Mastering file uploads: comprehensive guide for developers
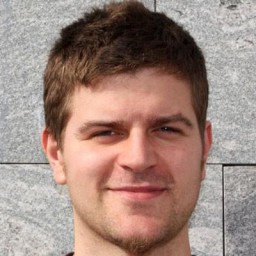
File uploads are a fundamental feature of many web applications, enabling users to transfer files from their local devices to your server. However, implementing a secure and efficient file upload API can be challenging. In this comprehensive guide, we'll delve into what a file upload is, how to set up file upload features, handle them across different frameworks, troubleshoot common issues like "why won't my file upload?", test your file upload API with Postman, and apply essential file upload security best practices.
Introduction: what is a file upload?
Before diving into implementation details, it's important to understand what a file upload is. In web development, a file upload refers to the process that allows users to send files from their local devices to your server through your application. This functionality is essential for applications that require user-generated content, such as profile pictures, documents, or media files. Implementing file uploads effectively ensures a seamless user experience while maintaining application security.
Setting up a basic file upload feature
To begin, let's set up a basic file upload feature using HTML and a simple server in Node.js with Express. This will form the foundation of understanding how file uploads work.
First, create an HTML form that allows users to select a file:
<form action="/upload" method="post" enctype="multipart/form-data">
<input type="file" name="myfile" />
<button type="submit">Upload File</button>
</form>
The enctype="multipart/form-data"
attribute is essential for file uploads, as it tells the browser
to send the form data as multipart/form-data
, which supports file data along with regular form
fields.
Next, set up a Node.js server using Express to handle the file upload:
const express = require('express')
const multer = require('multer')
const path = require('path')
const app = express()
const upload = multer({
dest: 'uploads/',
fileFilter: (req, file, cb) => {
// Only accept certain file types
const filetypes = /jpeg|jpg|png|gif/
const mimetype = filetypes.test(file.mimetype)
const extname = filetypes.test(path.extname(file.originalname).toLowerCase())
if (mimetype && extname) {
return cb(null, true)
} else {
cb(new Error('Error: File type not supported'))
}
},
limits: { fileSize: 5 * 1024 * 1024 }, // Limit file size to 5MB
})
app.post('/upload', upload.single('myfile'), (req, res) => {
res.send('File uploaded successfully.')
})
app.use((err, req, res, next) => {
res.status(400).send(err.message)
})
app.listen(3000, () => {
console.log('Server started on port 3000')
})
In this code:
- We use the
multer
middleware to handlemultipart/form-data
parsing. - We define a
fileFilter
function to validate file types, accepting only images with extensions.jpeg
,.jpg
,.png
, or.gif
. - We set a file size limit to prevent users from uploading excessively large files.
- The uploaded file is saved to the
uploads/
directory. - The
upload.single('myfile')
middleware processes a single file upload with the field namemyfile
. - We added error-handling middleware to catch and respond to errors gracefully.
Handling file uploads in different frameworks
File uploads can be handled in various frameworks. Here's a brief overview:
Python (flask)
from flask import Flask, request
from werkzeug.utils import secure_filename
import os
app = Flask(__name__)
app.config['UPLOAD_FOLDER'] = 'uploads'
@app.route('/upload', methods=['POST'])
def upload():
if 'myfile' not in request.files:
return 'No file part', 400
file = request.files['myfile']
if file.filename == '':
return 'No selected file', 400
if file:
filename = secure_filename(file.filename)
file.save(os.path.join(app.config['UPLOAD_FOLDER'], filename))
return 'File uploaded successfully.'
else:
return 'Error uploading file.', 400
if __name__ == '__main__':
app.run(port=3000)
In this code:
- We use
secure_filename
to sanitize the filename and prevent directory traversal attacks. - We check for the presence of the file and whether a file has been selected.
- The uploaded file is saved securely to the designated upload folder.
PHP
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$target_directory = "uploads/";
$filename = basename($_FILES["myfile"]["name"]);
// Sanitize filename
$filename = preg_replace("/[^A-Za-z0-9_\-\.]/", '_', $filename);
$target_file = $target_directory . $filename;
// Check file type
$file_type = mime_content_type($_FILES["myfile"]["tmp_name"]);
$allowed_types = ['image/jpeg', 'image/png', 'image/gif'];
if (in_array($file_type, $allowed_types)) {
if ($_FILES["myfile"]["size"] <= 5 * 1024 * 1024) { // Limit size to 5MB
if (move_uploaded_file($_FILES["myfile"]["tmp_name"], $target_file)) {
echo "File uploaded successfully.";
} else {
echo "Error uploading file.";
}
} else {
echo "File is too large.";
}
} else {
echo "File type not allowed.";
}
}
?>
In this code:
- We sanitize the uploaded file's name to prevent potentially malicious filenames.
- We check the MIME type of the file to allow only certain types (JPEG, PNG, GIF).
- We set a file size limit to prevent large file uploads.
Ruby on Rails (using active storage)
# In your model (e.g., app/models/user.rb)
class User < ApplicationRecord
has_one_attached :myfile
end
# In your controller (e.g., app/controllers/uploads_controller.rb)
class UploadsController < ApplicationController
def create
@user = User.find(params[:user_id])
if params[:myfile].present?
@user.myfile.attach(params[:myfile])
if @user.myfile.attached?
render plain: "File uploaded successfully."
else
render plain: "Error uploading file.", status: :unprocessable_entity
end
else
render plain: "No file selected.", status: :bad_request
end
end
end
In this code:
- We use Active Storage, Rails' built-in solution for handling file uploads.
- The file is attached to the user model, and we check for its presence before attempting to attach.
- Proper status codes are returned for different error conditions.
Common file upload issues and solutions
Why won't my file upload?
There are several common issues that may prevent files from uploading:
- Incorrect Form Encoding: Ensure your HTML form has
enctype="multipart/form-data"
. - File Size Limits: Servers often have limits on the maximum file size. Check your server
configuration (e.g.,
upload_max_filesize
in PHP, or limit settings in your server code). - Permission Errors: Verify that the server has write permissions to the directory where files are being saved.
- Invalid File Types: Your application might restrict certain file types. Make sure the file you're uploading meets the allowed criteria.
- Missing File Field: Ensure the file input field has the correct
name
attribute and that your server-side code is accessing the correct field. - Path Issues: Ensure that the file paths used in your code are correct and that the directories exist.
- URL or Route Mismatch: Confirm that the form's
action
attribute matches the server's route handling the upload.
Solutions
- Check Server Logs: Server-side error logs can provide insight into why a file isn't uploading.
- Debugging Tools: Use debugging tools or statements to verify that your code is executing as expected.
- Test with Different Files: Try uploading different files to rule out file-specific issues.
Testing file upload APIs with postman
How to test file upload API in postman
Testing your file upload API is crucial to ensure it's working correctly. Here's how to test file uploads using Postman:
- Open Postman and create a new request.
- Set the request method to POST and enter your endpoint URL (e.g.,
http://localhost:3000/upload
). - Go to the Body tab, select form-data.
- Add a key named
myfile
, change the type to File, and select a file from your system. - (Optional) Add any additional fields required by your API.
- Click the Send button to submit the request.
- Review the server's response to verify that the file was uploaded successfully.
If the upload fails, Postman will display the response from your server, which can help in troubleshooting the issue.
Security best practices for file uploads
File upload security
File uploads can introduce security vulnerabilities if not handled properly. Here are some best practices:
- Validate File Types: Only allow specific file types by checking the file's MIME type and extension. Be cautious, as file extensions can be spoofed.
- Limit File Sizes: Set maximum file size limits to prevent denial-of-service attacks.
- Store Files Securely: Save files in a directory not publicly accessible, or store them in cloud storage solutions.
- Rename Files: Generate unique filenames (e.g., using UUIDs) to prevent overwriting existing files and to avoid exposing user data.
- Scan for Malware: Use antivirus software to scan uploaded files for malicious content.
- Avoid Executable Files: Do not allow users to upload executable files or scripts.
- Use Content Security Policy (CSP): Implement CSP headers to prevent the execution of malicious scripts.
- Sanitize Filenames: Remove or replace potentially dangerous characters in filenames.
- Use Secure Protocols: Ensure that file uploads occur over HTTPS to encrypt data in transit.
- Regular Updates: Keep your server and dependencies up to date to mitigate known vulnerabilities.
Conclusion and further resources
Implementing file uploads in your application involves careful consideration of functionality, user experience, and security. By following best practices and thoroughly testing your implementation, you can provide a reliable and secure file upload feature for your users.
For more information, consider exploring the documentation of the frameworks you're using, or check out these resources:
If you're looking for a robust solution to handle file uploads and processing, consider using Transloadit. Transloadit provides powerful APIs and tools to simplify and secure file uploading, enabling you to focus on building your application.