Looping audio in Python: techniques for seamless playback
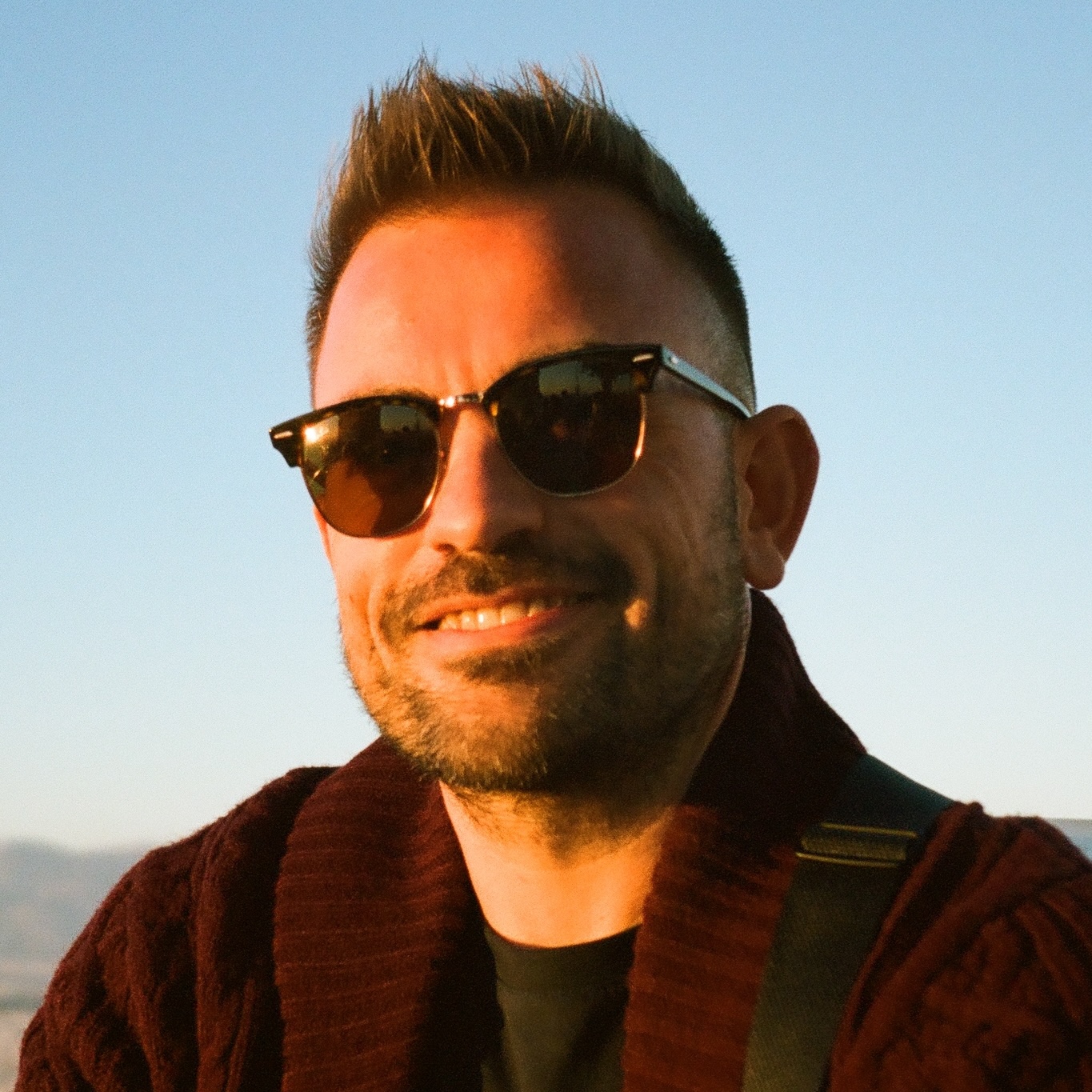
Whether you're building a meditation app or adding background music to a game, looping audio files smoothly in Python can be challenging. This guide explores practical techniques to loop audio without gaps using popular libraries like pydub, librosa, and FFmpeg. We also discuss how to handle various audio formats and batch processing workflows.
Prerequisites
Before starting, ensure that FFmpeg is installed on your system:
- Ubuntu/Debian:
sudo apt-get install ffmpeg
- macOS:
brew install ffmpeg
- Windows: Download from FFmpeg official website
Essential tools setup
Install the required Python libraries with specific versions for compatibility:
pip install pydub==0.25.1 librosa==0.10.2 ffmpeg-python==0.2.0 soundfile==0.12.1
The pydub
library simplifies audio manipulation, librosa
offers advanced signal analysis, and
ffmpeg-python
enables format conversion and batch processing. The soundfile
library provides
robust audio file I/O capabilities.
Basic looping with pydub
The pydub
library makes basic audio looping straightforward. In the example below, a function
repeats an audio segment a specified number of times:
from pydub import AudioSegment
from pydub.exceptions import CouldntDecodeError
def basic_loop(input_file, iterations=3):
try:
audio = AudioSegment.from_file(input_file)
looped = audio * iterations
looped.export("looped_output.mp3", format="mp3")
except CouldntDecodeError:
print(f"Error: Could not decode {input_file}. Check if FFmpeg is installed.")
except Exception as e:
print(f"Error processing {input_file}: {str(e)}")
For smoother transitions between loops, you can add a crossfade effect:
try:
audio = AudioSegment.from_file("chime.wav")
combined = AudioSegment.empty()
for _ in range(5):
combined = combined.append(audio, crossfade=25)
combined.export("smooth_loop.mp3", format="mp3")
except Exception as e:
print(f"Error creating crossfaded loop: {str(e)}")
Seamless loops with librosa
For more precise looping, especially when eliminating pops or gaps, use waveform analysis with
librosa
to align loop boundaries at zero-crossing points:
import librosa
import soundfile as sf
import numpy as np
def find_loop_points(y, sr):
# Detect beats in the audio
tempo, beats = librosa.beat.beat_track(y=y, sr=sr)
beat_samples = librosa.frames_to_samples(beats)
if len(beat_samples) < 2:
return 0, len(y)
# Locate zero crossings around the beat positions
zero_crossings = librosa.zero_crossings(y)
start = beat_samples[0]
end = beat_samples[-1]
# Adjust to the nearest zero crossing
while start > 0 and not zero_crossings[start]:
start -= 1
while end < len(y) and not zero_crossings[end]:
end += 1
return start, end
def create_seamless_loop(input_file, output_file, num_loops=3):
try:
y, sr = librosa.load(input_file, sr=None)
start_sample, end_sample = find_loop_points(y, sr)
loop_segment = y[start_sample:end_sample]
looped = np.tile(loop_segment, num_loops)
sf.write(output_file, looped, sr)
return True
except Exception as e:
print(f"Error creating seamless loop: {str(e)}")
return False
This method uses rhythmic analysis to ensure that the segments join naturally, reducing artifacts in the loop.
Batch processing with FFmpeg
For handling multiple files or format conversion, FFmpeg’s aloop
filter offers a powerful
command-line solution:
import subprocess
import os
def ffmpeg_loop(input_path, output_path, loops):
try:
command = [
"ffmpeg",
"-i", input_path,
"-filter_complex", f"aloop=loop={loops}:size=2e+06",
"-y", output_path
]
subprocess.run(command, check=True, capture_output=True, text=True)
return True
except subprocess.CalledProcessError as e:
print(f"FFmpeg error: {e.stderr}")
return False
except Exception as e:
print(f"Error: {str(e)}")
return False
def batch_process_directory(input_dir, output_dir, loops=3):
os.makedirs(output_dir, exist_ok=True)
for filename in os.listdir(input_dir):
if filename.endswith(('.mp3', '.wav', '.flac', '.ogg')):
input_path = os.path.join(input_dir, filename)
output_path = os.path.join(output_dir, f"looped_{filename}")
ffmpeg_loop(input_path, output_path, loops)
This approach is well suited for automated scripts and processing large batches of audio files.
Performance considerations
When looping long audio files or handling high volumes of data, efficient resource management is essential. Consider these best practices:
-
Memory Management
-
Use chunking to process large files without loading the entire audio into memory. For example:
from pydub.utils import make_chunks def process_large_file(input_file, chunk_size_ms=10000): audio = AudioSegment.from_file(input_file) chunks = make_chunks(audio, chunk_size_ms) return chunks
-
Process audio in 10-30 second segments.
-
Utilize temporary files where necessary.
-
-
Format Optimization
- Use uncompressed formats like WAV or FLAC for editing.
- Convert to compressed formats, such as MP3 or OGG, for distribution.
- Monitor bitrate and quality settings during export.
-
Resource Management
- Always clean up temporary files and use context managers for file operations.
- Consider using parallel processing or a processing pool for batch operations.
Conclusion
Looping audio in Python offers a range of techniques to suit different project needs—from basic repetition with pydub and advanced waveform analysis with librosa to efficient batch processing using FFmpeg. Experiment with these methods to determine the best approach for your application. For scalable, cloud-based audio processing workflows, you might also consider exploring the Transloadit Python SDK.