Exporting files to Dropbox in Ruby using the Dropbox SDK
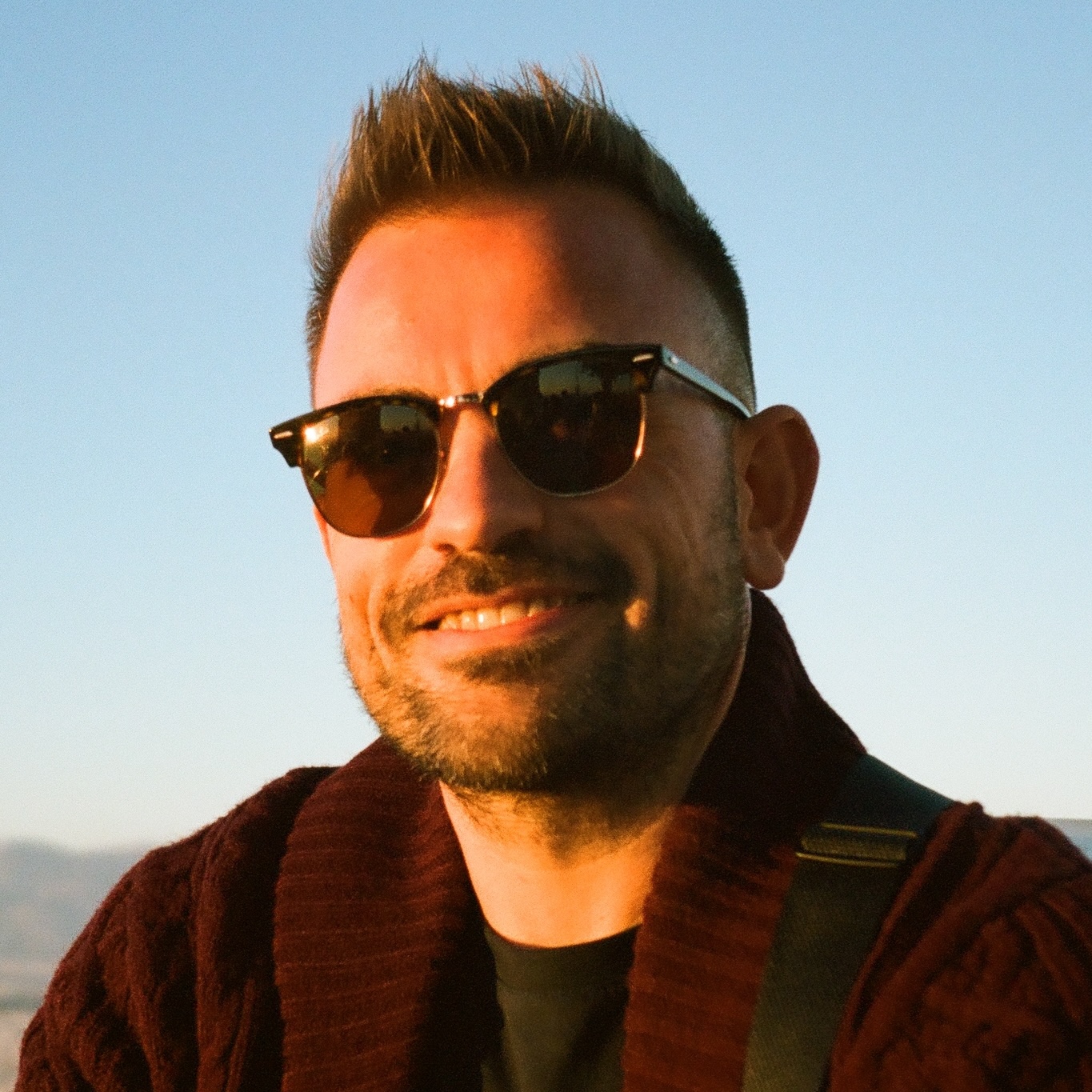
Integrating Dropbox file exports into your Ruby applications can streamline your workflow and enhance functionality. This guide walks you through using the Dropbox SDK for Ruby to upload files programmatically, providing practical examples to help you automate file uploads to Dropbox.
Prerequisites
Before you begin, ensure you have:
- Ruby 2.7 or higher installed on your machine
- A Dropbox account
- Basic knowledge of Ruby programming
Setting up a Dropbox app and obtaining access tokens
To interact with the Dropbox API, you need to create a Dropbox app and generate an access token:
-
Create a Dropbox App:
- Log in to the Dropbox App Console
- Click on "Create App"
- Choose "Scoped access" and select "Full Dropbox" or "App folder", depending on your needs
- Give your app a unique name and click "Create App"
-
Generate an Access Token:
- In your app's settings, navigate to the "OAuth 2" section
- Under "Generated access token", click "Generate"
- Copy the generated access token; you'll need it in your application
Installing the Dropbox SDK for Ruby
Install the Dropbox SDK for Ruby using the gem
command:
gem install dropbox_api
Authenticating with Dropbox in Ruby
Begin by requiring the Dropbox SDK and setting up authentication:
require 'dropbox_api'
# Replace with your access token
ACCESS_TOKEN = 'YOUR_ACCESS_TOKEN'
# Create a Dropbox client using the access token
dbx = DropboxApi::Client.new(ACCESS_TOKEN)
Uploading files to Dropbox using Ruby
Define a method to upload a file to Dropbox:
def upload_file(dbx, file_path, dropbox_path)
File.open(file_path, 'rb') do |file|
dbx.upload(dropbox_path, file)
puts "File '#{file_path}' uploaded to '#{dropbox_path}'"
end
rescue DropboxApi::Errors::HttpError => e
puts "Failed to upload '#{file_path}' to '#{dropbox_path}': #{e.message}"
end
Usage example:
# Local file path
local_file = 'path/to/your/local/file.txt'
# Dropbox destination path
dropbox_destination = '/Apps/YourAppFolder/file.txt'
# Upload the file
upload_file(dbx, local_file, dropbox_destination)
Handling errors and exceptions
Proper error handling is crucial when interacting with the Dropbox API. Enhance the upload_file
method to handle different exceptions:
def upload_file(dbx, file_path, dropbox_path)
File.open(file_path, 'rb') do |file|
dbx.upload(dropbox_path, file)
puts "File '#{file_path}' uploaded to '#{dropbox_path}'"
end
rescue DropboxApi::Errors::AuthError => e
puts "Authentication error: #{e.message}"
rescue DropboxApi::Errors::HttpError => e
puts "API error: #{e.message}"
rescue StandardError => e
puts "An error occurred: #{e.message}"
end
Automating file exports in your Ruby application
Automate file uploads by integrating the upload function into your application's workflow. For
example, use the rufus-scheduler
gem to upload files at regular intervals:
First, install the rufus-scheduler
gem:
gem install rufus-scheduler
Then, use it in your application:
require 'rufus-scheduler'
scheduler = Rufus::Scheduler.new
# Ensure dbx is accessible within the Job
dbx = DropboxApi::Client.new(ACCESS_TOKEN)
# Define the Job to run
def upload_job
local_file = 'path/to/your/local/file.txt'
dropbox_destination = '/Apps/YourAppFolder/file.txt'
upload_file(dbx, local_file, dropbox_destination)
end
# Schedule the Job to run every hour
scheduler.every '1h' do
upload_job
end
scheduler.join
Conclusion
By integrating the Dropbox SDK into your Ruby applications, you can automate file exports and streamline your workflow. This guide provided a step-by-step approach to authenticating with the Dropbox API, uploading files, handling errors, and automating the process. With these tools, you're well on your way to enhancing your application's functionality.
At Transloadit, we understand the importance of efficient file handling. If you're looking for a robust solution to manage file uploads and processing, check out our File Exporting services.