Troubleshooting file upload failures: practical solutions
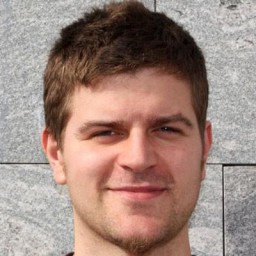
File uploads are a fundamental part of many web applications, yet they can often be a source of frustration when things don't go as planned. Understanding the common reasons behind file upload failures and knowing how to diagnose and resolve these issues quickly can save significant time and headaches.
Common reasons for file upload errors
File upload failures typically stem from a few common issues:
- File size limitations: Servers often impose limits on the maximum file size allowed.
- Network connectivity: Unstable or slow connections can interrupt uploads.
- File format compatibility: Servers may reject unsupported file types.
- Server storage issues: Insufficient disk space or quota limits can halt uploads.
- Server misconfigurations: Incorrect server settings can prevent successful uploads.
Diagnosing file upload issues using tools
Effective diagnosis starts with the right tools:
- Browser Developer Tools: Inspect network requests to identify HTTP status codes and error messages.
- Server Logs: Check logs for detailed error messages related to file uploads.
- Monitoring Tools: Use tools like Prometheus or Grafana to monitor server resources and detect storage or memory issues.
Security considerations for file uploads
File uploads present significant security risks if not properly handled. Follow these security best practices:
- Validate file extensions: Maintain a whitelist of allowed extensions rather than a blacklist.
- Verify file content: Use content validation libraries to verify the file type.
- Rename uploaded files: Generate random filenames to prevent directory traversal attacks.
- Store files outside the webroot: Store uploaded files in a location not directly accessible via the web server.
- Scan for malware: Implement virus scanning for uploaded files.
- Implement Content Disarm & Reconstruction (CDR): Neutralize potentially malicious content in documents.
Preventive measures to avoid file upload errors
Implementing preventive measures can significantly reduce upload issues:
- Clearly document and communicate file size and format restrictions to users.
- Implement client-side validation to catch issues before files reach the server.
- Regularly monitor and manage server storage capacity.
- Configure servers correctly, ensuring proper permissions and settings.
Step-by-step solutions to fix common upload errors
File size limitations
Adjust server-side settings (e.g., upload_max_filesize
and post_max_size
in PHP). Implement
chunked uploads to handle large files efficiently.
Implementing chunked uploads
Here's a basic JavaScript example for chunked uploads:
const fileInput = document.getElementById('file-upload')
fileInput.addEventListener('change', handleFileUpload)
function handleFileUpload(event) {
const file = event.target.files[0]
const chunkSize = 1024 * 1024 // 1MB chunks
let start = 0
while (start < file.size) {
uploadChunk(file.slice(start, start + chunkSize), start, file.name)
start += chunkSize
}
}
async function uploadChunk(chunk, start, fileName) {
const formData = new FormData()
formData.append('file', chunk)
formData.append('start', start)
formData.append('fileName', fileName)
await fetch('/upload-chunk', { method: 'POST', body: formData })
}
Network connectivity issues
Implement resumable uploads using protocols like tus.io.
Implementing resumable uploads with tus.io
Install tus-js-client:
npm install tus-js-client
Basic implementation:
import * as tus from 'tus-js-client'
const upload = new tus.Upload(file, { endpoint: 'https://your-tus-server.com/files/' })
upload.start()
File format compatibility
Clearly specify supported formats and validate file types on both client and server sides.
Server storage issues
Regularly monitor disk usage and automate cleanup of temporary files.
Server misconfigurations
Regularly audit server configurations and use tools like Ansible or Terraform.
Conclusion and additional resources
Understanding and addressing file upload failures proactively enhances reliability and user experience. For further reading, explore MDN Web Docs and tus.io.
For robust file upload handling, consider Transloadit's Upload Handling Service.