Export files to minio: a complete cURL guide
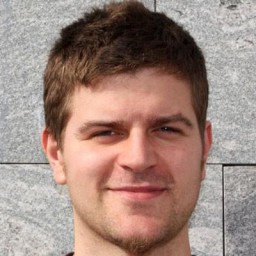
Exporting files to MinIO using cURL provides a flexible and powerful way to manage object storage operations from the command line. MinIO is a high-performance, S3-compatible object storage system, and when combined with cURL—the versatile command-line tool—you can efficiently automate file transfers and streamline your workflows. This guide covers everything you need to know about using cURL with MinIO, from basic uploads to advanced automation techniques.
Introduction to minio and its purpose
MinIO is an open-source, high-performance, distributed object storage system designed for large-scale data infrastructures. It's fully compatible with the Amazon S3 cloud storage service, allowing you to use S3 SDKs and tools to interact with MinIO. This compatibility makes it an excellent choice for building scalable and cost-effective storage solutions.
Overview of cURL for file transfers
cURL is a command-line tool used to transfer data with URLs. It supports various protocols, including HTTP, HTTPS, FTP, and S3. With cURL, you can perform tasks such as downloading files, submitting forms, and interacting with APIs. It's an essential tool for developers and system administrators looking to automate web-related tasks.
Setting up your environment: minio and cURL
Before you start exporting files to MinIO using cURL, ensure your environment is correctly set up.
Prerequisites
-
MinIO Server: Install and run a MinIO server instance. Follow the MinIO Quickstart Guide for installation instructions.
-
Access Credentials: Obtain your MinIO Access Key and Secret Key for authentication.
-
cURL Installed: Verify that cURL is installed by running
curl --version
in your terminal. If it's not installed, you can install it via your package manager. -
Bucket Creation: Create a bucket in MinIO where you will upload your files. You can do this through the MinIO web interface or the
mc
command-line tool.
Securing access to your minio bucket
Security is crucial when transferring files to your MinIO server. Here are some best practices:
-
Use HTTPS: Ensure that all connections to your MinIO server are over HTTPS to encrypt data in transit.
-
Access Keys: Keep your Access Key and Secret Key secure. Avoid hardcoding them in scripts; instead, use environment variables or secure credential storage mechanisms.
-
Bucket Policies: Set appropriate bucket policies to control access permissions.
-
Signature Authentication: Use AWS Signature Version 4 for request authentication to prevent unauthorized access.
Exporting files to minio with cURL: step-by-step guide
Now, let's dive into exporting files to MinIO using cURL.
Understanding the REST API
MinIO implements an S3-compatible REST API, so you can interact with it using standard HTTP requests. This compatibility allows you to use cURL to perform operations like uploading and downloading files.
Basic file upload
Uploading a file to MinIO involves making a PUT request with the appropriate headers and authentication.
Example:
curl -X PUT \
-T "file.txt" \
-H "Host: minio-server:9000" \
-H "Date: $(date -Ru)" \
-H "Content-Type: application/octet-stream" \
-H "Authorization: AWS4-HMAC-SHA256 Credential=ACCESS_KEY/.../aws4_request, SignedHeaders=..., Signature=..." \
"http://minio-server:9000/bucket-name/file.txt"
Generating the authorization header
Manually generating the AWS Signature Version 4 is complex. It's recommended to use tools or scripts that handle the signature generation.
Alternatively, you can use the aws-cli
or MinIO's mc
command-line tool, but since we're focusing
on cURL, here's a method using pre-signed URLs.
Generating pre-signed URLs
Pre-signed URLs allow you to upload files without having to handle authentication headers manually.
Generating a Pre-Signed URL:
Using MinIO's mc
tool:
# Generate a pre-signed put URL valid for 1 hour
mc presign put myminio/mybucket/file.txt --expire=1h
Uploading with cURL:
curl -X PUT \
-T "file.txt" \
"http://minio-server:9000/mybucket/file.txt?X-Amz-Algorithm=AWS4-HMAC-SHA256&..."
Downloading files using pre-signed URLs
Similarly, you can generate pre-signed URLs for downloading files.
# Generate a pre-signed get URL
mc presign get myminio/mybucket/file.txt --expire=1h
# Use cURL to download the file
curl -O "http://minio-server:9000/mybucket/file.txt?X-Amz-Algorithm=AWS4-HMAC-SHA256&..."
Automating exports with shell scripts
Automate the file export process using shell scripts to handle multiple files and directories.
Example Script:
#!/bin/bash
MINIO_HOST="http://minio-server:9000"
BUCKET="mybucket"
ACCESS_KEY="your-access-key"
SECRET_KEY="your-secret-key"
EXPIRATION="1h"
# Function to generate pre-signed URL for uploading
generate_presigned_url() {
local file_name="$1"
mc presign put "myminio/${BUCKET}/${file_name}" --expire=${EXPIRATION}
}
# Function to upload file using cURL and pre-signed URL
upload_file() {
local file_path="$1"
local file_name=$(basename "$file_path")
local presigned_url=$(generate_presigned_url "$file_name")
curl -X PUT -T "$file_path" "$presigned_url"
}
# Upload all files in the current directory
for file in *; do
if [ -f "$file" ]; then
echo "Uploading $file..."
upload_file "$file"
fi
done
Note: This script uses mc
to generate pre-signed URLs and cURL to upload files, combining both
tools for ease of use.
Advanced techniques: handling complex file structures
When dealing with large files or complex directory structures, consider the following techniques.
Working with large files
For large files, you can use multipart uploads to ensure reliable transfers.
Note: Manually handling multipart uploads with cURL is complex due to the need for multiple requests and signature calculations. It's recommended to use SDKs or tools that support multipart uploads out of the box.
Uploading directories
To upload an entire directory, you can modify the script to recursively traverse directories.
Example:
#!/bin/bash
# ... [previous variable definitions]
upload_directory() {
local dir_path="$1"
find "$dir_path" -type f | while read -r file; do
relative_path="${file#$dir_path/}"
presigned_url=$(mc presign put "myminio/${BUCKET}/${relative_path}" --expire=${EXPIRATION})
curl -X PUT -T "$file" "$presigned_url"
done
}
# Upload the specified directory
upload_directory "/path/to/directory"
Error handling and monitoring
Implement robust error handling and monitor your uploads to ensure data integrity.
Error handling in scripts
Modify your scripts to handle errors and retry failed uploads.
Example:
upload_file() {
local file_path="$1"
local retries=3
for ((i=1; i<=retries; i++)); do
if curl -X PUT -T "$file_path" "$presigned_url"; then
echo "Upload succeeded for $file_path"
return 0
else
echo "Upload failed for $file_path. Retrying ($i/$retries)..."
sleep $((i * 2))
fi
done
echo "Failed to upload $file_path after $retries attempts."
return 1
}
Monitoring upload progress
Use cURL's progress bar to monitor the upload progress of large files.
curl -X PUT \
-T "large-file.zip" \
--progress-bar \
"http://minio-server:9000/mybucket/large-file.zip?X-Amz-Algorithm=AWS4-HMAC-SHA256&..."
Troubleshooting common issues and solutions
Common issues
-
Authentication Errors: Ensure your access keys are correct and that the signature is properly generated.
-
Connection Timeouts: Check network connectivity and MinIO server status.
-
Access Denied: Verify bucket policies and permissions.
Solutions
-
Verbose Output: Use
curl -v
to get detailed logs of the request and response. -
Check Certificates: If using HTTPS, ensure SSL certificates are correctly installed and trusted.
-
Review Logs: Check MinIO server logs for any server-side errors.
Using Transloadit for optimized minio exports
If you're looking for a more efficient and robust solution for exporting files to MinIO, consider using Transloadit. Transloadit offers powerful file processing and exporting capabilities, making it easier to handle complex workflows without dealing with low-level details.
Conclusion
Exporting files to MinIO using cURL empowers you to automate and streamline your object storage operations directly from the command line. By leveraging the techniques and scripts provided in this guide, you can efficiently manage your file exports, handle large datasets, and integrate MinIO into your automation pipelines.
For more advanced file processing needs, Transloadit's services can further simplify and optimize your workflows.