Convert documents in Node.js: open-source tools explored
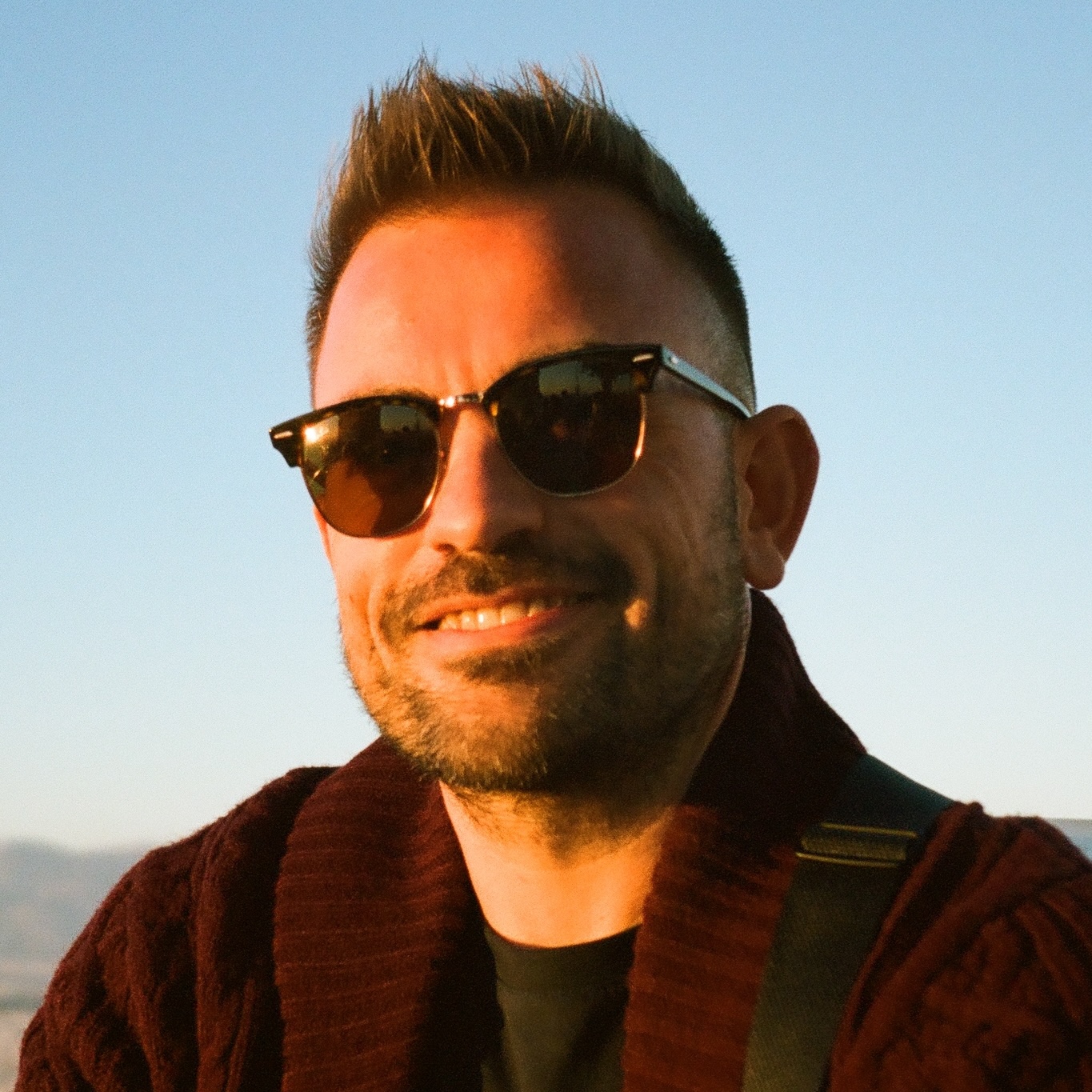
Document conversion is a frequent requirement in Node.js applications, whether you're generating PDFs from HTML, creating invoices, or exporting reports. Fortunately, the Node.js ecosystem provides several robust open-source libraries to simplify these tasks. Let's explore some of the best options available, along with practical examples to help you select the right tool for your project.
Popular open-source libraries for document conversion
Here are some widely used libraries:
- Puppeteer: Headless Chrome automation for HTML-to-PDF conversion.
- PDFKit: Server-side PDF generation with extensive customization.
- jsPDF: Client-side PDF generation directly in the browser.
- wkhtmltopdf: Command-line tool for converting HTML to PDF.
Let's dive deeper into each of these.
Html-to-pdf conversion with puppeteer
Puppeteer is a powerful Node.js library offering a high-level API to control headless Chrome (or Chromium), making it ideal for converting HTML pages into PDFs. It accurately renders web pages, including CSS and JavaScript.
Example usage
const puppeteer = require('puppeteer')
async function htmlToPdf(url, outputPath) {
const browser = await puppeteer.launch()
const page = await browser.newPage()
await page.goto(url, { waitUntil: 'networkidle0' }) // Wait for all network requests to finish
await page.pdf({ path: outputPath, format: 'A4' })
await browser.close()
}
htmlToPdf('https://example.com', 'example.pdf')
Pros and cons
- Pros: Accurate rendering, supports modern CSS and JavaScript, can handle Single Page Applications (SPAs).
- Cons: Higher resource usage due to the headless browser, can be slower than other methods.
Generating PDFs with PDFKit
PDFKit is a versatile library for creating PDFs programmatically on the server-side. It allows for fine-grained control over the PDF's content and layout.
Example usage
const PDFDocument = require('pdfkit')
const fs = require('fs')
const doc = new PDFDocument()
doc.pipe(fs.createWriteStream('output.pdf'))
doc.fontSize(25).text('Hello, PDFKit!', 100, 100)
doc.fontSize(12).text('This is a sample PDF generated using PDFKit.', 100, 150)
doc.end()
Pros and cons
- Pros: Highly customizable, lightweight, good for generating PDFs from scratch.
- Cons: Requires manual layout management, can be complex for intricate designs.
Client-side PDF generation with jsPDF
jsPDF enables PDF generation directly in the browser, often combined with html2canvas for capturing HTML content and converting it to an image, which is then added to the PDF.
Example usage
<script src="https://cdn.jsdelivr.net/npm/jspdf@latest/dist/jspdf.umd.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/html2canvas@latest/dist/html2canvas.min.js"></script>
<div id="content">Hello, jsPDF!</div>
<button onclick="generatePDF()">Download PDF</button>
<script>
async function generatePDF() {
const { jsPDF } = window.jspdf
const content = document.getElementById('content')
const canvas = await html2canvas(content)
const imgData = canvas.toDataURL('image/png')
const pdf = new jsPDF()
pdf.addImage(imgData, 'PNG', 10, 10)
pdf.save('download.pdf')
}
</script>
Pros and cons
- Pros: No server-side processing required, easy integration, suitable for client-side applications.
- Cons: Limited styling and layout control compared to server-side solutions, rendering may differ slightly from the original HTML due to html2canvas limitations.
Advanced conversion with wkhtmltopdf
wkhtmltopdf is a command-line tool that converts HTML to PDF using the WebKit rendering engine. It's known for its speed and ability to handle complex layouts.
Example usage
const { exec } = require('child_process')
exec('wkhtmltopdf https://example.com output.pdf', (error) => {
if (error) {
console.error(`Error: ${error.message}`)
return
}
console.log('PDF generated successfully')
})
Pros and cons
- Pros: Fast, reliable, supports complex layouts and CSS, good for batch processing.
- Cons: Requires installation of an external binary, may have compatibility issues with very modern web features.
Choosing the right library
Consider these factors when selecting a library:
- Complexity: Puppeteer and wkhtmltopdf handle complex layouts and dynamic content well. If you need pixel-perfect rendering of a webpage, these are good choices.
- Performance: PDFKit is lightweight and fast for generating simple PDFs from scratch. If speed is critical and your PDF structure is relatively simple, PDFKit is a strong contender.
- Environment: jsPDF is ideal for client-side applications where you want to generate PDFs directly in the user's browser.
- Dependencies: wkhtmltopdf requires a separate binary installation, while the others are pure Node.js libraries (although Puppeteer manages its own Chromium/Chrome binary).
Evaluate your project's specific needs to choose the best fit. For example, if you need to generate invoices with a consistent layout, PDFKit might be a good choice. If you need to convert a complex, JavaScript-heavy web page to PDF, Puppeteer would be more suitable.
Transloadit's document conversion
If you're looking for a managed solution, Transloadit's
Document Processing service offers robust
document conversion capabilities through its /document/convert
Robot. You can easily integrate
this into your Node.js applications using our node-sdk.