Implementing OCR in Android and iOS apps with open-source SDKs
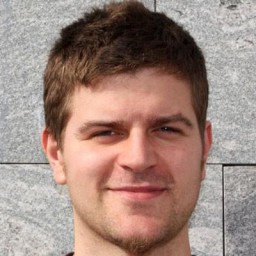
Adding OCR capabilities to your Android and iOS apps can significantly enhance their functionality. This guide will walk you through integrating Tesseract OCR, a popular open-source SDK, into your mobile applications for text recognition from images.
Introduction to OCR in mobile apps
Optical Character Recognition (OCR) technology enables computers to extract text from digital images. Implementing OCR in mobile apps opens up possibilities for document scanning, text recognition, and improved user experiences by converting images to editable text.
Overview of open-source OCR SDKs
Several open-source OCR SDKs are available for mobile app development. Tesseract OCR, maintained by Google, stands out as one of the most popular options. It supports a wide range of languages and offers accurate text recognition capabilities.
Setting up tesseract OCR in Android
To integrate Tesseract OCR into your Android app:
-
Add Tesseract OCR to your project:
Include Tesseract OCR using the Tess-Two library, an Android port of Tesseract. Add this dependency to your
build.gradle
file:dependencies { implementation 'com.googlecode.tesseract.android:tess-two:9.1.0' }
-
Include trained data files:
Download the appropriate
*.traineddata
files for your target languages from the Tesseract OCR Data GitHub repository. Place these files in theassets/tessdata
directory of your project.
Implementing OCR in an Android app
Here's how to implement OCR functionality in your Android app:
-
Initialize Tesseract OCR:
TessBaseAPI tessBaseAPI = new TessBaseAPI(); String dataPath = getFilesDir() + "/tesseract/"; tessBaseAPI.init(dataPath, "eng"); // 'eng' for English language
-
Prepare the image for OCR:
Bitmap image = // Obtain your bitmap image
-
Perform OCR on the image:
tessBaseAPI.setImage(image); String recognizedText = tessBaseAPI.getUTF8Text();
-
Release resources:
tessBaseAPI.end();
Complete example:
public String extractTextFromImage(Bitmap bitmap) {
TessBaseAPI tessBaseAPI = new TessBaseAPI();
String dataPath = getFilesDir() + "/tesseract/";
tessBaseAPI.init(dataPath, "eng");
tessBaseAPI.setImage(bitmap);
String recognizedText = tessBaseAPI.getUTF8Text();
tessBaseAPI.end();
return recognizedText;
}
Setting up tesseract OCR in iOS
To integrate Tesseract OCR into your iOS app:
-
Use a Tesseract OCR wrapper:
The TesseractOCRiOS library is commonly used to integrate Tesseract in iOS. Add it to your project via CocoaPods. In your
Podfile
, include:pod 'TesseractOCRiOS', '4.0.0'
Then run:
pod install
-
Include trained data files:
Download the
*.traineddata
files for your target languages from the Tesseract OCR Data GitHub repository. Add these files to your app bundle and ensure they're included in the build resources.
Implementing OCR in an iOS app
Here's how to implement OCR functionality in your iOS app:
-
Import the Tesseract OCR module:
#import <TesseractOCR/TesseractOCR.h>
-
Initialize and configure Tesseract:
- (NSString *)extractTextFromImage:(UIImage *)image { G8Tesseract *tesseract = [[G8Tesseract alloc] initWithLanguage:@"eng"]; tesseract.engineMode = G8OCREngineModeTesseractOnly; tesseract.pageSegmentationMode = G8PageSegmentationModeAuto; tesseract.image = [image g8_blackAndWhite]; [tesseract recognize]; return tesseract.recognizedText; }
This method takes a
UIImage
, processes it, and returns the recognized text.
Tips for optimizing OCR performance
To improve OCR accuracy and performance in your mobile apps:
-
Preprocess images: Enhance image quality by converting to grayscale, increasing contrast, or applying noise reduction filters.
-
Use appropriate page segmentation modes: Tesseract offers various page segmentation modes. Choose the one that best fits your content type.
-
Restrict character sets: If you expect only specific types of input (e.g., numeric), configure Tesseract to recognize only those characters.
-
Manage resource usage: Perform OCR operations on background threads to keep the UI responsive.
-
Cache results: For similar images, consider caching results to avoid redundant OCR operations.
Conclusion
Integrating OCR capabilities into your Android and iOS apps using open-source SDKs like Tesseract OCR can significantly enhance their functionality. This guide has walked you through the setup and implementation process for both platforms, enabling you to add powerful text recognition features to your mobile applications.
For more advanced file processing capabilities, including image manipulation and document processing, consider exploring Transloadit's services, which can complement your OCR functionalities and streamline your app's workflow.