Export files to Supabase with cURL
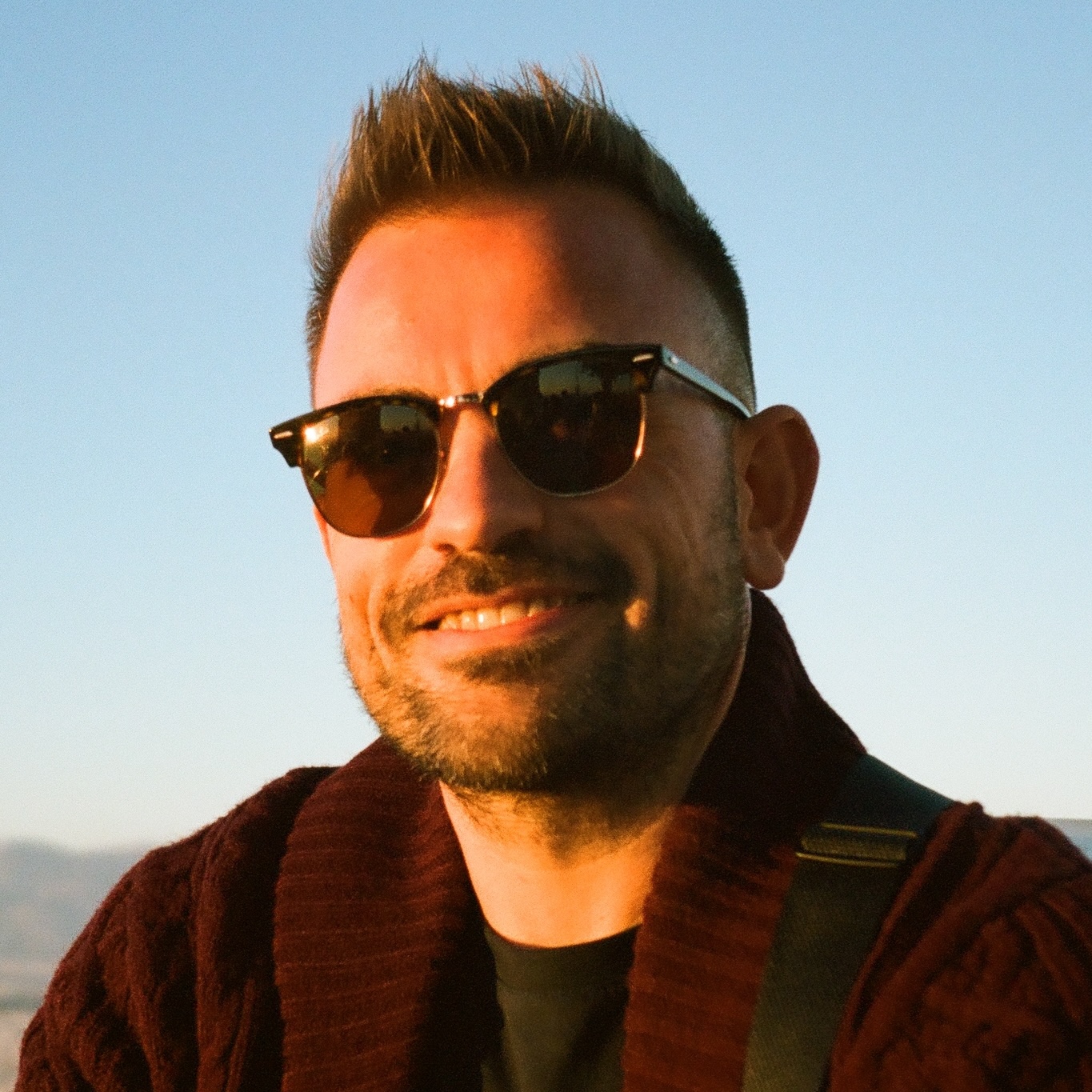
Supabase is a powerful open-source alternative to Firebase, offering developers a robust backend-as-a-service platform. One of its standout features is cloud storage, which allows you to store and manage files effortlessly. In this DevTip, we'll explore how you can streamline your file exports to Supabase storage buckets using cURL commands, enhancing your data management and automation workflows.
Set up your Supabase account and create buckets
First, sign up for a Supabase account if you haven't already. Once logged in, navigate to the "Storage" section and create a new bucket. Ensure you set appropriate permissions for your bucket, typically allowing authenticated users to upload files.
When creating a bucket, you can choose between public and private access policies:
- Public: Files are accessible to anyone with the URL.
- Private: Files require authentication to access.
For sensitive data, always use private buckets with appropriate access controls.
Download and configure cURL
Most systems come with cURL pre-installed. Verify your installation by running:
curl --version
If cURL isn't installed, you can easily install it:
- macOS: Use Homebrew
brew install curl
- Linux (Debian/Ubuntu):
sudo apt-get update && sudo apt-get install curl
- Windows: Use Windows Package Manager (winget) or download from the official website:
winget install curl
Understand cURL basics
cURL is a command-line tool for transferring data using various protocols. Basic syntax:
curl -X METHOD [options] URL
Common methods include GET
, POST
, and PUT
. For Supabase, we'll primarily use POST
for
uploading files.
Export files to Supabase using cURL
To upload files to Supabase, you'll need:
- Your Supabase project ID (found in the project URL)
- Your Supabase anon key (found in Project Settings → API)
- A JWT token for authentication (obtained after user login)
- Your bucket name
Here's the correct cURL command structure for uploading a file:
curl -X POST "https://YOUR_PROJECT_ID.supabase.co/storage/v1/object/YOUR_BUCKET_NAME/file.txt" \
-H "apikey: YOUR_SUPABASE_ANON_KEY" \
-H "Authorization: Bearer YOUR_JWT_TOKEN" \
-H "Content-Type: text/plain" \
--data-binary "@file.txt"
Replace YOUR_PROJECT_ID
, YOUR_BUCKET_NAME
, YOUR_SUPABASE_ANON_KEY
, and YOUR_JWT_TOKEN
with
your actual values. Make sure to specify the correct Content-Type
for your file.
Handle entries: add, overwrite, and update files
To overwrite or update files, use the same command structure. Supabase automatically overwrites files with the same path and name. If you want to prevent overwriting, you can check if a file exists first:
curl -X HEAD "https://YOUR_PROJECT_ID.supabase.co/storage/v1/object/YOUR_BUCKET_NAME/file.txt" \
-H "apikey: YOUR_SUPABASE_ANON_KEY" \
-H "Authorization: Bearer YOUR_JWT_TOKEN"
If the file exists, this will return a 200 status code. If not, it will return a 404.
Understand file size limits
Supabase has specific file size limits you should be aware of:
- Free tier: 50MB maximum file size
- Pro tier: Up to 50GB maximum file size
- Enterprise: Custom limits available
When uploading large files, consider implementing chunked uploads or progress monitoring, which is beyond the scope of this DevTip.
Automate file export in a daily workflow
Automating file uploads can significantly streamline your workflow. Here's a complete bash script example:
#!/bin/bash
# Required variables
PROJECT_ID="your-project-id"
ANON_KEY="your-anon-key"
JWT_TOKEN="your-jwt-token"
BUCKET_NAME="your-bucket"
FILE_PATH="/path/to/daily-report.csv"
FILE_NAME="reports/daily-report-$(date +%Y-%m-%d).csv"
# Upload command with retry logic
curl -X POST \
"https://$PROJECT_ID.supabase.co/storage/v1/object/$BUCKET_NAME/$FILE_NAME" \
-H "apikey: $ANON_KEY" \
-H "Authorization: Bearer $JWT_TOKEN" \
-H "Content-Type: text/csv" \
--retry 3 --retry-delay 5 \
--data-binary "@$FILE_PATH"
# Check for success
if [ $? -eq 0 ]; then
echo "File uploaded successfully to $BUCKET_NAME/$FILE_NAME"
else
echo "Error uploading file"
exit 1
fi
Schedule this script using cron for daily automation:
crontab -e
Add the following line to run the script daily at midnight:
0 0 * * * /path/to/your/script.sh
Troubleshoot common issues
Here are common errors you might encounter and how to resolve them:
Authentication errors (401 unauthorized)
{ "statusCode": "401", "error": "Unauthorized", "message": "Invalid JWT" }
Solution: Ensure your JWT token is valid and not expired. For testing purposes, you can generate a new token through the Supabase Auth API.
Duplicate file errors (409 conflict)
{ "statusCode": "409", "error": "Duplicate", "message": "The resource already exists" }
Solution: Use a unique filename or implement logic to handle existing files.
File size limits (413 payload too large)
{
"statusCode": "413",
"error": "PayloadTooLarge",
"message": "The file size exceeds the maximum limit"
}
Solution: Ensure your file is within the size limits for your Supabase plan.
Permission denied
Solution: Check bucket permissions in Supabase and ensure your JWT token has the necessary permissions.
Adhere to security best practices
When working with Supabase and cURL:
- Store API keys and tokens in environment variables, never hardcode them.
- Use appropriate bucket permissions (private buckets for sensitive data).
- Implement proper error handling and logging.
- Regularly rotate your API keys if they might be compromised.
- Use HTTPS for all requests (which Supabase enforces).
Optimize cURL commands
-
Use environment variables for sensitive data:
export SUPABASE_URL="https://YOUR_PROJECT_ID.supabase.co" export SUPABASE_ANON_KEY="YOUR_ANON_KEY" export SUPABASE_JWT="YOUR_JWT_TOKEN" curl -X POST "$SUPABASE_URL/storage/v1/object/YOUR_BUCKET_NAME/file.txt" \ -H "apikey: $SUPABASE_ANON_KEY" \ -H "Authorization: Bearer $SUPABASE_JWT" \ -H "Content-Type: text/plain" \ --data-binary "@file.txt"
-
Always specify the correct
Content-Type
header for your file type. -
Implement retries for network reliability:
curl --retry 3 --retry-delay 5 -X POST "https://YOUR_PROJECT_ID.supabase.co/storage/v1/object/YOUR_BUCKET_NAME/file.txt" \ -H "apikey: $SUPABASE_ANON_KEY" \ -H "Authorization: Bearer $SUPABASE_JWT" \ -H "Content-Type: text/plain" \ --data-binary "@file.txt"
-
Use the
-f
(fail) flag to make cURL return a non-zero exit code on server errors. -
Add the
-s
(silent) flag to suppress progress meters for cleaner logs.
Conclusion
Using cURL to export files to Supabase provides a powerful, flexible approach to file management and automation. With the correct API endpoints, authentication headers, and best practices, you can build robust file export workflows that integrate seamlessly with your applications.
If you're looking for even more streamlined file exporting solutions, Transloadit offers a comprehensive File Exporting service with support for various storage providers.