Processing videos in Java: transcode, resize, and watermark
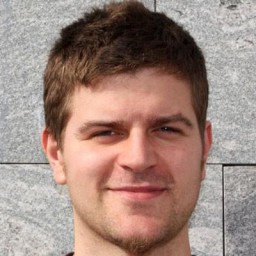
In today's digital landscape, video content is crucial. Whether you're developing a media application or managing a video platform, efficiently processing videos is essential. This post will guide you through transcoding, resizing, and watermarking videos in Java using the open-source library JavaCV, which provides Java wrappers for FFmpeg.
Prerequisites
Before we begin, ensure you have the following installed:
- Java Development Kit (JDK): Version 8 or higher
- Maven: For managing project dependencies
- FFmpeg: Installed and accessible in your system's PATH
Add the following dependency to your pom.xml
to use JavaCV:
<dependencies>
<dependency>
<groupId>org.bytedeco</groupId>
<artifactId>javacv-platform</artifactId>
<version>1.5.8</version>
</dependency>
</dependencies>
Transcoding videos in Java
Here's how to transcode a video from one format to another using JavaCV:
import org.bytedeco.javacv.FFmpegFrameGrabber;
import org.bytedeco.javacv.FFmpegFrameRecorder;
import org.bytedeco.javacv.Frame;
public class VideoTranscoder {
public static void transcode(String inputFile, String outputFile) throws Exception {
try (FFmpegFrameGrabber grabber = new FFmpegFrameGrabber(inputFile)) {
grabber.start();
FFmpegFrameRecorder recorder = new FFmpegFrameRecorder(outputFile,
grabber.getImageWidth(),
grabber.getImageHeight(),
grabber.getAudioChannels());
recorder.setVideoCodec(grabber.getVideoCodec());
recorder.setFormat("mp4");
recorder.setFrameRate(grabber.getFrameRate());
recorder.start();
Frame frame;
while ((frame = grabber.grab()) != null) {
recorder.record(frame);
}
recorder.stop();
recorder.release();
}
}
}
This code reads the input video file and writes it to the output file, transcoding it to the specified format.
Resizing videos using javacv
To resize videos, we'll process each frame and adjust its dimensions:
import org.bytedeco.javacv.*;
import org.bytedeco.opencv.opencv_core.Mat;
import org.bytedeco.opencv.opencv_core.Size;
import static org.bytedeco.opencv.global.opencv_imgproc.resize;
public class VideoResizer {
public static void resizeVideo(String inputFile, String outputFile, int width, int height) throws Exception {
try (FFmpegFrameGrabber grabber = new FFmpegFrameGrabber(inputFile)) {
grabber.start();
FFmpegFrameRecorder recorder = new FFmpegFrameRecorder(outputFile, width, height);
recorder.setVideoCodec(grabber.getVideoCodec());
recorder.setFormat("mp4");
recorder.setFrameRate(grabber.getFrameRate());
recorder.setVideoBitrate(grabber.getVideoBitrate());
recorder.start();
OpenCVFrameConverter.ToMat converter = new OpenCVFrameConverter.ToMat();
Frame frame;
while ((frame = grabber.grabImage()) != null) {
Mat mat = converter.convert(frame);
Mat resizedMat = new Mat();
resize(mat, resizedMat, new Size(width, height));
Frame resizedFrame = converter.convert(resizedMat);
recorder.record(resizedFrame);
mat.release();
resizedMat.release();
}
recorder.stop();
recorder.release();
}
}
}
This code resizes each frame of the input video to the specified dimensions and writes the frames to a new video file.
Adding watermarks to videos
Adding a watermark involves overlaying text or an image onto each frame of the video:
import org.bytedeco.javacv.*;
import org.bytedeco.opencv.opencv_core.*;
import static org.bytedeco.opencv.global.opencv_imgproc.*;
public class VideoWatermarker {
public static void addWatermark(String inputFile, String outputFile, String watermarkText) throws Exception {
try (FFmpegFrameGrabber grabber = new FFmpegFrameGrabber(inputFile)) {
grabber.start();
FFmpegFrameRecorder recorder = new FFmpegFrameRecorder(outputFile,
grabber.getImageWidth(),
grabber.getImageHeight(),
grabber.getAudioChannels());
recorder.setVideoCodec(grabber.getVideoCodec());
recorder.setFormat("mp4");
recorder.setFrameRate(grabber.getFrameRate());
recorder.start();
OpenCVFrameConverter.ToMat converter = new OpenCVFrameConverter.ToMat();
Scalar color = new Scalar(255, 255, 255, 0); // White color
int font = FONT_HERSHEY_SIMPLEX;
Frame frame;
while ((frame = grabber.grab()) != null) {
if (frame.image != null) {
Mat mat = converter.convert(frame);
putText(mat, watermarkText, new Point(50, 50), font, 1.0, color, 2, LINE_AA, false);
Frame watermarkedFrame = converter.convert(mat);
recorder.record(watermarkedFrame);
mat.release();
} else {
recorder.record(frame);
}
}
recorder.stop();
recorder.release();
}
}
}
This code overlays the specified text onto each frame of the video and saves it to a new file.
Integrating video processing into your Java application
Create a service class to encapsulate these functionalities:
public class VideoProcessingService {
public void transcodeVideo(String input, String output) throws Exception {
VideoTranscoder.transcode(input, output);
}
public void resizeVideo(String input, String output, int width, int height) throws Exception {
VideoResizer.resizeVideo(input, output, width, height);
}
public void addWatermark(String input, String output, String watermarkText) throws Exception {
VideoWatermarker.addWatermark(input, output, watermarkText);
}
}
By incorporating this service into your application, you can efficiently handle video processing tasks as needed.
Best practices and considerations
Error handling
Implement robust error handling to account for:
- Invalid file paths or formats
- Unsupported codecs
- Corrupted video files
- Insufficient system resources
Performance optimization
- Multithreading: Utilize Java's concurrency features to process multiple videos simultaneously
- Resource management: Use try-with-resources to ensure proper closure of resources
- Caching: Consider caching processed videos if the same operations are performed frequently
Legal and ethical considerations
When adding watermarks or processing videos, ensure you have the right to modify the content and comply with licensing agreements.
Conclusion
Processing videos in Java using open-source libraries like JavaCV allows you to transcode, resize, and watermark videos efficiently. By integrating these tools into your applications, you can create robust video processing pipelines tailored to your needs.
For more advanced video processing requirements or to handle high volumes of content, consider exploring Transloadit's Video Encoding service, which offers scalable and efficient solutions for video processing tasks.