Java PDF processing with Ghost4J & Ghostscript
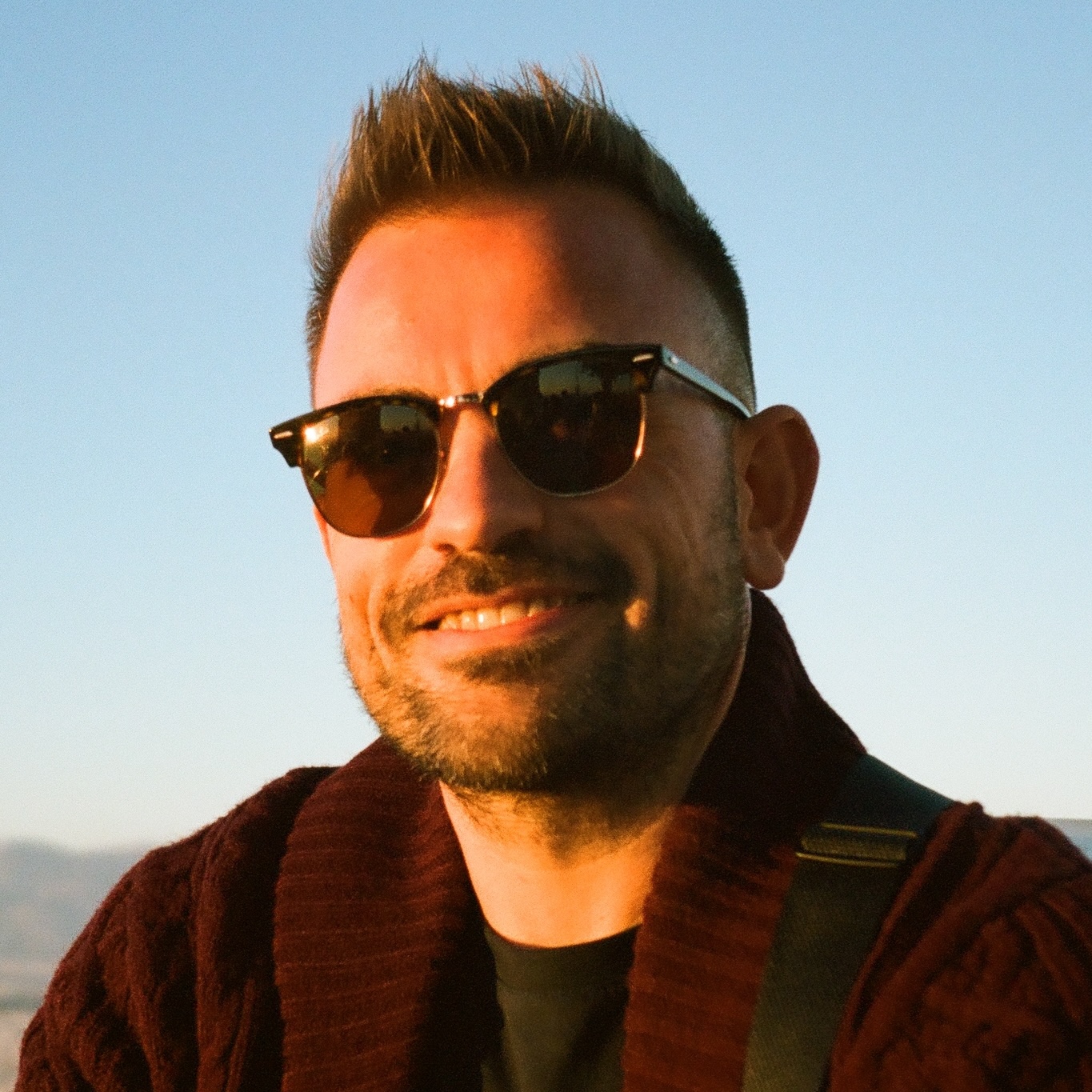
Ghostscript is a powerful open-source interpreter for PostScript and PDF files. It's widely used for tasks like PDF rendering, conversion, and analysis. Ghost4J is a Java wrapper around Ghostscript that simplifies integrating these capabilities into Java applications. This DevTip explores how to use Ghost4J to streamline your PDF processing workflows.
Introduction to Ghostscript
Ghostscript is a versatile tool capable of:
- Converting PDFs to images
- Rendering PDFs for viewing
- Analyzing PDF content, including fonts and metadata
- Optimizing and compressing PDF files
Interacting directly with Ghostscript from Java can be cumbersome. Ghost4J bridges this gap by providing a clean Java API.
What is Ghost4J?
Ghost4J is an open-source Java library that wraps Ghostscript. It enables Java developers to easily leverage Ghostscript's powerful PDF processing capabilities. It simplifies tasks such as:
- PDF to image conversion
- Concurrent PDF processing
- Metadata analysis
Set up Ghost4J in your Java project
First, add Ghost4J to your Maven project:
<dependency>
<groupId>org.ghost4j</groupId>
<artifactId>ghost4j</artifactId>
<version>1.0.1</version>
</dependency>
Ensure Ghostscript is installed on your system and accessible via your system's PATH. Install Ghostscript using package managers:
- On Ubuntu/Debian:
sudo apt-get install ghostscript
- On macOS (with Homebrew):
brew install ghostscript
Convert PDF to image
Here's how to convert a PDF to images using Ghost4J:
import org.ghost4j.document.PDFDocument;
import org.ghost4j.renderer.SimpleRenderer;
import java.awt.image.BufferedImage;
import javax.imageio.ImageIO;
import java.io.File;
import java.util.List;
public class PDFToImage {
public static void main(String[] args) throws Exception {
PDFDocument document = new PDFDocument();
document.load(new File("input.pdf"));
SimpleRenderer renderer = new SimpleRenderer();
renderer.setResolution(300);
List<BufferedImage> images = renderer.render(document);
for (int i = 0; i < images.size(); i++) {
ImageIO.write(images.get(i), "png", new File("output_" + (i + 1) + ".png"));
}
}
}
This snippet loads a PDF, renders each page at 300 DPI, and saves each page as a separate PNG image.
Concurrent PDF processing
Ghost4J supports concurrent processing, which significantly speeds up batch operations:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import org.ghost4j.document.PDFDocument;
import org.ghost4j.renderer.SimpleRenderer;
import java.awt.image.BufferedImage;
import javax.imageio.ImageIO;
import java.io.File;
import java.util.List;
public class ConcurrentPDFProcessing {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(4);
File[] pdfFiles = new File(".").listFiles(f -> f.getName().endsWith(".pdf")); // Example: list all PDFs in current directory
for (File pdfFile : pdfFiles) {
executor.submit(() -> {
try {
PDFDocument document = new PDFDocument();
document.load(pdfFile);
SimpleRenderer renderer = new SimpleRenderer();
renderer.setResolution(200);
List<BufferedImage> images = renderer.render(document);
for (int i = 0; i < images.size(); i++) {
ImageIO.write(images.get(i), "jpg", new File(pdfFile.getName() + "_" + (i + 1) + ".jpg"));
}
} catch (Exception e) {
e.printStackTrace();
}
});
}
executor.shutdown();
}
}
This example processes multiple PDFs concurrently, leveraging Java's ExecutorService
for parallel
execution.
Analyze fonts in PDF documents
For font analysis, Apache PDFBox is a reliable alternative to Ghost4j:
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.text.PDFTextStripper;
import org.apache.pdfbox.pdmodel.font.PDFont;
import java.io.File;
public class FontAnalysis {
public static void main(String[] args) throws Exception {
PDDocument document = PDDocument.load(new File("input.pdf"));
PDFTextStripper stripper = new PDFTextStripper();
stripper.getText(document); // Needed to properly initialize fonts
for (PDFont font : document.getDocumentCatalog().getPages().get(0).getResources().getFontNames()) {
System.out.println(font.getName());
}
document.close();
}
}
This snippet lists all fonts used in a PDF, which is useful for compliance checks or optimization.
Note the use of stripper.getText(document);
. While seemingly unrelated, this line is crucial. It
forces PDFBox to process the text content of the PDF, which, in turn, initializes the font
information that is later accessed. Without this line, the font information might not be fully
loaded, leading to incomplete or incorrect results.
Performance considerations and best practices
- Resolution: Higher resolutions improve quality but increase processing time and memory usage.
- Concurrency: Balance the thread count with available CPU cores to avoid performance degradation.
- Resource Management: Always close streams and dispose of resources properly to prevent memory leaks.
Conclusion
Ghost4J simplifies PDF processing in Java, offering robust capabilities for conversion, rendering, and analysis. For further exploration, check out:
If you're looking for a managed solution to handle document processing at scale, consider exploring Transloadit's Document Processing Service.