Secure file uploads to Amazon S3 with boto3: best practices
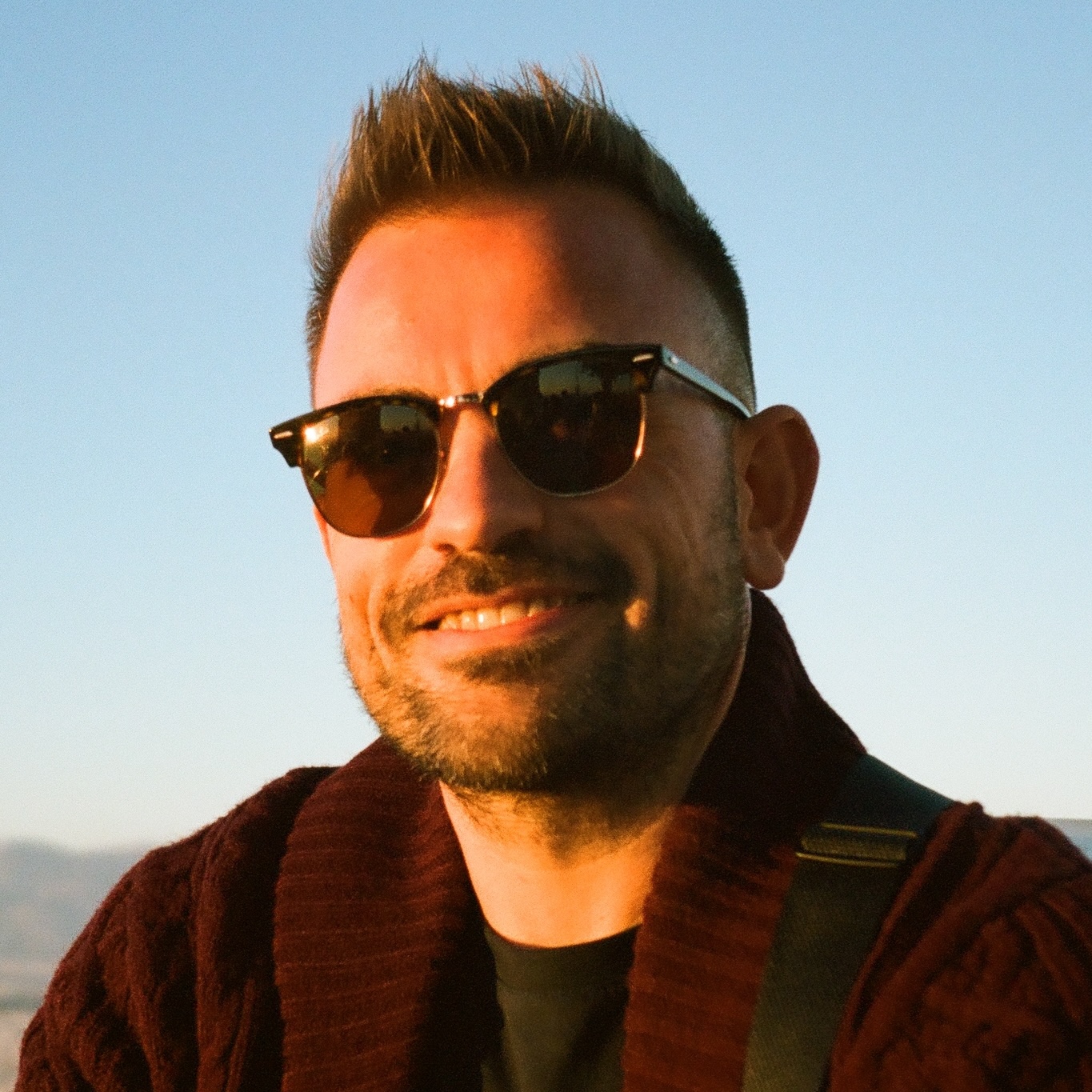
Uploading files to Amazon S3 is a common task for many applications. However, ensuring that these uploads are secure is crucial for protecting sensitive data and maintaining compliance with security standards. In this post, we'll explore how to use Boto3 to securely upload files to Amazon S3, focusing on encryption and access control mechanisms.
Why use boto3 for S3 file uploads?
Boto3 is the Amazon Web Services (AWS) Software Development Kit (SDK) for Python, allowing developers to interact programmatically with AWS services like S3. Using Boto3 provides a seamless way to manage file uploads, handle encryption, and manage access control policies directly from your Python applications.
Setting up your AWS environment
Before diving into code, ensure that you have the necessary environment set up:
-
Install Boto3:
pip install boto3
-
Configure AWS Credentials:
Boto3 uses AWS credentials to authenticate requests. These can be set up using the AWS CLI or by creating a configuration file.
aws configure
Alternatively, you can set environment variables:
export AWS_ACCESS_KEY_ID='YOUR_ACCESS_KEY' export AWS_SECRET_ACCESS_KEY='YOUR_SECRET_KEY' export AWS_DEFAULT_REGION='YOUR_PREFERRED_REGION'
To prevent security risks, never hard-code your AWS credentials in your code.
Uploading a file to S3 with boto3
Here's a basic example of uploading a file to an S3 bucket using Boto3:
import boto3
s3_client = boto3.client('s3')
bucket_name = 'your-bucket-name'
file_path = '/path/to/your/file.txt'
key = 'uploads/file.txt'
response = s3_client.upload_file(file_path, bucket_name, key)
This code snippet uploads file.txt
from your local system to the specified S3 bucket under the key
uploads/file.txt
.
Implementing file encryption on S3
To enhance security, it's important to encrypt your data both in transit and at rest.
Server-side encryption
Amazon S3 offers server-side encryption options. You can specify encryption when uploading files:
response = s3_client.upload_file(
file_path,
bucket_name,
key,
ExtraArgs={
'ServerSideEncryption': 'AES256'
}
)
This enables server-side encryption with Amazon S3-managed keys (SSE-S3). For more advanced encryption, you can use AWS Key Management Service (KMS):
response = s3_client.upload_file(
file_path,
bucket_name,
key,
ExtraArgs={
'ServerSideEncryption': 'aws:kms',
'SSEKMSKeyId': 'your-kms-key-id'
}
)
Client-side encryption
For client-side encryption, you can use the AWS Encryption SDK:
pip install aws-encryption-sdk
import boto3
import aws_encryption_sdk
from aws_encryption_sdk.keyrings.aws_kms import AwsKmsKeyring
session = boto3.session.Session()
kms_key_id = 'your-kms-key-id'
bucket_name = 'your-bucket-name'
file_path = '/path/to/your/file.txt'
key = 'uploads/file.txt'
# Create an AWS kms keyring
keyring = AwsKmsKeyring(generator_key_id=kms_key_id)
# Read the plaintext file
with open(file_path, 'rb') as f:
plaintext = f.read()
# Encrypt the plaintext data
ciphertext, encryptor_header = aws_encryption_sdk.encrypt(
source=plaintext,
keyring=keyring
)
# Upload the encrypted data to S3
s3_client = session.client('s3')
s3_client.put_object(
Bucket=bucket_name,
Key=key,
Body=ciphertext
)
This approach encrypts the data on the client side before uploading it to S3, providing end-to-end encryption.
Managing access control policies for secure uploads
Controlling who can access your data is vital. S3 provides several mechanisms for managing access:
Bucket policies
You can define a bucket policy that specifies who has access to the bucket and what actions they can perform. Here's an example of attaching a policy to your bucket:
import json
bucket_policy = {
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": {"AWS": "arn:aws:iam::AWS-ACCOUNT-ID:user/Username"},
"Action": "s3:*",
"Resource": [
f"arn:aws:s3:::{bucket_name}",
f"arn:aws:s3:::{bucket_name}/*"
]
}
]
}
bucket_policy_json = json.dumps(bucket_policy)
s3_client.put_bucket_policy(
Bucket=bucket_name,
Policy=bucket_policy_json
)
Access control lists (acls)
When uploading a file, you can set the ACL to control access:
response = s3_client.upload_file(
file_path,
bucket_name,
key,
ExtraArgs={
'ACL': 'private'
}
)
By default, objects are private. However, specifying the ACL ensures that the object's access remains restricted.
Automating file upload processes with Python scripts
Automation can streamline your workflows and reduce the potential for human error. Here's a script to upload all files in a directory to S3:
import os
import boto3
s3_client = boto3.client('s3')
def upload_directory(directory_path, bucket_name, s3_prefix=''):
for root, dirs, files in os.walk(directory_path):
for filename in files:
local_path = os.path.join(root, filename)
relative_path = os.path.relpath(local_path, directory_path)
s3_path = os.path.join(s3_prefix, relative_path)
s3_client.upload_file(
local_path,
bucket_name,
s3_path.replace("\\", "/"), # For Windows compatibility
ExtraArgs={
'ServerSideEncryption': 'AES256',
'ACL': 'private'
}
)
print(f'Uploaded {local_path} to s3://{bucket_name}/{s3_path}')
upload_directory('/path/to/your/directory', 'your-bucket-name', 'uploads/')
This script recursively uploads all files in a directory to S3, applying encryption and setting the ACL to private.
Troubleshooting common issues
Access denied errors
Ensure that your AWS IAM user or role has the necessary permissions to perform S3 operations. Attach
a policy that grants the required permissions following the principle of least privilege. Avoid
using overly permissive policies like AmazonS3FullAccess
in production environments.
Credential problems
Double-check that your AWS credentials are correctly configured and that there are no typos.
Kms key access
If using AWS KMS for encryption, verify that your IAM policies allow use of the specified KMS key.
Conclusion
Securing file uploads to Amazon S3 is essential for protecting data and ensuring compliance with security policies. By leveraging Boto3, you can programmatically manage file uploads with encryption and access controls directly in your Python applications.
Key Takeaways:
- Use Boto3 to facilitate secure file uploads efficiently.
- Implement server-side or client-side encryption for data protection.
- Manage access controls using bucket policies and ACLs.
- Automate uploads to improve efficiency and consistency.
By following these best practices, you'll enhance the security of your file uploads and streamline your development workflow.
Looking to simplify your file upload processes even further? Check out Transloadit for powerful file uploading and encoding solutions.