Scan files for viruses in .Net using open source
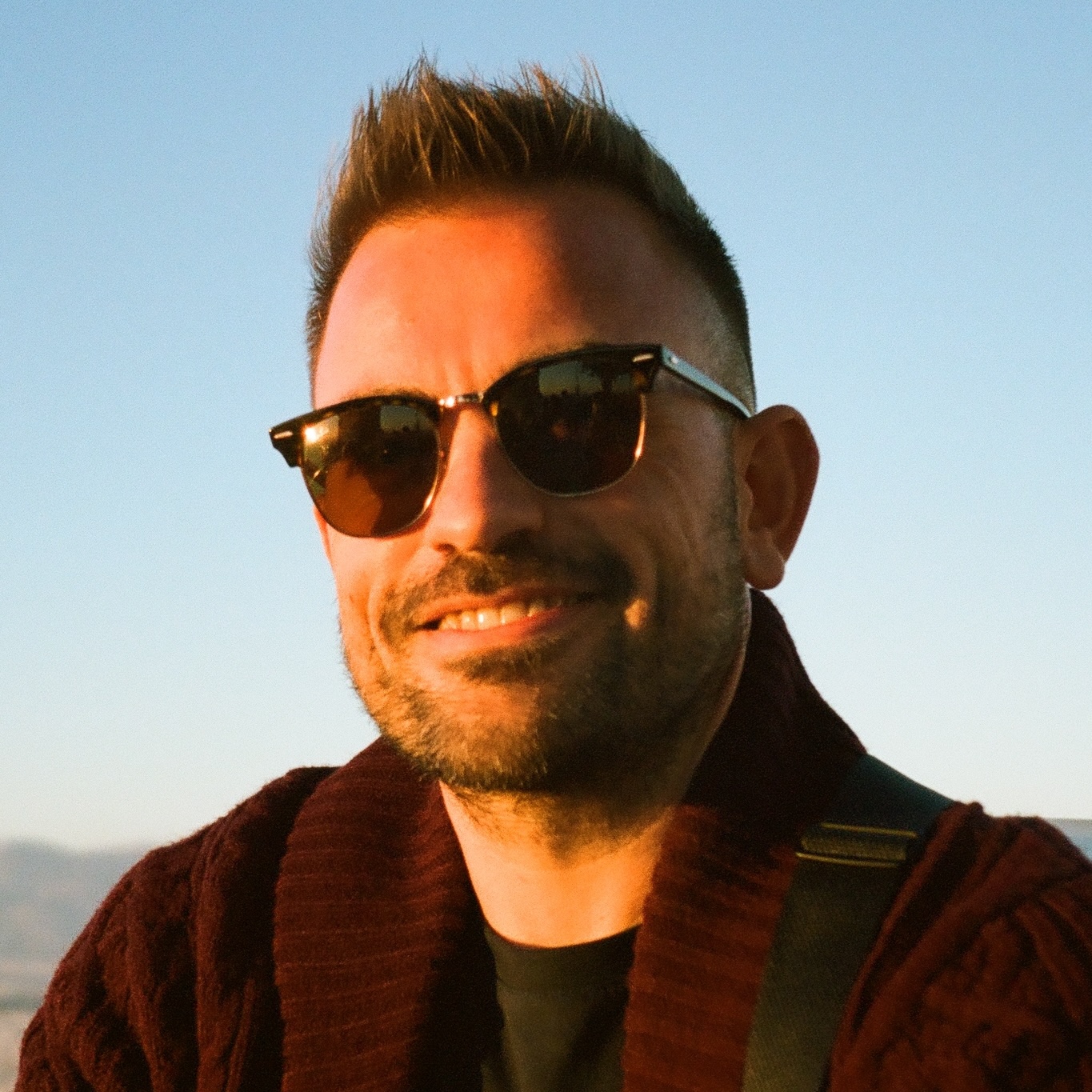
File security is crucial when your applications handle user-uploaded content. In this DevTip, we'll show how to scan files for viruses in your .NET applications using the open-source antivirus tools nClam and AntiVirusScanner.
Introduction
Accepting file uploads introduces the risk of malicious content. Integrating virus scanning into your workflow mitigates this risk, safeguarding your users and infrastructure.
Open-source antivirus tools for .Net
Two effective open-source antivirus tools for .NET are:
- nClam: A .NET client for ClamAV, a widely used open-source antivirus engine.
- AntiVirusScanner: A library that integrates with Windows Defender and other installed antivirus software.
Set up ClamAV and nClam
First, install ClamAV for Windows. After installation, update the virus definitions:
freshclam
Next, install nClam via NuGet:
dotnet add package nClam
Implement file scanning with nClam in C#
Here's how to scan a file using nClam:
using nClam;
public async Task<bool> IsFileSafeAsync(string filePath)
{
var clam = new ClamClient("localhost", 3310);
var scanResult = await clam.SendAndScanFileAsync(filePath);
switch (scanResult.Result)
{
case ClamScanResults.Clean:
return true;
case ClamScanResults.VirusDetected:
Console.WriteLine($"Virus detected: {scanResult.InfectedFiles[0].VirusName}");
return false;
default:
Console.WriteLine("Scan error or unknown result.");
return false;
}
}
Use antivirusscanner for integration with Windows antivirus
AntiVirusScanner uses antivirus software already installed on Windows. Install it via NuGet:
dotnet add package AntiVirusScanner
Here's a simple implementation:
using AntiVirus;
public bool IsFileSafe(string filePath)
{
var scanner = new Scanner();
var result = scanner.ScanAndClean(filePath);
switch (result)
{
case ScanResult.VirusNotFound:
return true;
case ScanResult.VirusFound:
Console.WriteLine("Virus detected!");
return false;
default:
Console.WriteLine("Error during scanning.");
return false;
}
}
Compare nClam and antivirusscanner
Tool | Pros | Cons |
---|---|---|
nClam | Cross-platform, detailed control, ClamAV-based | Requires ClamAV setup and maintenance |
AntiVirusScanner | Easy integration, leverages existing antivirus software | Windows-only, limited scanning control |
Best practices
- Scan files immediately after upload.
- Regularly update antivirus definitions.
- Handle scanning errors gracefully.
- Log scanning results for auditing and troubleshooting.
Conclusion
Integrating virus scanning into your .NET applications significantly enhances security. Both nClam and AntiVirusScanner provide robust solutions, tailored to different needs and environments.
Additional resources:
At Transloadit, we prioritize file security. Our File Filtering service includes a Virus Scan Robot that proactively rejects millions of malicious threats. While we don't currently offer a .NET SDK, you can easily integrate our REST API directly into your applications.