How to crop images and videos with FFmpeg
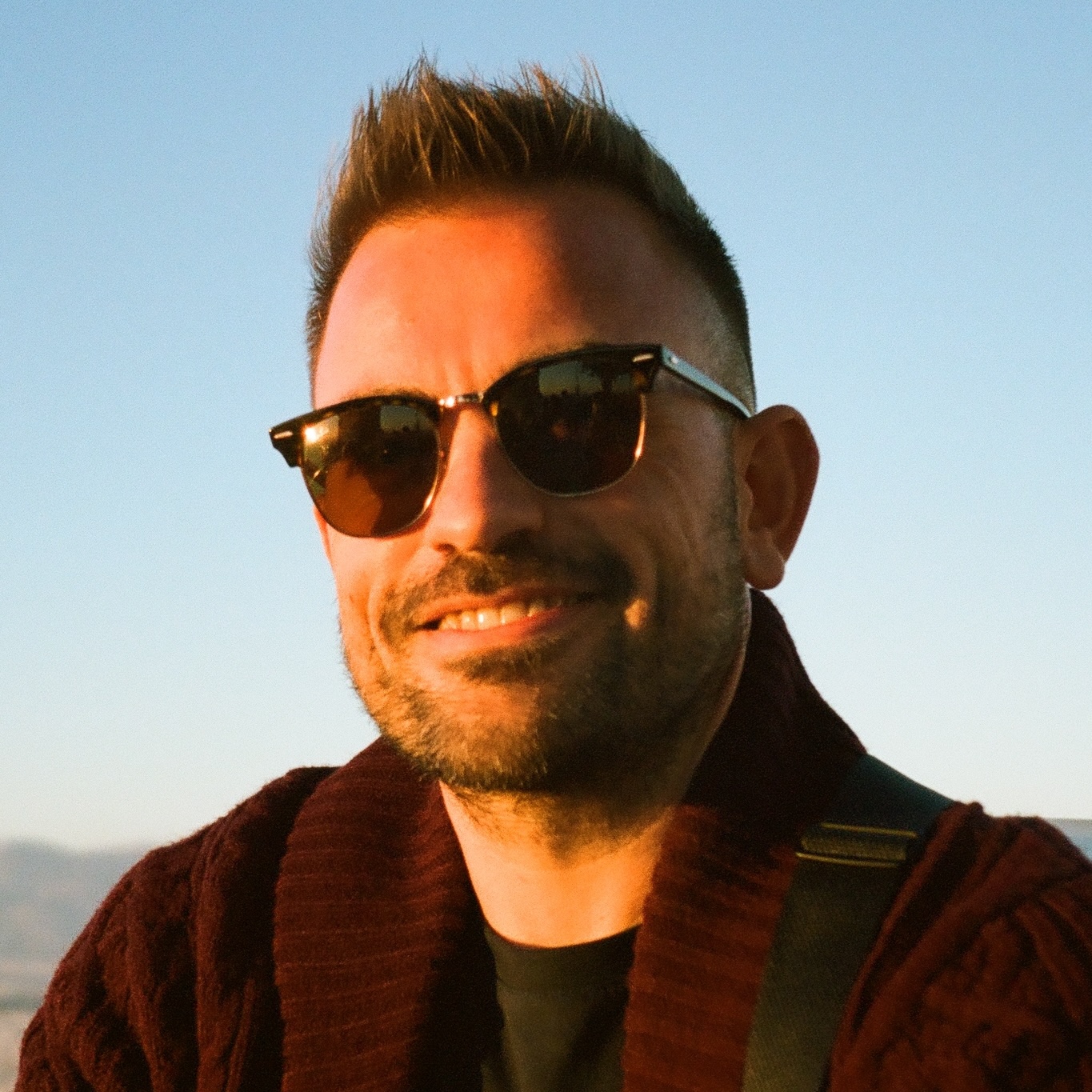
FFmpeg is a powerful command-line tool that enables efficient image and video cropping. This guide explores how to use FFmpeg to crop media files directly from the command line, streamlining your development workflow.
Introduction to FFmpeg for image and video cropping
FFmpeg is a versatile command-line utility used for processing audio and video files. It's widely used for converting between formats, recording, encoding, and streaming. One of its key features is the ability to crop images and videos, making it invaluable for developers who need to manipulate media files programmatically.
Installing FFmpeg on your system
Before we begin, you need to have FFmpeg installed on your system.
Installing on macOS
You can install FFmpeg on macOS using Homebrew:
brew install ffmpeg
Installing on Linux
For Debian-based systems:
sudo apt update
sudo apt install ffmpeg
For Red Hat-based systems:
sudo dnf install ffmpeg
Installing on Windows
Download the latest FFmpeg build from the official website.
Extract the binaries and add the FFmpeg bin
directory to your system's PATH environment variable.
Basic concepts of cropping in FFmpeg
Cropping in FFmpeg is accomplished using the crop
filter, which requires four parameters:
width
(out_w
): The width of the output video or image.height
(out_h
): The height of the output video or image.x
: The x-coordinate of the top-left corner from where to start cropping.y
: The y-coordinate of the top-left corner from where to start cropping.
The general syntax for cropping is:
ffmpeg -i input -vf "crop=out_w:out_h:x:y" output
Cropping images with FFmpeg
FFmpeg can be used to crop images as well as videos. Here's how you can crop an image using FFmpeg.
Example: cropping an image
Suppose you have an image input.jpg
and you want to crop a 200x200 pixel region starting from
coordinates (50,50):
ffmpeg -i input.jpg -vf "crop=200:200:50:50" output.jpg
Explanation
-i input.jpg
: Specifies the input file.-vf "crop=200:200:50:50"
: Applies the crop video filter with the specified parameters.output.jpg
: The name of the output file.
Cropping videos with FFmpeg
Cropping videos works similarly to cropping images. FFmpeg applies the crop filter to every frame of the video.
Example: cropping a video
Suppose you have a video input.mp4
and you want to crop a 1280x720 region starting from
coordinates (0,0):
ffmpeg -i input.mp4 -vf "crop=1280:720:0:0" output.mp4
Explanation
-i input.mp4
: Specifies the input video file.-vf "crop=1280:720:0:0"
: Crops the video to 1280x720 starting from the top-left corner.output.mp4
: The name of the output video file.
Dynamic cropping based on input dimensions
You can also use expressions to crop relative to the input video's dimensions.
For example, to crop the central square of a video:
ffmpeg -i input.mp4 -vf "crop='min(in_w\,in_h)':'min(in_w\,in_h)':(in_w - min(in_w\,in_h))/2:(in_h - min(in_w\,in_h))/2" output.mp4
in_w
andin_h
represent the input video's width and height.- The
min(in_w\,in_h)
function calculates the smallest dimension of the input video. - The crop's
out_w
andout_h
are set tomin(in_w\,in_h)
to create a square crop. (in_w - out_w)/2
and(in_h - out_h)/2
center the crop horizontally and vertically.
Command line options for cropping in FFmpeg
The key parameters for cropping are specified in the crop
filter. Here's a breakdown:
crop=out_w:out_h:x:y
Alternatively, you can use named parameters:
ffmpeg -i input.mp4 -vf "crop=w=1280:h=720:x=0:y=0" output.mp4
This is functionally the same but may be clearer to read.
Automating cropping tasks with scripts
You can automate cropping tasks by writing shell scripts or batch files.
Example: batch cropping images
Suppose you have multiple images in a directory that you want to crop identically.
Bash script (linux/macos)
#!/bin/bash
for img in *.jpg; do
ffmpeg -i "$img" -vf "crop=200:200:50:50" "cropped_$img"
done
Batch script (Windows)
for %%i in (*.jpg) do ffmpeg -i "%%i" -vf "crop=200:200:50:50" "cropped_%%i"
These scripts loop over all .jpg
files in the current directory and apply the same crop to each,
saving the output with a cropped_
prefix.
Using FFmpeg in other languages
You can also invoke FFmpeg commands from within programming languages like Python.
Python example
import subprocess
import glob
for img in glob.glob("*.jpg"):
subprocess.call([
"ffmpeg",
"-i", img,
"-vf", "crop=200:200:50:50",
f"cropped_{img}"
])
Why use FFmpeg for image cropping?
FFmpeg is a powerful and flexible tool that can handle a wide variety of media processing tasks. Some reasons to use FFmpeg for image cropping include:
- Automation: Easily scriptable for batch processing.
- Versatility: Supports many input and output formats.
- Efficiency: Processes media quickly and integrates well into larger processing pipelines.
- Command Line: Suitable for servers and headless environments; no GUI needed.
Conclusion and further resources
Cropping images and videos using FFmpeg is straightforward once you understand the basic parameters. Whether you're working on a one-time task or need to automate media processing in your application, FFmpeg offers a robust set of tools to get the job done efficiently.
For more information, check out the FFmpeg documentation.
If you're looking for a scalable solution to automate media processing tasks, including image and video cropping, Transloadit offers a powerful API that can help streamline your workflow.