Import files from Backblaze in go: efficient techniques
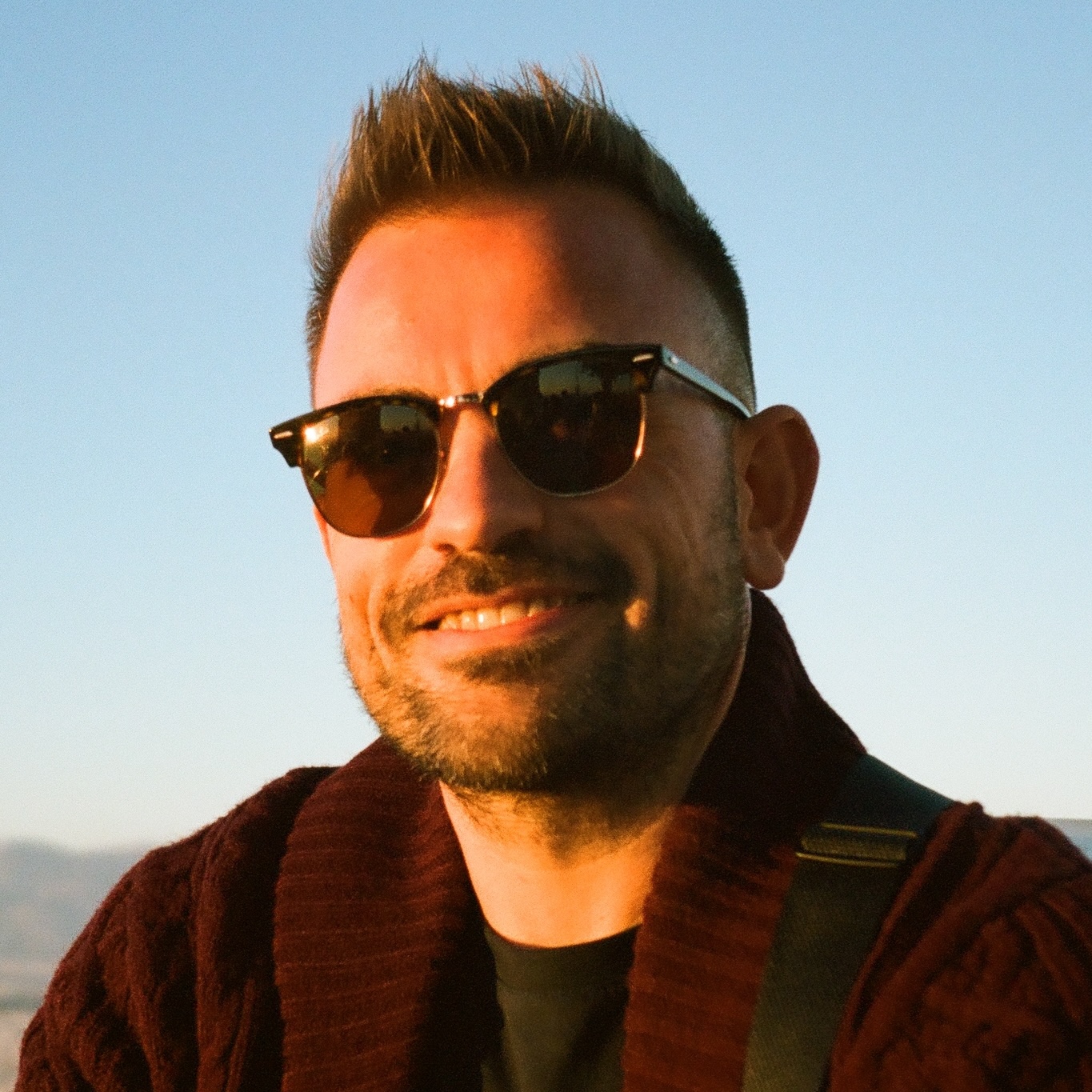
Backblaze B2 is a cost-effective cloud storage solution favored by developers for its simplicity and scalability. In this guide, we'll explore efficient techniques to import files from Backblaze B2 using Go. We'll cover everything from setting up your Go environment to handling errors and optimizing performance when importing files and directories.
Understanding the Backblaze B2 API
Backblaze B2 provides a RESTful API that allows developers to interact with their cloud storage programmatically. The API supports various operations, including file upload, download, and listing. For Go developers, Backblaze offers an official SDK, making it easier to integrate B2 storage into Go applications.
Setting up your Go environment for Backblaze file importing
To begin, ensure you have Go installed on your machine. Then, create a new Go module and install the Backblaze B2 SDK:
go mod init backblaze-import
go get github.com/Backblaze/b2-sdk-go
Using the Go SDK to connect to Backblaze B2
Next, we'll establish a connection to Backblaze B2 using your account credentials. Replace
'YOUR_ACCOUNT_ID'
, 'YOUR_APPLICATION_KEY'
, and 'YOUR_BUCKET_NAME'
with your actual Backblaze
B2 account ID, application key, and bucket name.
package main
import (
"context"
"log"
"github.com/Backblaze/b2-sdk-go/b2"
)
func main() {
ctx := context.Background()
accountID := "YOUR_ACCOUNT_ID"
applicationKey := "YOUR_APPLICATION_KEY"
// Create a new B2 client
b2Client, err := b2.NewClient(ctx, accountID, applicationKey, nil)
if err != nil {
log.Fatalf("Failed to create B2 client: %v", err)
}
// Get the bucket
bucketName := "YOUR_BUCKET_NAME"
bucket, err := b2Client.Bucket(ctx, bucketName)
if err != nil {
log.Fatalf("Failed to get bucket: %v", err)
}
// Your code to interact with the bucket goes here
}
This code initializes a B2 client and retrieves a reference to your bucket, which we'll use in subsequent operations.
Implementing basic file import functionality in go
Now, let's implement a function to download a single file from Backblaze B2:
import (
"context"
"fmt"
"io"
"os"
)
func downloadFile(ctx context.Context, bucket *b2.Bucket, fileName, localPath string) error {
// Open the local file for writing
file, err := os.Create(localPath)
if err != nil {
return fmt.Errorf("failed to create local file: %v", err)
}
defer file.Close()
// Download the file from B2
reader, err := bucket.DownloadFileByName(ctx, fileName)
if err != nil {
return fmt.Errorf("failed to download file: %v", err)
}
defer reader.Close()
// Write the content to the local file
_, err = io.Copy(file, reader)
if err != nil {
return fmt.Errorf("failed to write file: %v", err)
}
return nil
}
This function creates a local file and streams the content from Backblaze B2 directly into it, efficiently handling memory usage.
Handling directories and batch imports: best practices
To import multiple files or entire directories, you can implement batch processing. Here's how to list files with a specific prefix and download them:
import (
"path/filepath"
)
func batchDownload(ctx context.Context, bucket *b2.Bucket, prefix string) error {
// Initialize a continuation token
var next string
for {
// List files with the given prefix
files, err := bucket.ListFileNames(ctx, 1000, prefix, next)
if err != nil {
return fmt.Errorf("failed to list files: %v", err)
}
for _, fileInfo := range files.Files {
localPath := filepath.Join("downloads", fileInfo.Name)
// Create directory structure if needed
if dir := filepath.Dir(localPath); dir != "" {
if err := os.MkdirAll(dir, 0755); err != nil {
return fmt.Errorf("failed to create directory: %v", err)
}
}
if err := downloadFile(ctx, bucket, fileInfo.Name, localPath); err != nil {
return fmt.Errorf("failed to download %s: %v", fileInfo.Name, err)
}
}
// Check if there are more files to list
if files.Next == "" {
break
}
next = files.Next
}
return nil
}
This function handles pagination with the Next
token, enabling you to download all files matching
the specified prefix. It also creates the necessary local directory structure to mirror the files in
your Backblaze B2 bucket.
Error handling and debugging: ensuring smooth file transfers
Network issues and transient errors can occur when transferring files. Implementing retries with exponential backoff can improve reliability:
import (
"time"
)
func downloadWithRetry(ctx context.Context, bucket *b2.Bucket, fileName, localPath string, maxRetries int) error {
var lastErr error
for i := 0; i < maxRetries; i++ {
err := downloadFile(ctx, bucket, fileName, localPath)
if err == nil {
return nil
}
lastErr = err
wait := time.Duration(1<<uint(i)) * time.Second
log.Printf("Retrying in %v...", wait)
time.Sleep(wait)
}
return fmt.Errorf("failed after %d retries: %v", maxRetries, lastErr)
}
This function attempts to download a file, retrying with an exponential backoff strategy if it encounters errors.
Progress tracking for large file transfers
For large file imports, it's helpful to track and display progress:
import (
"github.com/cheggaaa/pb/v3"
)
func downloadFileWithProgress(ctx context.Context, bucket *b2.Bucket, fileName, localPath string) error {
// Get file info to know the total size
fileInfo, err := bucket.FileByName(ctx, fileName)
if err != nil {
return fmt.Errorf("failed to get file info: %v", err)
}
// Open the local file for writing
file, err := os.Create(localPath)
if err != nil {
return fmt.Errorf("failed to create local file: %v", err)
}
defer file.Close()
// Download the file from B2
reader, err := bucket.DownloadFileByName(ctx, fileName)
if err != nil {
return fmt.Errorf("failed to download file: %v", err)
}
defer reader.Close()
// Set up progress bar
bar := pb.Full.Start64(fileInfo.ContentLength)
barReader := bar.NewProxyReader(reader)
defer bar.Finish()
// Write the content to the local file with progress
_, err = io.Copy(file, barReader)
if err != nil {
return fmt.Errorf("failed to write file: %v", err)
}
return nil
}
This example uses the github.com/cheggaaa/pb/v3
library to display a progress bar in the terminal
during the file download.
Common pitfalls and troubleshooting tips
Memory management
For large files, it's essential to stream downloads rather than loading files entirely into memory. The previous examples stream the content directly to disk, ensuring efficient memory usage.
Rate limiting
Backblaze B2 imposes rate limits on API calls. To avoid throttling, implement rate limiting in your application:
import (
"golang.org/x/time/rate"
)
var limiter = rate.NewLimiter(10, 1) // 10 requests per second
func rateLimitedDownload(ctx context.Context, bucket *b2.Bucket, fileName, localPath string) error {
err := limiter.Wait(ctx)
if err != nil {
return err
}
return downloadFile(ctx, bucket, fileName, localPath)
}
This code snippet uses the rate
package from the Go standard library to limit the number of
requests per second.
Handling network interruptions
Ensure your application can handle network interruptions gracefully by implementing timeouts and cancellation contexts.
func downloadFileWithTimeout(bucket *b2.Bucket, fileName, localPath string, timeout time.Duration) error {
ctx, cancel := context.WithTimeout(context.Background(), timeout)
defer cancel()
return downloadFile(ctx, bucket, fileName, localPath)
}
Conclusion and further resources
Importing files from Backblaze B2 using Go is straightforward when leveraging the official Go SDK. By following best practices for error handling, rate limiting, and resource management, you can build robust applications that efficiently import files and directories.
If you're looking for a managed solution for importing files from Backblaze B2, check out Transloadit's file importing service, which handles these complexities for you.