Export files to YouTube in .Net C# using open source libraries
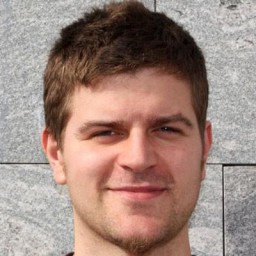
Exporting videos to YouTube programmatically is essential for applications that handle video content, such as media management systems, content creators' tools, or automated services. While YouTube provides official .NET client libraries for its Data API, there are scenarios where you might prefer to interact directly with the REST API using open-source libraries for greater control or flexibility.
In this guide, we'll explore how to export files to YouTube in .NET C# using open-source libraries and direct REST API integration. We'll cover setting up authentication, uploading videos, handling large files, implementing error handling, and monitoring upload progress.
Prerequisites
- .NET 6 SDK or later
- A Google Cloud Project with YouTube Data API enabled
- OAuth 2.0 credentials from the Google Cloud Console
- Basic knowledge of C#, REST APIs, and HTTP communication
Setting up authentication
To interact with the YouTube Data API, you'll need to authenticate your application using OAuth 2.0.
Since we're aiming to use open-source libraries and direct REST API calls, we'll utilize libraries
like IdentityModel
for handling OAuth 2.0 flows.
First, install the required NuGet packages:
dotnet add package IdentityModel
dotnet add package Newtonsoft.Json
Create a method to obtain an access token using your client credentials:
using IdentityModel.Client;
// ...
public async Task<string> GetAccessTokenAsync(string clientId, string clientSecret, string[] scopes)
{
var client = new HttpClient();
var discovery = await client.GetDiscoveryDocumentAsync("https://accounts.google.com");
if (discovery.IsError)
{
throw new Exception(discovery.Error);
}
var tokenResponse = await client.RequestClientCredentialsTokenAsync(new ClientCredentialsTokenRequest
{
Address = discovery.TokenEndpoint,
ClientId = clientId,
ClientSecret = clientSecret,
Scope = string.Join(" ", scopes)
});
if (tokenResponse.IsError)
{
throw new Exception(tokenResponse.Error);
}
return tokenResponse.AccessToken;
}
Note: For YouTube Data API, you'll typically need to use the OAuth 2.0 Authorization Code flow for user-delegated access rather than client credentials grant. Implementing this flow involves redirecting the user to Google's authorization endpoint and handling the authorization code returned.
Uploading videos with REST API
Once you have an access token, you can use HttpClient
to make REST API calls to the YouTube Data
API.
using System.Net.Http.Headers;
// ...
public async Task<string> UploadVideoAsync(string accessToken, string filePath, string title, string description, string[] tags)
{
var client = new HttpClient();
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
// Create the video metadata
var videoMetadata = new
{
snippet = new
{
title = title,
description = description,
tags = tags,
categoryId = "22" // People & Blogs category
},
status = new
{
privacyStatus = "private" // or "public", "unlisted"
}
};
var serializedMetadata = Newtonsoft.Json.JsonConvert.SerializeObject(videoMetadata);
var requestContent = new MultipartFormDataContent();
var metadataContent = new StringContent(serializedMetadata);
metadataContent.Headers.ContentType = new MediaTypeHeaderValue("application/json; charset=UTF-8");
var fileStream = new FileStream(filePath, FileMode.Open);
var fileContent = new StreamContent(fileStream);
fileContent.Headers.ContentType = new MediaTypeHeaderValue("video/*");
requestContent.Add(metadataContent, "snippet");
requestContent.Add(fileContent, "video", Path.GetFileName(filePath));
var requestUri = "https://www.googleapis.com/upload/youtube/v3/videos?uploadType=multipart&part=snippet,status";
var response = await client.PostAsync(requestUri, requestContent);
if (!response.IsSuccessStatusCode)
{
var errorContent = await response.Content.ReadAsStringAsync();
throw new Exception($"Failed to upload video: {response.ReasonPhrase} - {errorContent}");
}
var responseContent = await response.Content.ReadAsStringAsync();
dynamic responseData = Newtonsoft.Json.JsonConvert.DeserializeObject(responseContent);
return responseData.id;
}
Note: This example uses a multipart upload, which is suitable for small files. For larger files, you should use resumable uploads.
Handling large files with resumable uploads
For large video files, implement resumable uploads to handle interruptions and improve reliability. The YouTube Data API supports resumable uploads using the resumable upload protocol.
Implementing resumable uploads involves:
- Initiating a resumable upload session.
- Uploading the video file in chunks.
- Handling interruptions by resuming the upload from the last byte received by the server.
This process can be complex to implement manually. You might use open-source libraries that handle
the resumable upload protocol, such as Google.Apis.ResumableUpload
.
Error handling best practices
Implement comprehensive error handling to manage API errors, rate limits, and quota issues.
public async Task<string> UploadVideoWithErrorHandlingAsync(string accessToken, string filePath, string title, string description, string[] tags)
{
try
{
return await UploadVideoAsync(accessToken, filePath, title, description, tags);
}
catch (HttpRequestException ex)
{
Console.WriteLine($"HTTP Request error: {ex.Message}");
// Handle network errors
throw;
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
// Handle other exceptions
throw;
}
}
Check the error response details and handle specific error codes as needed. For instance, if you receive a 403 error due to quota limits, you might implement a delay before retrying or adjust your application's usage patterns.
Monitoring upload progress
To improve user experience, monitor the upload progress of your videos.
public async Task<string> UploadVideoWithProgressAsync(string accessToken, string filePath, string title, string description, string[] tags, IProgress<double> progress)
{
var client = new HttpClient();
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
// ... Set up request as before ...
var fileStream = new FileStream(filePath, FileMode.Open, FileAccess.Read);
var totalBytes = fileStream.Length;
var uploadedBytes = 0L;
var buffer = new byte[8192];
int bytesRead;
// Create the content
var content = new StreamContent(fileStream);
content.Headers.ContentType = new MediaTypeHeaderValue("video/*");
// Send the request
var requestUri = "https://www.googleapis.com/upload/youtube/v3/videos?uploadType=multipart&part=snippet,status";
var requestMessage = new HttpRequestMessage(HttpMethod.Post, requestUri)
{
Content = new MultipartFormDataContent
{
{ new StringContent(serializedMetadata, Encoding.UTF8, "application/json"), "snippet" },
{ content, "video", Path.GetFileName(filePath) }
}
};
var response = await client.SendAsync(requestMessage, HttpCompletionOption.ResponseHeadersRead);
// Optionally, read the response and report progress (advanced implementation required)
// Handle response as before...
return responseData.id;
}
Implementing progress reporting with HttpClient
requires custom handlers or third-party libraries
that support progress events during HTTP content uploads.
Conclusion
Exporting files to YouTube in .NET C# using open-source libraries and direct REST API integration provides you with full control over the upload process. While it requires more effort than using the official client libraries, it allows for customization and a deeper understanding of the YouTube Data API.
For a streamlined approach to handling file exports and video processing at scale, consider using Transloadit's file exporting service. Transloadit can automate uploads to YouTube and other platforms, simplifying your workflow and reducing the overhead of managing file uploads manually.