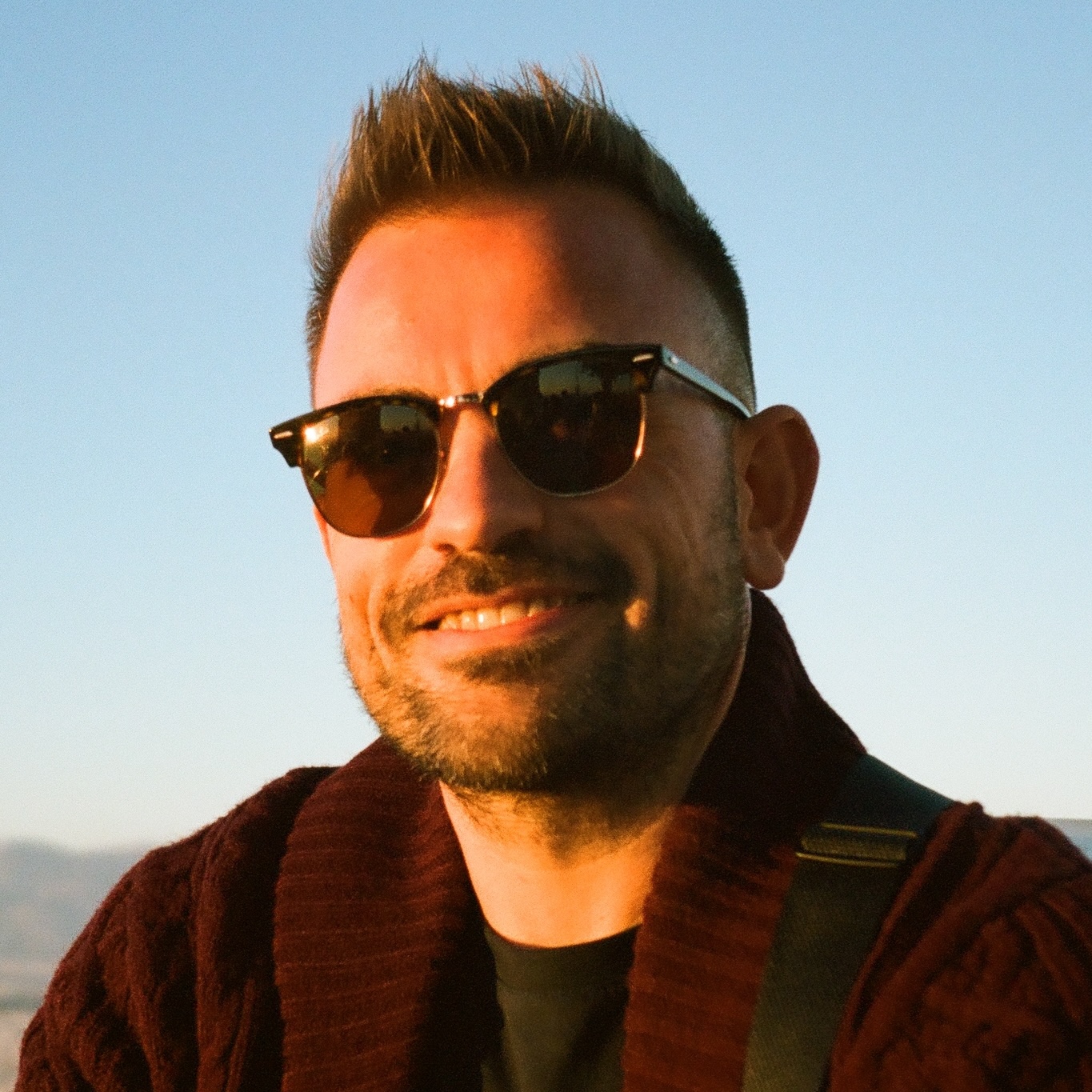
Exporting files to Amazon S3 is a common requirement for modern web applications. Whether you're building a file storage system, implementing backups, or managing user uploads, understanding how to efficiently export files to Amazon S3 using Node.js is essential.
Prerequisites
Before we begin, ensure you have:
- Node.js 18 or later installed
- An AWS account with access credentials
- Basic understanding of JavaScript and Node.js
- A project set up with
"type": "module"
in yourpackage.json
Setting up AWS credentials
To interact with Amazon S3 from your Node.js application, you'll need to configure your AWS credentials. There are several ways to do this:
-
Environment Variables: Set the following environment variables in your system or
.env
file:AWS_ACCESS_KEY_ID=YOUR_ACCESS_KEY_ID AWS_SECRET_ACCESS_KEY=YOUR_SECRET_ACCESS_KEY AWS_REGION=YOUR_AWS_REGION
-
Shared Credentials File: Use the AWS credentials file located at
~/.aws/credentials
:[default] aws_access_key_id = YOUR_ACCESS_KEY_ID aws_secret_access_key = YOUR_SECRET_ACCESS_KEY
-
IAM Roles: If you're running your application on AWS services like EC2, you can assign an IAM role to your instance, and the AWS SDK will automatically use those credentials.
Installing and using the AWS SDK
We'll start with the official AWS SDK for JavaScript (v3):
npm install @aws-sdk/client-s3 @aws-sdk/lib-storage
Here's a basic example of uploading a file to Amazon S3:
import { S3Client, PutObjectCommand } from '@aws-sdk/client-s3'
import { createReadStream } from 'fs'
const s3Client = new S3Client({ region: 'us-east-1' })
async function uploadFile(filePath, bucketName, key) {
try {
const uploadParams = {
Bucket: bucketName,
Key: key,
Body: createReadStream(filePath),
}
const command = new PutObjectCommand(uploadParams)
await s3Client.send(command)
console.log('Upload completed successfully')
} catch (err) {
console.error('Upload failed:', err)
}
}
// Usage
await uploadFile('path/to/file.txt', 'my-bucket', 'file.txt')
This example uses the PutObjectCommand
to upload a file to S3. The createReadStream
function
streams the file for efficient uploading.
Handling large files with multipart upload
For large files, it's recommended to use multipart uploads to improve reliability and performance. The AWS SDK provides utilities for this.
Using the Upload
class from @aws-sdk/lib-storage
simplifies this process:
import { S3Client } from '@aws-sdk/client-s3'
import { Upload } from '@aws-sdk/lib-storage'
import { createReadStream } from 'fs'
const s3Client = new S3Client({ region: 'us-east-1' })
async function uploadLargeFile(filePath, bucketName, key) {
try {
const upload = new Upload({
client: s3Client,
params: {
Bucket: bucketName,
Key: key,
Body: createReadStream(filePath),
},
})
upload.on('httpUploadProgress', (progress) => {
console.log(`Progress: ${progress.loaded}/${progress.total}`)
})
await upload.done()
console.log('Large file upload completed successfully')
} catch (err) {
console.error('Upload failed:', err)
}
}
// Usage
await uploadLargeFile('path/to/large-file.zip', 'my-bucket', 'large-file.zip')
The Upload
class automatically handles multipart uploads, making it easy to upload large files
without worrying about the underlying complexities.
Alternative open-source tools
While the official AWS SDK is robust and fully featured, there are open-source tools that provide alternative ways to interact with Amazon S3.
Using minio
for s3-compatible storage
If you are working with S3-compatible storage services or prefer an open-source library, you might
consider using minio
.
npm install minio
Example usage:
import { Client } from 'minio'
const minioClient = new Client({
endPoint: 's3.amazonaws.com',
accessKey: 'YOUR_ACCESS_KEY_ID',
secretKey: 'YOUR_SECRET_ACCESS_KEY',
})
async function uploadFile(filePath, bucketName, objectName) {
try {
await minioClient.fPutObject(bucketName, objectName, filePath)
console.log('File uploaded successfully')
} catch (err) {
console.error('Error uploading file:', err)
}
}
// Usage
await uploadFile('path/to/file.txt', 'my-bucket', 'file.txt')
Note on deprecated packages
Some older packages like s3fs
and Knox
were popular in the past for interacting with S3 but are
now outdated and no longer maintained. It's recommended to use the official AWS SDK or
well-maintained open-source alternatives to ensure compatibility and security.
Security best practices
When exporting files to Amazon S3, it's crucial to follow security best practices:
-
Use IAM Roles: Instead of hardcoding access keys, use IAM roles when deploying to AWS services like EC2 or ECS. This eliminates the need to manage long-term credentials.
const s3Client = new S3Client({ region: 'us-east-1', })
The AWS SDK will automatically use the credentials provided by the IAM role.
-
Implement Least Privilege Access: Restrict your IAM user or role permissions to only those necessary for the task.
{ "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Action": ["s3:PutObject"], "Resource": "arn:aws:s3:::your-bucket/*" } ] }
-
Enable Server-Side Encryption: Protect your data at rest by enabling server-side encryption.
const uploadParams = { Bucket: bucketName, Key: key, Body: createReadStream(filePath), ServerSideEncryption: 'AES256', }
-
Use HTTPS: Ensure that all data in transit is encrypted by using HTTPS endpoints. The AWS SDK uses HTTPS by default.
Error handling and retries
Implement robust error handling and retry logic to make your application resilient.
The AWS SDK has built-in retry logic, but you can customize it:
import { S3Client, PutObjectCommand } from '@aws-sdk/client-s3'
const s3Client = new S3Client({
region: 'us-east-1',
maxAttempts: 3, // Number of retry attempts
})
async function uploadFileWithRetry(filePath, bucketName, key) {
try {
const uploadParams = {
Bucket: bucketName,
Key: key,
Body: createReadStream(filePath),
}
const command = new PutObjectCommand(uploadParams)
await s3Client.send(command)
console.log('Upload completed successfully')
} catch (err) {
console.error('Upload failed:', err)
}
}
For additional control, you can implement your own retry logic:
async function uploadFileWithCustomRetry(filePath, bucketName, key, maxRetries = 3) {
let attempt = 0
while (attempt < maxRetries) {
try {
await uploadFile(filePath, bucketName, key)
return
} catch (err) {
attempt++
if (attempt === maxRetries) {
console.error(`Failed to upload after ${maxRetries} attempts.`)
throw err
}
console.log(`Retrying upload (${attempt}/${maxRetries})...`)
}
}
}
Conclusion
Efficiently exporting files to Amazon S3 using Node.js involves understanding how to interact with the AWS SDK and following best practices for security and error handling. By leveraging the AWS SDK and implementing the strategies discussed, you can build robust file export functionality in your Node.js applications.
At Transloadit, we focus on making file processing and infrastructure tasks easier. If you're looking for a solution to handle file uploads and processing without the hassle, feel free to check out our services.