Extract audio on iOS & macOS with FFmpeg
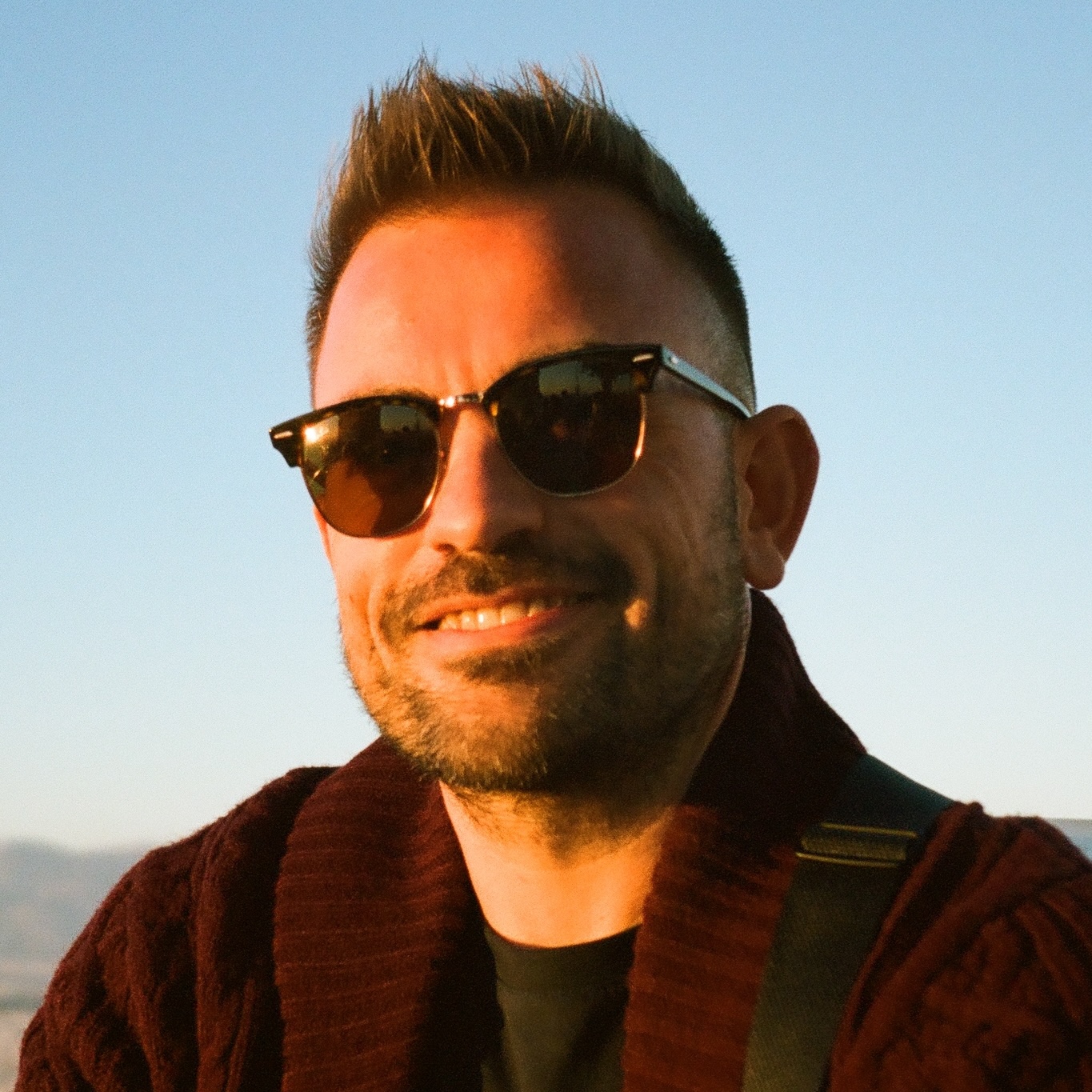
FFmpeg is a versatile multimedia framework widely used for audio and video processing. On Apple platforms like iOS and macOS, FFmpeg enhances multimedia app development by enabling efficient audio extraction from video files. In this DevTip, we'll explore how to set up and use FFmpegKit—a popular FFmpeg wrapper—to extract audio streams in your Swift applications.
Introduction to FFmpeg on apple platforms
FFmpeg is an open-source multimedia processing tool capable of handling various audio and video formats. It supports encoding, decoding, transcoding, and stream extraction. FFmpegKit simplifies integrating FFmpeg's capabilities into your Swift projects on iOS and macOS.
Setting up FFmpegKit on iOS and macOS
We'll use FFmpegKit, a wrapper around FFmpeg, designed for mobile and desktop platforms.
Install via Swift package manager
In Xcode:
- Open your project and navigate to
File > Add Packages
. - Enter the repository URL:
https://github.com/arthenica/ffmpeg-kit.git
. - Select the latest stable version and add it to your project.
Extracting audio streams from video files
Let's explore a practical example of extracting audio from a video file using FFmpegKit in Swift.
Step-by-step guide for audio extraction
Here's a Swift example demonstrating audio extraction:
import FFmpegKit
func extractAudio(from videoURL: URL, outputURL: URL, completion: @escaping (Bool, String?) -> Void) {
let command = "-i \"\(videoURL.path)\" -vn -acodec copy \"\(outputURL.path)\""
FFmpegKit.executeAsync(command) { session in
guard let returnCode = session?.getReturnCode() else {
completion(false, "No return code available")
return
}
if returnCode.isValueSuccess() {
completion(true, nil)
} else {
let failStackTrace = session?.getFailStackTrace()
completion(false, failStackTrace)
}
}
}
Usage example
let videoURL = Bundle.main.url(forResource: "sample-video", withExtension: "mp4")!
let outputURL = FileManager.default.temporaryDirectory.appendingPathComponent("extracted-audio.m4a")
extractAudio(from: videoURL, outputURL: outputURL) { success, error in
if success {
print("Audio extraction successful: \(outputURL.path)")
} else {
print("Audio extraction failed: \(error ?? "Unknown error")")
}
}
Handling common issues
Here are common issues and how to resolve them:
- Permission Issues: Ensure your app has the necessary permissions to read and write files.
- Unsupported Formats: Verify the input video format and audio codec compatibility.
- Debugging FFmpeg Commands: Use
session?.getOutput()
to inspect detailed FFmpeg logs.
Optimizing performance
To optimize performance:
- Use hardware acceleration when available (
-hwaccel videotoolbox
). - Avoid unnecessary transcoding by copying audio streams directly (
-acodec copy
). - Manage memory efficiently by processing large files asynchronously.
Additional resources
Integrating FFmpegKit into your iOS and macOS apps provides powerful multimedia processing capabilities. For further exploration, check out the FFmpegKit documentation.
At Transloadit, we leverage FFmpeg in our Audio Encoding and Video Encoding services, powering Robots like /audio/encode and /video/encode.